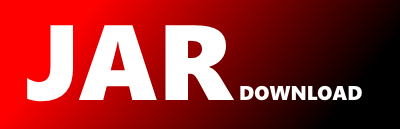
com.eworkcloud.web.util.SshClientUtils Maven / Gradle / Ivy
package com.eworkcloud.web.util;
import ch.ethz.ssh2.Connection;
import ch.ethz.ssh2.SFTPv3Client;
import ch.ethz.ssh2.SFTPv3DirectoryEntry;
import ch.ethz.ssh2.SFTPv3FileHandle;
import ch.ethz.ssh2.Session;
import ch.ethz.ssh2.StreamGobbler;
import lombok.extern.slf4j.Slf4j;
import org.apache.commons.io.IOUtils;
import org.springframework.util.ObjectUtils;
import org.springframework.util.StringUtils;
import java.io.BufferedInputStream;
import java.io.ByteArrayInputStream;
import java.io.ByteArrayOutputStream;
import java.io.File;
import java.io.FileInputStream;
import java.io.IOException;
import java.io.InputStream;
import java.nio.charset.StandardCharsets;
import java.util.ArrayList;
import java.util.List;
@Slf4j
public abstract class SshClientUtils {
/**
* 获取Connection,端口(默认:22)
*
* @param host 主机
* @param username 登录名称
* @return 连接
*/
public static Connection getConnection(String host, String username) {
return getConnection(host, 22, username);
}
/**
* 获取Connection
*
* @param host 主机
* @param port 端口
* @param username 登录名称
* @return 连接
*/
public static Connection getConnection(String host, int port, String username) {
return getConnection(host, port, username, null);
}
/**
* 获取Connection,端口(默认:22)
*
* @param host 主机
* @param username 登录名称
* @param password 登录密码
* @return 连接
*/
public static Connection getConnection(String host, String username, String password) {
return getConnection(host, 22, username, password);
}
/**
* 获取Connection
*
* @param host 主机
* @param port 端口
* @param username 登录名称
* @param password 登录密码
* @return 连接
*/
public static Connection getConnection(String host, int port, String username, String password) {
Connection connection = null;
try {
connection = new Connection(host, port);
connection.connect();
boolean isAuthenticate;
if (StringUtils.hasText(password)) {
isAuthenticate = connection.authenticateWithPassword(username, password);
} else {
isAuthenticate = connection.authenticateWithNone(username);
}
if (isAuthenticate) {
return connection;
} else {
connection.close();
throw new RuntimeException("Connection authentication failed.");
}
} catch (IOException ex) {
connection.close();
throw new RuntimeException(ex);
}
}
/*************************************** ssh **********************************************/
private static String processStdout(InputStream is) {
StringBuilder builder = new StringBuilder();
try {
InputStream stdout = new StreamGobbler(is);
char[] chars = IOUtils.toCharArray(stdout, StandardCharsets.UTF_8);
if (!ObjectUtils.isEmpty(chars)) {
builder.append(System.getProperty("line.separator"));
builder.append(chars);
}
} catch (IOException ex) {
log.error(ex.getMessage(), ex.getCause());
}
return builder.toString();
}
/**
* 执行SSH命令
*
* @param connection SSH连接
* @param command SSH命令
* @return 执行结果
*/
public static String execute(Connection connection, String command) {
Session session = null;
try {
session = connection.openSession();
session.execCommand(command);
String result = processStdout(session.getStdout());
if (StringUtils.isEmpty(result)) {
return processStdout(session.getStderr());
} else {
return result;
}
} catch (IOException ex) {
throw new RuntimeException(ex);
} finally {
if (null != session) {
session.close();
}
}
}
/*************************************** ftp **********************************************/
/**
* SFTP上传文件
*
* @param connection SSH连接
* @param file 文件
* @param path 远程路径
*/
public static void upLoad(Connection connection, File file, String path) {
try {
upLoad(connection, new BufferedInputStream(new FileInputStream(file)), path);
} catch (IOException ex) {
throw new RuntimeException(ex);
}
}
/**
* SFTP上传文件
*
* @param connection SSH连接
* @param input 文件流
* @param path 远程路径
*/
public static void upLoad(Connection connection, byte[] input, String path) {
upLoad(connection, new ByteArrayInputStream(input), path);
}
/**
* SFTP上传文件
*
* @param connection SSH连接
* @param input 文件流
* @param path 远程路径
*/
public static void upLoad(Connection connection, InputStream input, String path) {
SFTPv3Client client = null;
SFTPv3FileHandle handle = null;
try {
client = new SFTPv3Client(connection);
handle = client.createFile(path);
byte[] buffer = new byte[8192];
long offset = 0;
int count;
while ((count = input.read(buffer, 0, buffer.length)) >= 0) {
client.write(handle, offset, buffer, 0, count);
offset += count;
}
} catch (IOException ex) {
throw new RuntimeException(ex);
} finally {
if (null != client) {
if (null != handle) {
try {
client.closeFile(handle);
} catch (IOException ex) {
log.error(ex.getMessage(), ex.getCause());
}
}
client.close();
}
}
}
/**
* SFTP下载文件
*
* @param connection SSH连接
* @param path 远程路径
* @return 文件流
*/
public static byte[] download(Connection connection, String path) {
SFTPv3Client client = null;
SFTPv3FileHandle handle = null;
try {
client = new SFTPv3Client(connection);
handle = client.openFileRO(path);
ByteArrayOutputStream baos = new ByteArrayOutputStream();
byte[] buffer = new byte[8192];
long offset = 0;
int count;
while ((count = client.read(handle, offset, buffer, 0, buffer.length)) >= 0) {
baos.write(buffer, 0, count);
offset += count;
}
return baos.toByteArray();
} catch (IOException ex) {
throw new RuntimeException(ex);
} finally {
if (null != client) {
if (null != handle) {
try {
client.closeFile(handle);
} catch (IOException ex) {
log.error(ex.getMessage(), ex.getCause());
}
}
client.close();
}
}
}
/**
* SFTP名称列表
*
* @param connection SSH连接
* @param path 远程目录
* @return 名称列表
*/
public static List listNames(Connection connection, String path) {
SFTPv3Client client = null;
try {
client = new SFTPv3Client(connection);
List files = client.ls(path);
List nameList = new ArrayList<>();
for (SFTPv3DirectoryEntry entry : files) {
String fileName = entry.filename.trim();
if (!fileName.equals(".") && !fileName.equals("..")) {
nameList.add(fileName);
}
}
return nameList;
} catch (IOException ex) {
throw new RuntimeException(ex);
} finally {
if (null != client) {
client.close();
}
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy