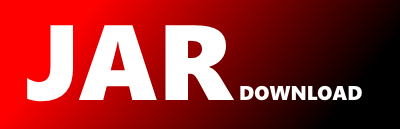
com.exasol.projectkeeper.shared.dependencies.ProjectDependency Maven / Gradle / Ivy
The newest version!
package com.exasol.projectkeeper.shared.dependencies;
import java.util.*;
import java.util.stream.Collectors;
/** Generic dependency. */
public final class ProjectDependency implements BaseDependency {
/**
* Create a new builder.
*
* @return Builder for new instances of {@link ProjectDependency}.
*/
public static Builder builder() {
return new Builder();
}
private Type type;
private String name;
private String websiteUrl;
private List licenses = new ArrayList<>();
/** Create an empty project dependency */
public ProjectDependency() {
}
/**
* Create a new instance of {@link ProjectDependency} with all fields defined
*
* @param type type of the dependency
* @param name name of the module of the dependency
* @param websiteUrl URL of the website associated with the current project dependency
* @param licenses list of licenses associated with the current project dependency
*/
public ProjectDependency(final Type type, final String name, final String websiteUrl,
final List licenses) {
this.type = type;
this.name = name;
this.websiteUrl = websiteUrl;
this.licenses = licenses;
}
/**
* Get type.
*
* @return type of the dependency
*/
@Override
public Type getType() {
return this.type;
}
/**
* Get name.
*
* @return name of the module of the dependency
*/
@Override
public String getName() {
return this.name;
}
/**
* Get website URL.
*
* @return URL of the website associated with the current project dependency
*/
public String getWebsiteUrl() {
return this.websiteUrl;
}
/**
* Get licenses.
*
* @return list of licenses associated with the current project dependency
*/
public List getLicenses() {
return this.licenses;
}
/**
* Only needed for serialization and deserialization.
*
* @param type type of the dependency
*/
public void setType(final Type type) {
this.type = type;
}
/**
* Only needed for serialization and deserialization.
*
* @param name name of the module of the dependency
*/
public void setName(final String name) {
this.name = name;
}
/**
* Only needed for serialization and deserialization.
*
* @param websiteUrl URL of the website associated with the current project dependency
*/
public void setWebsiteUrl(final String websiteUrl) {
this.websiteUrl = websiteUrl;
}
/**
* Only needed for serialization and deserialization.
*
* @param licenses list of licenses associated with the current project dependency
*/
public void setLicenses(final List licenses) {
this.licenses = licenses;
}
@Override
public int hashCode() {
return Objects.hash(this.licenses, this.name, this.type, this.websiteUrl);
}
@Override
public boolean equals(final Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (getClass() != obj.getClass()) {
return false;
}
final ProjectDependency other = (ProjectDependency) obj;
return Objects.equals(this.licenses, other.licenses) && Objects.equals(this.name, other.name)
&& (this.type == other.type) && Objects.equals(this.websiteUrl, other.websiteUrl);
}
@Override
public String toString() {
return this.type + " dependency '" + this.name + "', " + this.websiteUrl + ", " + this.licenses.size()
+ " licenses: " + this.licenses.stream().map(License::toString).collect(Collectors.joining(", "));
}
/** Builder for a new instance of {@link ProjectDependency} */
public static final class Builder {
private final ProjectDependency projectDependency = new ProjectDependency();
private Builder() {
}
/**
* Set type.
*
* @param type type of the dependency to build
* @return this for fluent programming
*/
public Builder type(final Type type) {
this.projectDependency.type = type;
return this;
}
/**
* Set name.
*
* @param name name of the dependency to build
* @return this for fluent programming
*/
public Builder name(final String name) {
this.projectDependency.name = name;
return this;
}
/**
* Set website URL.
*
* @param websiteUrl URL of the website for the dependency to build
* @return this for fluent programming
*/
public Builder websiteUrl(final String websiteUrl) {
this.projectDependency.websiteUrl = websiteUrl;
return this;
}
/**
* Set licenses.
*
* @param licenses list of licenses to associate with the dependency to build
* @return this for fluent programming
*/
public Builder licenses(final List licenses) {
this.projectDependency.licenses = licenses;
return this;
}
/**
* Build a new instance.
*
* @return new instance of {@link ProjectDependency}
*/
public ProjectDependency build() {
return this.projectDependency;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy