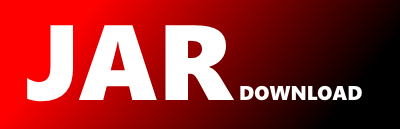
com.eventsourcing.EventStream Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of eventsourcing-core Show documentation
Show all versions of eventsourcing-core Show documentation
Event capture and querying framework for Java
/**
* Copyright (c) 2016, All Contributors (see CONTRIBUTORS file)
*
* This Source Code Form is subject to the terms of the Mozilla Public
* License, v. 2.0. If a copy of the MPL was not distributed with this
* file, You can obtain one at http://mozilla.org/MPL/2.0/.
*/
package com.eventsourcing;
import lombok.Getter;
import java.util.stream.Stream;
/**
* EventStream is a wrapper around Event<Stream>
that holds
* a typed state.
*
* It is used for event generation and passing of state from {@link Command#events(Repository, LockProvider)}
* to {@link Command#result(Object, Repository, LockProvider)}
*
* @param state type
*/
public class EventStream {
@Getter
private Stream extends Event> stream;
@Getter
private S state;
EventStream(S state, Stream extends Event> stream) {
this.state = state;
this.stream = stream;
}
public static class Builder {
private final S state;
private final Stream.Builder builder = Stream.builder();
public Builder(S state) {
this.state = state;
}
public void accept(Event event) {
builder.accept(event);
}
public Builder add(Event event) {
accept(event);
return this;
}
public EventStream build() {
return new EventStream<>(state, builder.build());
}
}
/**
* EventStream builder
*
* @param state state
* @param state type
* @return
*/
public static Builder builder(S state) {
return new Builder<>(state);
}
/**
* EventStream builder with state set to null
*
* @param state type
* @return
*/
public static Builder builder() {
return new Builder<>(null);
}
/**
* @param state type
* @return empty event stream with state set to null
*/
public static EventStream empty() {
return new EventStream<>(null, Stream.empty());
}
/**
* @param state state
* @param state type
* @return empty event stream with a state
*/
public static EventStream empty(S state) {
return new EventStream<>(state, Stream.empty());
}
/**
* @param state state
* @param stream stream of events
* @param state type
* @return event stream with a state and a stream
*/
public static EventStream ofWithState(S state, Stream extends Event> stream) {
return new EventStream<>(state, stream);
}
/**
* @param stream stream of events
* @param state type
* @return event stream with a state set to null
and a stream
*/
public static EventStream of(Stream extends Event> stream) {
return new EventStream<>(null, stream);
}
/**
* @param state state
* @param event event
* @param state type
* @return event stream with a state and a stream of one event
*/
public static EventStream ofWithState(S state, Event event) {
return new EventStream<>(state, Stream.of(event));
}
/**
* @param event event
* @param state type
* @return event stream with a state set to null
and a stream of one event
*/
public static EventStream of(Event event) {
return new EventStream<>(null, Stream.of(event));
}
/**
* @param state state
* @param events events
* @param state type
* @return event stream with a state and a stream of multiple events
*/
public static EventStream ofWithState(S state, Event ...events) {
return new EventStream<>(state, Stream.of(events));
}
/**
*
* @param events events
* @param state type
* @return event stream with a state set to null
and a stream of multiple events
*/
public static EventStream of(Event ...events) {
return new EventStream<>(null, Stream.of(events));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy