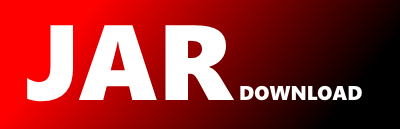
com.expleague.commons.seq.Seq Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of commons Show documentation
Show all versions of commons Show documentation
Utilities including math, charsequence based text processing, sequences etc.
package com.expleague.commons.seq;
import org.jetbrains.annotations.NotNull;
import java.lang.reflect.Array;
import java.util.Iterator;
import java.util.stream.BaseStream;
/**
* User: Manokk
* Date: 31.08.11
* Time: 2:45
*/
public interface Seq extends Iterable {
T at(int i);
Seq sub(int start, int end);
Seq sub(int[] indices);
int length();
boolean isImmutable();
Class extends T> elementType();
> S stream();
A toArray();
@SafeVarargs
static Seq of(T... arr) {
return new ArraySeq<>(arr);
}
abstract class Stub implements Seq {
@Override
public String toString() {
final StringBuilder builder = new StringBuilder();
final int length = length();
builder.append('[');
for (int i = 0; i < length; i++) {
if (i != 0)
builder.append(", ");
builder.append(at(i).toString());
}
builder.append(']');
return builder.toString();
}
private int hash = -1;
@Override
public int hashCode() {
if (hash != -1)
return hash;
int result = length();
result <<= 1;
for (int i = 0; i < length(); i++) {
result += at(i).hashCode();
result <<= 2;
}
if (result == -1)
result = 0;
return hash = result;
}
@Override
public boolean equals(Object obj) {
if (obj == this)
return true;
if (!(obj instanceof Seq))
return false;
final Seq other = (Seq)obj;
if (other.length() != length())
return false;
for (int i = 0; i < length(); i++) {
if (!at(i).equals(other.at(i)))
return false;
}
return true;
}
@Override
public A toArray() {
//noinspection unchecked
final T[] array = (T[])Array.newInstance(elementType(), length());
for (int i = 0; i < array.length; i++) {
array[i] = at(i);
}
//noinspection unchecked
return (A)array;
}
}
@NotNull
@Override
default Iterator iterator() {
return new Iterator() {
private int pos = 0;
@Override
public boolean hasNext() {
return pos < length();
}
@Override
public T next() {
return at(pos++);
}
};
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy