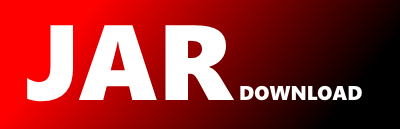
com.evrythng.thng.resource.model.store.Thng Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of thng-resource-model Show documentation
Show all versions of thng-resource-model Show documentation
Models for REST resources representations.
/*
* (c) Copyright 2012 EVRYTHNG Ltd London / Zurich
* www.evrythng.com
*/
package com.evrythng.thng.resource.model.store;
import com.evrythng.thng.resource.model.core.DurableResourceModel;
import java.util.Collections;
import java.util.HashMap;
import java.util.Map;
import java.util.Set;
/**
* Model representation for thngs.
*/
public class Thng extends DurableResourceModel implements ResourceWithProperties {
private static final long serialVersionUID = -5495600871904690325L;
private String name;
public static final String FIELD_NAME = "name";
private String description;
private EmbeddedLocation location;
/**
* Reference to {@link Product#id}.
*/
private String product;
private Map properties;
/**
* An array of global identifiers for this thng
*/
private Map identifiers;
/**
* An array of collection ids this thng is part of.
*/
private Set collections;
protected Long activatedAt;
public String getName() {
return name;
}
public void setName(final String name) {
this.name = name;
}
public String getDescription() {
return description;
}
public void setDescription(final String description) {
this.description = description;
}
public EmbeddedLocation getLocation() {
return location;
}
public void setLocation(final EmbeddedLocation location) {
this.location = location;
}
public String getProduct() {
return product;
}
public void setProduct(final String product) {
this.product = product;
}
@Override
public Map getProperties() {
return properties != null ? Collections.unmodifiableMap(properties) : null;
}
@Override
public void setProperties(final Map properties) {
this.properties = properties != null ? new HashMap<>(properties) : null;
}
@Override
public String toString() {
return "Thng [name=" + name + ", description=" + description + ", location=" + location + ", product=" + product + ", properties=" + properties + ", id=" + getId() + ", identifiers=" + identifiers + "]";
}
public void addIdentifier(final String type, final String value) {
if (identifiers == null) {
identifiers = new HashMap<>();
}
identifiers.put(type, value);
}
public Map getIdentifiers() {
return identifiers;
}
public void setIdentifiers(final Map identifiers) {
this.identifiers = identifiers;
}
public String firstIdentifier() {
return identifiers.values().iterator().next();
}
public Set getCollections() {
return collections;
}
public void setCollections(final Set collections) {
this.collections = collections;
}
public Long getActivatedAt() {
return activatedAt;
}
public void setActivatedAt(final Long activatedAt) {
this.activatedAt = activatedAt;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy