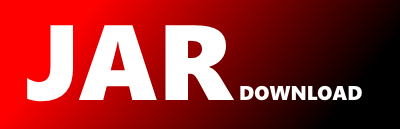
com.exadatum.xsuite.xmaven.bash.doc.BashDocMojo Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of bash-maven-plugin Show documentation
Show all versions of bash-maven-plugin Show documentation
Bash Maven Plugin is used to generate documentation as well as to run unit test for bash scripts.
package com.exadatum.xsuite.xmaven.bash.doc;
/*-
* #%L
* Exadatum Bash Function Document Generator
* %%
* Copyright (C) 2016 - 2017 Exadatum Software Services Pvt. Ltd.
* %%
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
* #L%
*/
import java.io.File;
import java.io.IOException;
import java.nio.file.Files;
import java.util.ArrayList;
import java.util.List;
import org.apache.maven.execution.MavenSession;
import org.apache.maven.plugin.AbstractMojo;
import org.apache.maven.plugin.MojoExecutionException;
import org.apache.maven.plugin.MojoFailureException;
import org.apache.maven.plugins.annotations.Component;
import org.apache.maven.plugins.annotations.Mojo;
import org.apache.maven.plugins.annotations.Parameter;
import org.apache.maven.project.MavenProject;
import org.apache.maven.shared.model.fileset.FileSet;
import org.apache.maven.shared.model.fileset.util.FileSetManager;
/**
* Generates documentation for bash functions & constants.
* It defines following specific structure for the function and constant
* documentation.
*
*
* #/**
* #* Write description for method or constant here.
* #*
* #* @author Name of the author. e.g. Abhijit Shingate
* #* @version Version of the function e.g. 1.0.0
* #* @since Since when the function is added. e.g. 10/12/2016
* #* @catagory Catagory to which this function belongs to. e.g. general, hadoop/hdfs,
* #* @param parameter parameter description e.g. dir_name directory name
* #* @scope Scope of the method. possible values are public, private
* #*\/
*
*
*
* Rules:
* Documentation block starts with 4 characters #/** as start tag
* Start tag shall always be on its own line
* Documentation block ends with 3 characters #*\/ as end tag
* End tag shall always be on its own line
* Documentation block continues with 2 characters #*
* Annotations start with @ symbol
* Parameter type can be any of file | number | string
* Scope of the method can be private | public
*
*
*
*/
@Mojo(name = "doc")
public class BashDocMojo extends AbstractMojo {
/**
* Current maven project
*/
@Component
private MavenProject mavenProject;
/**
* Current maven session
*/
@Component
private MavenSession mavenSession;
/**
* Document generator to be used. Plugin provides apache velocity based
* built in generator that generates document. Value of this property shall
* be fully qualified class name that implements {@link DocGenerator}
* interface
*/
@Parameter
private String docGeneratorClassName = DefaultDocPageGenerator.class.getName();
/**
* Template to be used to generate documentation page.By default plugin
* looks for README.md.template file in the project root dir to generate the
* doc. README.md.template shall if a velocity template to which tvar_docs
* variable shall be passed. tvar_docs is a list of {@link DocBlock}
* objects. See example template at src/test/resources/README.md.template
*/
@Parameter
private File docPageTemplate;
/**
* Output file to be generated by the doc generator
*/
@Parameter(defaultValue = "${basedir}/README.md")
private File outputDocFile;
/**
* List of files to be processed to generate documentation.
*/
@Parameter
private List fileSets = new ArrayList<>();
/*
* (non-Javadoc)
*
* @see org.apache.maven.plugin.Mojo#execute()
*/
@Override
public void execute() throws MojoExecutionException, MojoFailureException {
FileSetManager fileSetManager = new FileSetManager();
List docBlocks = new ArrayList<>();
for (FileSet fileSet : fileSets) {
String[] includedFiles = fileSetManager.getIncludedFiles(fileSet);
for (int i = 0; i < includedFiles.length; i++) {
File includedFile = new File(fileSet.getDirectory(), includedFiles[i]);
try {
docBlocks = generateDoc(includedFile);
} catch (Exception e) {
getLog().error("Failed to parse bash script " + includedFile.getAbsolutePath(), e);
}
}
}
if (docBlocks.isEmpty()) {
getLog().warn("No documentation blocks found");
}
DocGenerator docGenerator = getDocGenerator();
DocGeneratorContext context = new DocGeneratorContext();
context.setDocBlocks(docBlocks);
context.setOutputPage(outputDocFile);
context.setPageTemplate(docPageTemplate);
try {
docGenerator.generate(context);
} catch (IOException e) {
throw new RuntimeException(e);
}
}
private DocGenerator getDocGenerator() {
DocGenerator docGenerator = null;
try {
Object newInstance = Class.forName(docGeneratorClassName).newInstance();
if (newInstance instanceof DocGenerator) {
docGenerator = (DocGenerator) newInstance;
} else {
throw new RuntimeException(String.format(
"Invalid doc generator implementation [%s]. Doc generator shall implement [%s] interface ",
docGeneratorClassName, DocGenerator.class.getName()));
}
} catch (InstantiationException | IllegalAccessException | ClassNotFoundException e) {
throw new RuntimeException(e);
}
return docGenerator;
}
private List generateDoc(File bashScript) throws IOException {
List docs = new ArrayList<>();
String contents = getBashScriptContents(bashScript);
DocBlockIterator blockIterator = new DocBlockIterator(contents);
while (blockIterator.hasNext()) {
DocBlock docBlock = (DocBlock) blockIterator.next();
docs.add(docBlock);
}
return docs;
}
/**
* It is assumed that bash scripts are small files.
*
* @param bashScript
* @return contents of bash script
* @throws IOException
*/
private String getBashScriptContents(File bashScript) throws IOException {
String fileContents = new String(Files.readAllBytes(bashScript.toPath()));
return fileContents;
}
public static void main(String[] args) throws IOException {
BashDocMojo mojo = new BashDocMojo();
List generateDoc = mojo.generateDoc(new File(
"/Users/Shingate/Documents/Workspaces/XArchitect/bdoc-maven-plugin/src/test/resources/Sample.java"));
for (DocBlock documentation : generateDoc) {
System.out.println(documentation);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy