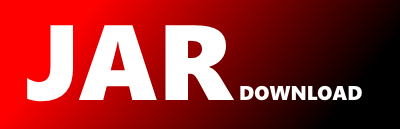
com.eventsourcing.Journal Maven / Gradle / Ivy
Show all versions of eventsourcing-core Show documentation
/**
* Copyright (c) 2016, All Contributors (see CONTRIBUTORS file)
*
* This Source Code Form is subject to the terms of the Mozilla Public
* License, v. 2.0. If a copy of the MPL was not distributed with this
* file, You can obtain one at http://mozilla.org/MPL/2.0/.
*/
package com.eventsourcing;
import com.eventsourcing.hlc.HybridTimestamp;
import com.google.common.util.concurrent.Service;
import com.googlecode.cqengine.index.support.CloseableIterator;
import lombok.SneakyThrows;
import java.util.Optional;
import java.util.Set;
import java.util.UUID;
import java.util.function.Consumer;
import java.util.stream.Stream;
/**
* Journal is the storage of all events and commands registered
* through Eventsourcing.
*/
public interface Journal extends Service {
Listener DEFAULT_LISTENER = new Listener() {};
default void onCommandsAdded(Set> commands) {}
default void onEventsAdded(Set> events) {}
/**
* Set repository. Should be done before invoking {@link #startAsync()}
*
* @param repository
*/
void setRepository(Repository repository);
/**
* Get repository
* @return
*/
Repository getRepository();
/**
* Retrieves a command or event by UUID
*
* @param uuid
* @param
* @return Empty {@link Optional} if neither a command nor an event are found by uuid
*/
Optional get(UUID uuid);
/**
* Iterate over commands of a specific type (through {@code EntityHandler})
*
* @param klass
* @param
* @return iterator
*/
> CloseableIterator> commandIterator(Class klass);
/**
* Iterate over events of a specific type (through {@code EntityHandler})
*
* @param klass
* @param
* @return iterator
*/
CloseableIterator> eventIterator(Class klass);
/**
* Removes everything from the journal.
*
* Use with caution: the data will be lost irrevocably
*/
void clear();
/**
* Returns the count of entities of specified type
*
* @param klass
* @param
* @return
*/
long size(Class klass);
/**
* Returns true if there are no entities of specified type stored
*
* @param klass
* @param
* @return
*/
boolean isEmpty(Class klass);
/**
* Record command within a transaction
* @param tx
* @param command
* @param
* @param
* @return
*/
Command journal(Transaction tx, Command command);
/**
* Record event within a transaction
* @param tx
* @param event
* @return
*/
Event journal(Transaction tx, Event event);
/**
* Starts a transaction
* @return
*/
Transaction beginTransaction();
/**
* An interface abstracting journal's transaction
*/
interface Transaction {
void commit();
void rollback();
}
}