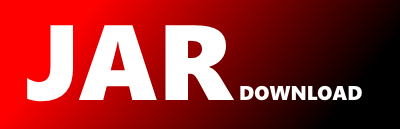
com.ebay.ejmask.core.util.CommonUtils Maven / Gradle / Ivy
package com.ebay.ejmask.core.util;
/**
* Copyright (c) 2023 eBay Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* https://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
import java.io.PrintWriter;
import java.io.StringWriter;
import java.lang.reflect.Array;
import java.util.Collection;
import java.util.Collections;
/**
* Set of utility methods inspired form apache commons
*
* @author prakv
*/
public class CommonUtils {
/**
* Null-safe check if the specified collection is empty.
*
* @param coll the collection to check, may be null
* @return true if empty or null
*/
public static boolean isEmpty(Collection coll) {
return (coll == null || coll.isEmpty());
}
/**
* Null-safe check if the specified collection is not empty.
*
* @param coll the collection to check, may be null
* @return true if non-null and non-empty
*/
public static boolean isNotEmpty(Collection coll) {
return !CommonUtils.isEmpty(coll);
}
/**
* Returns an immutable empty list if the argument is null
,
* or the argument itself otherwise.
*
* @param the element type
* @return an empty list if the argument is null
*/
public static Collection emptyIfNull(Collection collection) {
return isEmpty(collection) ? Collections.emptyList() : collection;
}
/**
* Checks if an array of Objects is not empty or not null
.
*
* @param array the array to test
* @return true
if the array is not empty or not null
* @since 2.5
*/
public static boolean isNotAnEmptyArray(Object array) {
return getLength(array) > 0;
}
/**
* Checks if an array of Objects is empty or not null
.
*
* @param array the array to test
* @return true
if the array is emptynull
* @since 2.5
*/
public static boolean isAnEmptyArray(Object array) {
return getLength(array) == 0;
}
/**
* Returns the length of the specified array.
*
* @param array the array to retrieve the length from, may be null
* @return The length of the array, or {@code 0} if the array is {@code null}
*/
private static int getLength(final Object array) {
return (array == null) ? 0 : Array.getLength(array);
}
/**
*
Checks if a CharSequence is not empty (""), not null and not whitespace only.
*
* Whitespace is defined by {@link Character#isWhitespace(char)}.
*
* @param cs the CharSequence to check, may be null
* @return {@code true} if the CharSequence is
* not empty and not null and not whitespace only
*/
public static boolean isNotBlank(final CharSequence cs) {
return !isBlank(cs);
}
/**
* Checks if a CharSequence is empty (""), null or whitespace only.
*
* Whitespace is defined by {@link Character#isWhitespace(char)}.
*
* @param cs the CharSequence to check, may be null
* @return {@code true} if the CharSequence is null, empty or whitespace only
*/
public static boolean isBlank(final CharSequence cs) {
final int strLen = cs == null ? 0 : cs.length();
if (strLen != 0) {
for (int i = 0; i < strLen; i++) {
if (!Character.isWhitespace(cs.charAt(i))) {
return false;
}
}
}
return true;
}
/**
* Gets the stack trace from a Throwable as a String.
*
* The result of this method vary by JDK version as this method
* uses {@link Throwable#printStackTrace(java.io.PrintWriter)}.
* On JDK1.3 and earlier, the cause exception will not be shown
* unless the specified throwable alters printStackTrace.
*
* @param throwable the Throwable
to be examined
* @return the stack trace as generated by the exception's
* printStackTrace(PrintWriter)
method
*/
public static String getStackTrace(Throwable throwable) {
StringWriter sw = new StringWriter();
PrintWriter pw = new PrintWriter(sw, true);
throwable.printStackTrace(pw);
return sw.getBuffer().toString();
}
}