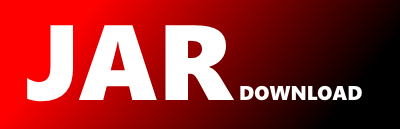
com.exasol.projectkeeper.shared.config.workflow.WorkflowStep Maven / Gradle / Ivy
The newest version!
package com.exasol.projectkeeper.shared.config.workflow;
import java.util.*;
/**
* A build step in a GitHub workflow.
*/
public final class WorkflowStep {
private final Map rawStep;
private WorkflowStep(final Map rawStep) {
this.rawStep = Objects.requireNonNull(rawStep, "rawStep");
}
/**
* Create a list of workflow steps from a list of raw steps parsed from a YAML file.
*
* @param setupSteps list of raw steps
* @return list of workflow steps
*/
public static List createSteps(final List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy