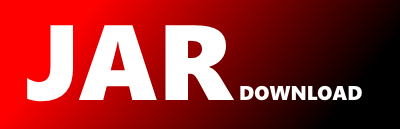
com.evasion.AbstractModule Maven / Gradle / Ivy
/*
* To change this template, choose Tools | Templates
* and open the template in the editor.
*/
package com.evasion;
import com.evasion.ejb.local.ParametreManagerLocal;
import com.evasion.exception.EvasionException;
import java.lang.reflect.Field;
import java.security.Principal;
import java.util.*;
import javax.annotation.PostConstruct;
import javax.annotation.Resource;
import javax.ejb.EJB;
import javax.ejb.SessionContext;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.springframework.beans.BeanWrapperImpl;
import org.springframework.beans.BeansException;
/**
*
* @author sglon
*/
public abstract class AbstractModule implements IModule {
private static final Logger LOGGER = LoggerFactory.getLogger(AbstractModule.class);
@EJB
private ParametreManagerLocal paramManager;
private Map propertiesMap = new HashMap();
@Override
public void refreshModuleProperties() {
for (ModuleProperty object : propertiesMap.values()) {
object.setValue(paramManager.getProperty(object.getKey()));
}
}
@PostConstruct
@Override
public void registerModule() {
String value;
for (ModuleProperty object : propertiesMap.values()) {
if (object.getValue() == null || object.getValue().isEmpty()) {
value = object.getDefaultValue();
} else {
value = object.getValue();
}
paramManager.saveParametre(object.getKey(), value, Boolean.TRUE);
}
}
/**
* Recherche par
*
* @return
*/
@Override
public Collection getModuleProperties() {
return this.propertiesMap.values();
}
@Override
public void setModuleProperty(String key, Object value) {
throw new UnsupportedOperationException("Not supported yet.");
}
@Override
public String getModuleProperty(String key) {
return paramManager.getProperty(key);
}
@Override
public String getPrinciaplUserName() throws EvasionException {
return (String) getPrincipalProperty("name");
}
@Override
public Object getPrincipalProperty(String property) throws EvasionException {
Object result = null;
Principal user = getPrincipal();
if (user == null) {
return null;
}
try {
LOGGER.debug("Principal type: " + user);
BeanWrapperImpl wrapper = new BeanWrapperImpl(user);
result = wrapper.getPropertyValue(property);
} catch (BeansException ex) {
throw new EvasionException("Can't find principal property.", ex);
}
//escapeEntities(String.valueOf(result));
return result;
}
@Override
public Date getUserLastLogin() throws EvasionException {
Calendar date = new GregorianCalendar();
if (getPrincipalProperty("lastLogin") != null) {
date.setTime((Date) getPrincipalProperty("lastLogin"));
}
return date.getTime();
}
@Override
public abstract Principal getPrincipal() throws EvasionException;
protected static Principal glassfishWorkAround(Principal webPrincipal) {
Principal evPrincipal = null;
try {
if (webPrincipal != null) {
LOGGER.debug("UserPrincipal type: {}", webPrincipal.getClass().getName());
Class clazzGlassfish = Class.forName("com.sun.enterprise.security.web.integration.WebPrincipal");
Class clazzEvasion = Class.forName("com.evasion.sam.jaas.EvasionPrincipal");
if (clazzEvasion.isInstance(webPrincipal)) {
evPrincipal = webPrincipal;
} else if (clazzGlassfish.isInstance(webPrincipal)) {
Field customPrincipal = clazzGlassfish.getDeclaredField("customPrincipal");
customPrincipal.setAccessible(true);
evPrincipal = (Principal) customPrincipal.get(webPrincipal);
}
}
} catch (IllegalArgumentException ex) {
LOGGER.error("glassfishWorkAround", ex);
} catch (IllegalAccessException ex) {
LOGGER.error("glassfishWorkAround", ex);
} catch (NoSuchFieldException ex) {
LOGGER.error("glassfishWorkAround", ex);
} catch (SecurityException ex) {
LOGGER.error("glassfishWorkAround", ex);
} catch (ClassNotFoundException ex) {
LOGGER.error("glassfishWorkAround", ex);
}
return evPrincipal;
}
protected ParametreManagerLocal getParamManager() {
return paramManager;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy