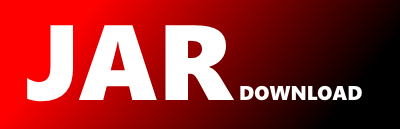
com.eworkcloud.log.LoghubAppender Maven / Gradle / Ivy
package com.eworkcloud.log;
import ch.qos.logback.classic.spi.IThrowableProxy;
import ch.qos.logback.classic.spi.LoggingEvent;
import ch.qos.logback.classic.spi.StackTraceElementProxy;
import ch.qos.logback.classic.spi.ThrowableProxyUtil;
import ch.qos.logback.core.CoreConstants;
import ch.qos.logback.core.UnsynchronizedAppenderBase;
import com.aliyun.openservices.aliyun.log.producer.LogProducer;
import com.aliyun.openservices.aliyun.log.producer.Producer;
import com.aliyun.openservices.aliyun.log.producer.ProducerConfig;
import com.aliyun.openservices.aliyun.log.producer.ProjectConfig;
import com.aliyun.openservices.log.common.LogItem;
import java.time.Instant;
import java.time.ZoneId;
import java.time.format.DateTimeFormatter;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
import java.util.Optional;
public class LoghubAppender extends UnsynchronizedAppenderBase {
private static final ZoneId timeZone = ZoneId.of("GMT+8");
private static final String timeFormat = "yyyy-MM-dd HH:mm:ss";
private static final DateTimeFormatter formatter = DateTimeFormatter.ofPattern(timeFormat).withZone(timeZone);
private ProducerConfig producerConfig = new ProducerConfig();
private Producer producer;
private String endpoint;
private String accessKeyId;
private String accessKeySecret;
private String project;
private String logStore;
private String topic = "";
private String source = "";
private String mdcFields;
@Override
public void start() {
if (endpoint == null || endpoint.endsWith("UNDEFINED")) {
return;
}
if (accessKeyId == null || accessKeyId.endsWith("UNDEFINED")) {
return;
}
if (accessKeySecret == null || accessKeySecret.endsWith("UNDEFINED")) {
return;
}
try {
producer = new LogProducer(producerConfig);
producer.putProjectConfig(new ProjectConfig(project, endpoint, accessKeyId, accessKeySecret));
super.start();
} catch (Exception ex) {
addError("Failed to start LoghubAppender.", ex);
}
}
@Override
public void stop() {
try {
if (!isStarted()) {
return;
}
super.stop();
producer.close();
} catch (Exception ex) {
addError("Failed to stop LoghubAppender.", ex);
}
}
@Override
public void append(E eventObject) {
try {
appendEvent(eventObject);
} catch (Exception ex) {
addError("Failed to append event.", ex);
}
}
private void appendEvent(E eventObject) {
if (!(eventObject instanceof LoggingEvent)) {
return;
}
LoggingEvent event = (LoggingEvent) eventObject;
List logItems = new ArrayList<>();
LogItem item = new LogItem();
logItems.add(item);
item.SetTime((int) (event.getTimeStamp() / 1000));
Instant instant = Instant.ofEpochMilli(event.getTimeStamp());
item.PushBack("time", formatter.format(instant));
item.PushBack("level", event.getLevel().toString());
item.PushBack("thread", event.getThreadName());
StackTraceElement[] caller = event.getCallerData();
if (caller != null && caller.length > 0) {
item.PushBack("location", caller[0].toString());
}
String message = event.getFormattedMessage();
item.PushBack("message", message);
IThrowableProxy iThrowableProxy = event.getThrowableProxy();
if (iThrowableProxy != null) {
String throwable = getExceptionInfo(iThrowableProxy);
throwable += fullDump(event.getThrowableProxy().getStackTraceElementProxyArray());
item.PushBack("throwable", throwable);
}
Optional.ofNullable(mdcFields).ifPresent(
f -> event.getMDCPropertyMap().entrySet().stream()
.filter(v -> Arrays.stream(f.split(",")).anyMatch(i -> i.equals(v.getKey())))
.forEach(map -> item.PushBack(map.getKey(), map.getValue()))
);
try {
producer.send(project, logStore, topic, source, logItems, new LoghubAppenderCallback<>(
this, project, logStore, topic, source, logItems)
);
} catch (Exception ex) {
this.addError(
"Failed to send log, project=" + project
+ ", logStore=" + logStore
+ ", topic=" + topic
+ ", source=" + source
+ ", logItem=" + logItems, ex);
}
}
private String getExceptionInfo(IThrowableProxy iThrowableProxy) {
String className = iThrowableProxy.getClassName();
String message = iThrowableProxy.getMessage();
return (message != null) ? (className + ": " + message) : className;
}
private String fullDump(StackTraceElementProxy[] stackTraceElementProxyArray) {
StringBuilder builder = new StringBuilder();
for (StackTraceElementProxy step : stackTraceElementProxyArray) {
builder.append(CoreConstants.LINE_SEPARATOR);
String string = step.toString();
builder.append(CoreConstants.TAB).append(string);
ThrowableProxyUtil.subjoinPackagingData(builder, step);
}
return builder.toString();
}
public String getLogStore() {
return logStore;
}
public void setLogStore(String logStore) {
this.logStore = logStore;
}
public String getTopic() {
return topic;
}
public void setTopic(String topic) {
if (topic == null || topic.endsWith("UNDEFINED")) {
this.topic = "";
} else {
this.topic = topic;
}
}
public String getSource() {
return source;
}
public void setSource(String source) {
if (source == null || source.endsWith("UNDEFINED")) {
this.source = "";
} else {
this.source = source;
}
}
public void setMdcFields(String mdcFields) {
if (mdcFields == null || mdcFields.endsWith("UNDEFINED")) {
this.mdcFields = null;
} else {
this.mdcFields = mdcFields;
}
}
// **** ==- ProjectConfig -== **********************
public String getProject() {
return project;
}
public void setProject(String project) {
this.project = project;
}
public String getEndpoint() {
return endpoint;
}
public void setEndpoint(String endpoint) {
this.endpoint = endpoint;
}
public String getAccessKeyId() {
return accessKeyId;
}
public void setAccessKeyId(String accessKeyId) {
this.accessKeyId = accessKeyId;
}
public String getAccessKeySecret() {
return accessKeySecret;
}
public void setAccessKeySecret(String accessKeySecret) {
this.accessKeySecret = accessKeySecret;
}
// **** ==- ProducerConfig -== **********************
public int getTotalSizeInBytes() {
return producerConfig.getTotalSizeInBytes();
}
public void setTotalSizeInBytes(int totalSizeInBytes) {
producerConfig.setTotalSizeInBytes(totalSizeInBytes);
}
public long getMaxBlockMs() {
return producerConfig.getMaxBlockMs();
}
public void setMaxBlockMs(long maxBlockMs) {
producerConfig.setMaxBlockMs(maxBlockMs);
}
public int getIoThreadCount() {
return producerConfig.getIoThreadCount();
}
public void setIoThreadCount(int ioThreadCount) {
producerConfig.setIoThreadCount(ioThreadCount);
}
public int getBatchSizeThresholdInBytes() {
return producerConfig.getBatchSizeThresholdInBytes();
}
public void setBatchSizeThresholdInBytes(int batchSizeThresholdInBytes) {
producerConfig.setBatchSizeThresholdInBytes(batchSizeThresholdInBytes);
}
public int getBatchCountThreshold() {
return producerConfig.getBatchCountThreshold();
}
public void setBatchCountThreshold(int batchCountThreshold) {
producerConfig.setBatchCountThreshold(batchCountThreshold);
}
public int getLingerMs() {
return producerConfig.getLingerMs();
}
public void setLingerMs(int lingerMs) {
producerConfig.setLingerMs(lingerMs);
}
public int getRetries() {
return producerConfig.getRetries();
}
public void setRetries(int retries) {
producerConfig.setRetries(retries);
}
public int getMaxReservedAttempts() {
return producerConfig.getMaxReservedAttempts();
}
public void setMaxReservedAttempts(int maxReservedAttempts) {
producerConfig.setMaxReservedAttempts(maxReservedAttempts);
}
public long getBaseRetryBackoffMs() {
return producerConfig.getBaseRetryBackoffMs();
}
public void setBaseRetryBackoffMs(long baseRetryBackoffMs) {
producerConfig.setBaseRetryBackoffMs(baseRetryBackoffMs);
}
public long getMaxRetryBackoffMs() {
return producerConfig.getMaxRetryBackoffMs();
}
public void setMaxRetryBackoffMs(long maxRetryBackoffMs) {
producerConfig.setMaxRetryBackoffMs(maxRetryBackoffMs);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy