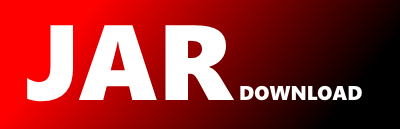
com.exasol.projectkeeper.xpath.XPathSplitter Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of project-keeper-core Show documentation
Show all versions of project-keeper-core Show documentation
Project keeper is a tool that verifies and fixes project setups.
package com.exasol.projectkeeper.xpath;
import java.util.ArrayList;
import java.util.List;
/**
* This class splits xPath expressions at the root level slashes.
*/
public class XPathSplitter {
private XPathSplitter() {
// empty on purpose
}
/**
* Split the xPath expression at the root level slashes.
*
* @param xPath xPath expression
* @return fragments
*/
public static List split(final String xPath) {
var nestLevel = 0;
final List fragments = new ArrayList<>();
var fragmentBuilder = new StringBuilder();
for (var position = 0; position < xPath.length(); position++) {
final var currentChar = xPath.charAt(position);
if (currentChar == '[') {
nestLevel++;
fragmentBuilder.append(currentChar);
} else if (currentChar == ']') {
nestLevel--;
fragmentBuilder.append(currentChar);
} else if (currentChar == '/' && position != 0 && nestLevel == 0) {
fragments.add(fragmentBuilder.toString());
fragmentBuilder = new StringBuilder();
} else {
fragmentBuilder.append(currentChar);
}
}
final var fragment = fragmentBuilder.toString();
if (!fragment.isEmpty()) {
fragments.add(fragment);
}
return fragments;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy