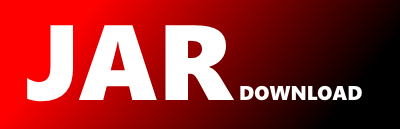
com.eventsourcing.index.AbstractAttributeIndex Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of eventsourcing-core Show documentation
Show all versions of eventsourcing-core Show documentation
Event capture and querying framework for Java
/**
* Copyright (c) 2016, All Contributors (see CONTRIBUTORS file)
*
* This Source Code Form is subject to the terms of the Mozilla Public
* License, v. 2.0. If a copy of the MPL was not distributed with this
* file, You can obtain one at http://mozilla.org/MPL/2.0/.
*/
package com.eventsourcing.index;
import com.eventsourcing.Entity;
import com.eventsourcing.EntityHandle;
import com.eventsourcing.layout.*;
import com.eventsourcing.layout.binary.BinarySerialization;
import com.eventsourcing.layout.types.ObjectTypeHandler;
import com.fasterxml.classmate.ResolvedType;
import com.fasterxml.classmate.TypeResolver;
import com.googlecode.cqengine.query.Query;
import lombok.SneakyThrows;
import java.lang.reflect.AnnotatedParameterizedType;
import java.lang.reflect.AnnotatedType;
import java.util.Set;
public abstract class AbstractAttributeIndex
extends com.googlecode.cqengine.index.support.AbstractAttributeIndex> {
protected Serializer attributeSerializer;
protected Deserializer attributeDeserializer;
protected ObjectSerializer objectSerializer;
protected ObjectDeserializer objectDeserializer;
private final static Serialization serialization = BinarySerialization.getInstance();
protected final TypeHandler attrTypeHandler;
/**
* Protected constructor, called by subclasses.
*
* @param attribute The attribute on which the index will be built
* @param supportedQueries The set of {@link Query} types which the subclass implementation supports
*/
@SneakyThrows
protected AbstractAttributeIndex(Attribute attribute, Set>
supportedQueries) {
super(attribute, supportedQueries);
ResolvedType attributeType = new TypeResolver().resolve(attribute.getAttributeType());
AnnotatedParameterizedType cls = (AnnotatedParameterizedType) attribute.getClass().getAnnotatedSuperclass();
AnnotatedType annotatedType = cls.getAnnotatedActualTypeArguments()[1];
attrTypeHandler = TypeHandler.lookup(attributeType, annotatedType);
attributeSerializer = serialization.getSerializer(attrTypeHandler);
attributeDeserializer = serialization.getDeserializer(attrTypeHandler);
ResolvedType objectType = new TypeResolver().resolve(attribute.getEffectiveObjectType());
ObjectTypeHandler objectTypeHandler = (ObjectTypeHandler) TypeHandler.lookup(objectType, null);
if (!(objectTypeHandler instanceof ObjectTypeHandler)) {
throw new RuntimeException("Index " + attribute.getAttributeName() +
" is not an object, but " + objectType.getBriefDescription());
} else {
objectSerializer = serialization.getSerializer(objectTypeHandler.getWrappedClass());
objectDeserializer = serialization.getDeserializer(objectTypeHandler.getWrappedClass());
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy