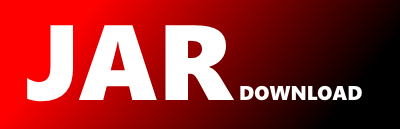
com.evrythng.thng.resource.model.store.jobs.Job Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of thng-resource-model Show documentation
Show all versions of thng-resource-model Show documentation
Models for REST resources representations.
/*
* (c) Copyright 2016 EVRYTHNG Ltd London / Zurich
* www.evrythng.com
*/
package com.evrythng.thng.resource.model.store.jobs;
import com.evrythng.thng.resource.model.core.DurableResourceModel;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
public abstract class Job
© 2015 - 2025 Weber Informatics LLC | Privacy Policy