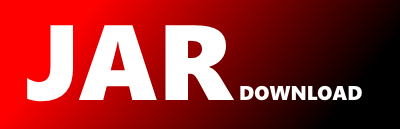
com.extjs.gxt.ui.client.widget.toolbar.ToolBar Maven / Gradle / Ivy
/*
* Ext GWT - Ext for GWT
* Copyright(c) 2007-2009, Ext JS, LLC.
* [email protected]
*
* http://extjs.com/license
*/
package com.extjs.gxt.ui.client.widget.toolbar;
import com.extjs.gxt.ui.client.Style;
import com.extjs.gxt.ui.client.Style.HorizontalAlignment;
import com.extjs.gxt.ui.client.event.BaseEvent;
import com.extjs.gxt.ui.client.event.ComponentEvent;
import com.extjs.gxt.ui.client.event.ContainerEvent;
import com.extjs.gxt.ui.client.event.ToolBarEvent;
import com.extjs.gxt.ui.client.widget.Component;
import com.extjs.gxt.ui.client.widget.Container;
import com.extjs.gxt.ui.client.widget.button.Button;
import com.extjs.gxt.ui.client.widget.layout.ToolBarLayout;
import com.google.gwt.user.client.DOM;
import com.google.gwt.user.client.Element;
import com.google.gwt.user.client.Event;
/**
* A standard tool bar.
*
* Events:
*
* BeforeAdd : ToolBarEvent(container, item, index)
* Fires before a item is added or inserted. Listeners can cancel the
* action by calling {@link BaseEvent#setCancelled(boolean)}.
*
* - container : this
* - item : the item being added
* - index : the index at which the item will be added
*
*
*
* BeforeRemove : ToolBarEvent(container, item)
* Fires before a item is removed. Listeners can cancel the action by
* calling {@link BaseEvent#setCancelled(boolean)}.
*
* - container : this
* - item : the item being removed
*
*
*
* Add : ToolBarEvent(container, item, index)
* Fires after a item has been added or inserted.
*
* - container : this
* - item : the item that was added
* - index : the index at which the item will be added
*
*
*
* Remove : ToolBarEvent(container, item)
* Fires after a item has been removed.
*
* - container : this
* - item : the item being removed
*
*
*
*
*
* - Inherited Events:
* - BoxComponent Move
* - BoxComponent Resize
* - Component Enable
* - Component Disable
* - Component BeforeHide
* - Component Hide
* - Component BeforeShow
* - Component Show
* - Component Attach
* - Component Detach
* - Component BeforeRender
* - Component Render
* - Component BrowserEvent
* - Component BeforeStateRestore
* - Component StateRestore
* - Component BeforeStateSave
* - Component SaveState
*
*
*
* - CSS:
* - x-toolbar (the tool bar)
*
*/
public class ToolBar extends Container {
private HorizontalAlignment alignment = HorizontalAlignment.LEFT;
private int minButtonWidth = Style.DEFAULT;
private boolean enableOverflow = true;
private int spacing = 0;
/**
* Creates a new tool bar.
*/
public ToolBar() {
setLayoutOnChange(true);
enableLayout = true;
addStyleName("x-toolbar");
setLayout(new ToolBarLayout());
}
/**
* Adds a item to the tool bar.
*
* @param item the item to add
*/
@Override
public boolean add(Component item) {
return super.add(item);
}
/**
* Returns the alignment of the items.
*
* @return the alignment
*/
public HorizontalAlignment getAlignment() {
return alignment;
}
/**
* Returns the min button width.
*
* @return the min button width
*/
public int getMinButtonWidth() {
return minButtonWidth;
}
/**
* Returns the child component spacing.
*
* @return the spacing
*/
public int getSpacing() {
return spacing;
}
/**
* Inserts a item into the tool bar.
*
* @param item the item to add
* @param index the insert location
*/
public boolean insert(Component item, int index) {
boolean result = super.insert(item, index);
if (item instanceof Button && ((Button) item).getMinWidth() == Style.DEFAULT) {
((Button) item).setMinWidth(minButtonWidth);
}
return result;
}
/**
* Returns true if overflow is enabled.
*
* @return the overflow state
*/
public boolean isEnableOverflow() {
return enableOverflow;
}
@Override
public boolean layout() {
return super.layout();
}
/**
* Removes a component from the tool bar.
*
* @param item the item to be removed
*/
public boolean remove(Component item) {
return super.remove(item);
}
/**
* Sets the ailgnment of the items. (defaults to LEFT, pre-render).
*
* @param alignment the alignment to set
*/
public void setAlignment(HorizontalAlignment alignment) {
assertPreRender();
this.alignment = alignment;
}
/**
* Sets the minWidth for any Component of type Button
*
* @param minButtonWidth the min button width to set
*/
public void setMinButtonWidth(int minButtonWidth) {
this.minButtonWidth = minButtonWidth;
for (Component c : getItems()) {
if (c instanceof Button && ((Button) c).getMinWidth() == Style.DEFAULT) {
((Button) c).setMinWidth(minButtonWidth);
}
}
}
/**
* True to show a drop down icon when the available width is less than the
* required width (defaults to false).
*
* @param enableOverflow true to enable overflow support
*/
public void setEnableOverflow(boolean enableOverflow) {
this.enableOverflow = enableOverflow;
}
/**
* Sets the spacing between child items (defaults to 0).
*
* @param spacing the spacing
*/
public void setSpacing(int spacing) {
this.spacing = spacing;
((ToolBarLayout) getLayout()).setSpacing(spacing);
}
@Override
protected ComponentEvent createComponentEvent(Event event) {
return new ToolBarEvent(this);
}
@Override
@SuppressWarnings("unchecked")
protected ContainerEvent createContainerEvent(Component item) {
return new ToolBarEvent(this, item);
}
protected void onRender(Element target, int index) {
setElement(DOM.createDiv(), target, index);
super.onRender(target, index);
addStyleName("x-small-editor");
if (alignment.equals(HorizontalAlignment.CENTER)) {
addStyleName("x-panel-btns-center");
} else if (alignment.equals(HorizontalAlignment.RIGHT)) {
if (getItemCount() == 0 || (getItemCount() > 0 && !(getItem(0) instanceof FillToolItem))) {
boolean state = layoutOnChange;
layoutOnChange = false;
insert(new FillToolItem(), 0);
layoutOnChange = state;
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy