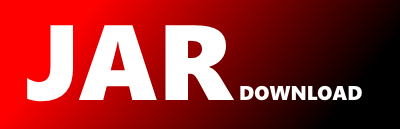
com.evasion.entity.geolocation.City Maven / Gradle / Ivy
/*
* To change this template, choose Tools | Templates
* and open the template in the editor.
*/
package com.evasion.entity.geolocation;
import javax.persistence.*;
import org.apache.commons.lang.builder.EqualsBuilder;
import org.apache.commons.lang.builder.HashCodeBuilder;
import org.apache.commons.lang.builder.ToStringBuilder;
/**
*
* @author sglon
*/
@Entity(name = City.ENTITY_NAME)
@Table(name = City.ENTITY_NAME)
public class City extends Location {
/**
* *
* serialVersionUID.
*/
private static final long serialVersionUID = 1L;
public static final String ENTITY_NAME = "GEO_CITY";
@ManyToOne(optional = false)
private Country country;
@ManyToOne(optional = true)
private AdminDivision admDivision;
//@ManyToOne(fetch = FetchType.LAZY, optional = true)
//@JoinColumn(unique=true,name="SUB_DIVISION")
//private List subdivisionInternal;
@Embedded
private Geoname geoname;
/**
* Constructeur par défaut pour la persistence.
*/
protected City() {
}
/**
* Constructeur général.
*
* @param country {@link Country}
* @param name Nom complet.
* @param location Position et altitude geographique.
*/
public City(Country country, Location location) {
super(location);
this.country = country;
}
public AdminDivision getAdmDivision() {
return admDivision;
}
public void setAdmDivision(AdminDivision admDivision) {
this.admDivision = admDivision;
}
public com.evasion.entity.geolocation.Country getCountry() {
return country;
}
public void setCountry(com.evasion.entity.geolocation.Country country) {
this.country = country;
}
public Geoname getGeoname() {
return geoname;
}
public void setGeoname(Geoname geoname) {
this.geoname = geoname;
}
/**
* {@inheritDoc }
*/
@Override
public boolean equals(final Object obj) {
if (obj == null) {
return false;
}
if (this == obj) {
return true;
}
if (!(obj instanceof City)) {
return false;
}
City rhs = (City) obj;
return new EqualsBuilder().append(this.getName(), rhs.getName()).
isEquals();
}
/**
* @{@inheritDoc }
*/
@Override
public int hashCode() {
return new HashCodeBuilder(17, 37).append(this.getName()).toHashCode();
}
/**
* @{@inheritDoc }
*/
@Override
public String toString() {
return new ToStringBuilder(this).append("name=", this.getName()).
append("country", this.getCountry()).
append("admDiv", this.getAdmDivision()).
toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy