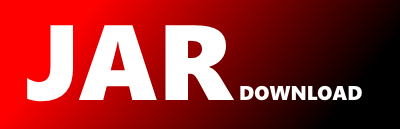
com.exasol.projectkeeper.config.ProjectKeeperRawConfig Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of project-keeper-core Show documentation
Show all versions of project-keeper-core Show documentation
Project keeper is a tool that verifies and fixes project setups.
package com.exasol.projectkeeper.config;
import java.util.List;
import java.util.Map;
/**
* Intermediate class for reading the config. This is used by {@link ProjectKeeperConfigReader}.
*
* SnakeYML requires this to be public.
*
*/
public class ProjectKeeperRawConfig {
private List sources;
private List linkReplacements;
private List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy