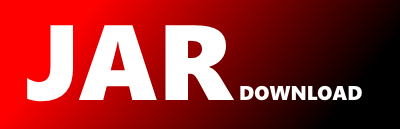
com.extjs.gxt.ui.client.widget.grid.RowEditor Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of gxt Show documentation
Show all versions of gxt Show documentation
Rich Internet Application Framework for GWT
/*
* Sencha GXT 2.3.0 - Sencha for GWT
* Copyright(c) 2007-2013, Sencha, Inc.
* [email protected]
*
* http://www.sencha.com/products/gxt/license/
*/
package com.extjs.gxt.ui.client.widget.grid;
import java.util.Map;
import com.extjs.gxt.ui.client.GXT;
import com.extjs.gxt.ui.client.Style;
import com.extjs.gxt.ui.client.core.El;
import com.extjs.gxt.ui.client.core.FastMap;
import com.extjs.gxt.ui.client.core.XDOM;
import com.extjs.gxt.ui.client.data.ModelData;
import com.extjs.gxt.ui.client.event.BaseEvent;
import com.extjs.gxt.ui.client.event.ButtonEvent;
import com.extjs.gxt.ui.client.event.ColumnModelEvent;
import com.extjs.gxt.ui.client.event.ComponentEvent;
import com.extjs.gxt.ui.client.event.Events;
import com.extjs.gxt.ui.client.event.GridEvent;
import com.extjs.gxt.ui.client.event.Listener;
import com.extjs.gxt.ui.client.event.RowEditorEvent;
import com.extjs.gxt.ui.client.event.SelectionListener;
import com.extjs.gxt.ui.client.store.Record;
import com.extjs.gxt.ui.client.util.KeyNav;
import com.extjs.gxt.ui.client.util.Margins;
import com.extjs.gxt.ui.client.util.Point;
import com.extjs.gxt.ui.client.widget.Component;
import com.extjs.gxt.ui.client.widget.ComponentHelper;
import com.extjs.gxt.ui.client.widget.ComponentManager;
import com.extjs.gxt.ui.client.widget.ComponentPlugin;
import com.extjs.gxt.ui.client.widget.ContentPanel;
import com.extjs.gxt.ui.client.widget.Html;
import com.extjs.gxt.ui.client.widget.button.Button;
import com.extjs.gxt.ui.client.widget.form.Field;
import com.extjs.gxt.ui.client.widget.form.LabelField;
import com.extjs.gxt.ui.client.widget.form.TriggerField;
import com.extjs.gxt.ui.client.widget.grid.EditorGrid.ClicksToEdit;
import com.extjs.gxt.ui.client.widget.layout.HBoxLayout;
import com.extjs.gxt.ui.client.widget.layout.HBoxLayoutData;
import com.extjs.gxt.ui.client.widget.layout.MarginData;
import com.extjs.gxt.ui.client.widget.layout.TableLayout;
import com.extjs.gxt.ui.client.widget.tips.ToolTip;
import com.extjs.gxt.ui.client.widget.tips.ToolTipConfig;
import com.google.gwt.event.dom.client.KeyCodes;
import com.google.gwt.user.client.Command;
import com.google.gwt.user.client.DeferredCommand;
import com.google.gwt.user.client.Element;
import com.google.gwt.user.client.Timer;
import com.google.gwt.user.client.ui.Widget;
/**
* This RowEditor should be used as a plugin to {@link Grid}. It displays an
* editor for all cells in a row.
*
*
* - Events:
*
* - BeforeEdit : RowEditorEvent(rowEditor, rowIndex)
* Fires before row editing is triggered. Listeners can cancel the action
* by calling {@link BaseEvent#setCancelled(boolean)}.
*
* - rowEditor : this
* - rowIndex : the row index of the row about to be edited
*
*
*
* - ValidateEdit : RowEditorEvent(rowEditor, rowIndex, changes)
* Fires right before the model is updated. Listeners can cancel the action
* by calling {@link BaseEvent#setCancelled(boolean)}.
*
* - rowEditor : this
* - rowIndex : the row index of the row about to be edited
* - changes : a map of property name and new values
*
*
*
* - AfterEdit : RowEditorEvent(rowEditor, rowIndex, changes)
* Fires after a row has been edited.
*
* - rowEditor : this
* - rowIndex : the row index of the row that was edited
* - changes : a map of property name and new values
*
*
*
*
* @param the model type
*/
@SuppressWarnings("deprecation")
public class RowEditor extends ContentPanel implements ComponentPlugin {
public class RowEditorMessages {
private String cancelText = GXT.MESSAGES.rowEditor_cancelText();
private String dirtyText = GXT.MESSAGES.rowEditor_dirtyText();
private String errorTipTitleText = GXT.MESSAGES.rowEditor_tipTitleText();
private String saveText = GXT.MESSAGES.rowEditor_saveText();
/**
* Returns the buttons cancel text.
*
* @return the text
*/
public String getCancelText() {
return cancelText;
}
/**
* Returns the tool tip dirty text.
*
* @return the dirtyText
*/
public String getDirtyText() {
return dirtyText;
}
/**
* Returns the error tool tip title.
*
* @return the errorTipTitleText
*/
public String getErrorTipTitleText() {
return errorTipTitleText;
}
/**
* Returns the buttons save text.
*
* @return the text
*/
public String getSaveText() {
return saveText;
}
/**
* Sets the buttons cancel text
*
* @param cancelText the cancel text
*/
public void setCancelText(String cancelText) {
this.cancelText = cancelText;
}
/**
* Sets the tool tip dirty text.
*
* @param dirtyText the dirtyText to set
*/
public void setDirtyText(String dirtyText) {
this.dirtyText = dirtyText;
}
/**
* Sets the error tool tip title.
*
* @param errorTipTitleText the errorTipTitleText to set
*/
public void setErrorTipTitleText(String errorTipTitleText) {
this.errorTipTitleText = errorTipTitleText;
}
/**
* Sets the buttons save text
*
* @param saveText the save text
*/
public void setSaveText(String saveText) {
this.saveText = saveText;
}
}
protected ContentPanel btns;
protected Grid grid;
protected RowEditorMessages messages;
protected boolean renderButtons = true;
protected int rowIndex;
protected Button saveBtn, cancelBtn;
private boolean bound;
private int buttonPad = 3;
private ClicksToEdit clicksToEdit = ClicksToEdit.ONE;
private boolean editing;
private boolean errorSummary = true;
private int frameWidth = 5;
private boolean initialized;
private boolean lastValid;
private Listener> listener;
private int monitorPoll = 200;
private Timer monitorTimer;
private boolean monitorValid = true;
private Record record;
private ToolTip tooltip;
protected Html toolTipAlignWidget;
public RowEditor() {
super();
setFooter(true);
setLayout(new HBoxLayout());
addStyleName("x-small-editor");
baseStyle = "x-row-editor";
messages = new RowEditorMessages();
}
/**
* Returns the clicks to edit.
*
* @return the clicks to edit
*/
public ClicksToEdit getClicksToEdit() {
return clicksToEdit;
}
/**
* Returns the roweditors's messages.
*
* @return the messages
*/
public RowEditorMessages getMessages() {
return messages;
}
/**
* Returns the interval in ms in that the roweditor is validated
*
* @return the interval in ms in that the roweditor is validated
*/
public int getMonitorPoll() {
return monitorPoll;
}
@SuppressWarnings("unchecked")
public void init(Component component) {
grid = (Grid) component;
grid.disableTextSelection(false);
listener = new Listener>() {
public void handleEvent(GridEvent be) {
if (be.getType() == Events.RowDoubleClick) {
onRowDblClick(be);
} else if (be.getType() == Events.RowClick) {
onRowClick(be);
} else if (be.getType() == Events.OnKeyDown) {
onGridKey(be);
} else if (be.getType() == Events.ColumnResize || be.getType() == Events.Resize) {
verifyLayout(false);
} else if (be.getType() == Events.BodyScroll) {
positionButtons();
} else if (be.getType() == Events.Detach) {
stopEditing(false);
} else if (be.getType() == Events.Reconfigure && initialized) {
stopEditing(false);
removeAll();
initialized = false;
}
}
};
grid.addListener(Events.RowDoubleClick, listener);
grid.addListener(Events.Resize, listener);
grid.addListener(Events.RowClick, listener);
grid.addListener(Events.OnKeyDown, listener);
grid.addListener(Events.ColumnResize, listener);
grid.addListener(Events.BodyScroll, listener);
grid.addListener(Events.Detach, listener);
grid.addListener(Events.Reconfigure, listener);
grid.getColumnModel().addListener(Events.HiddenChange, new Listener() {
public void handleEvent(ColumnModelEvent be) {
verifyLayout(false);
}
});
grid.getColumnModel().addListener(Events.ColumnMove, new Listener() {
public void handleEvent(ColumnModelEvent be) {
if (initialized) {
stopEditing(false);
removeAll();
initialized = false;
}
}
});
grid.getView().addListener(Events.Refresh, new Listener() {
public void handleEvent(BaseEvent be) {
stopEditing(false);
}
});
}
/**
* Returns true of the RowEditor is active and editing.
*
* @return true if the RowEditor is active
*/
public boolean isEditing() {
return editing;
}
/**
* Returns true if a tooltip with an error summary is shown.
*
* @return true if a tooltip with an error summary is shown
*/
public boolean isErrorSummary() {
return errorSummary;
}
/**
* Returns true if this roweditor is monitored.
*
* @return true if the roweditor is monitored
*/
public boolean isMonitorValid() {
return monitorValid;
}
@Override
public void onComponentEvent(ComponentEvent ce) {
super.onComponentEvent(ce);
if (ce.getEventTypeInt() == KeyNav.getKeyEvent().getEventCode()) {
if (ce.getKeyCode() == KeyCodes.KEY_ENTER) {
onEnter(ce);
} else if (ce.getKeyCode() == KeyCodes.KEY_ESCAPE) {
onEscape(ce);
} else if (ce.getKeyCode() == KeyCodes.KEY_TAB) {
onTab(ce);
}
}
}
/**
* Sets the number of clicks to edit (defaults to ONE).
*
* @param clicksToEdit the clicks to edit
*/
public void setClicksToEdit(ClicksToEdit clicksToEdit) {
this.clicksToEdit = clicksToEdit;
}
/**
* True to show a tooltip with an error summary (defaults to true)
*
* @param errorSummary true to show an error summary.
*/
public void setErrorSummary(boolean errorSummary) {
this.errorSummary = errorSummary;
}
/**
* Sets the roweditors's messages.
*
* @param messages the messages
*/
public void setMessages(RowEditorMessages messages) {
this.messages = messages;
}
/**
* Sets the polling interval in ms in that the roweditor validation is done
* (defaults to 200)
*
* @param monitorPoll the polling interval in ms in that validation is done
*/
public void setMonitorPoll(int monitorPoll) {
this.monitorPoll = monitorPoll;
}
/**
* True to monitor the valid status of this roweditor (defaults to true)
*
* @param monitorValid true to monitor this roweditor
*/
public void setMonitorValid(boolean monitorValid) {
this.monitorValid = monitorValid;
}
/**
* Start editing of a specific row.
*
* @param rowIndex the index of the row to edit.
* @param doFocus true to focus the field
*/
@SuppressWarnings("unchecked")
public void startEditing(int rowIndex, boolean doFocus) {
if (disabled) {
return;
}
if (editing && isDirty()) {
showTooltip(getMessages().getDirtyText());
return;
}
hideTooltip();
M model = (M) grid.getStore().getAt(rowIndex);
Record r = getRecord(model);
RowEditorEvent ree = new RowEditorEvent(this, rowIndex);
ree.setRecord(r);
Element row = (Element) grid.getView().getRow(rowIndex);
if (row == null || model == null || !fireEvent(Events.BeforeEdit, ree)) {
return;
}
editing = true;
record = r;
this.rowIndex = rowIndex;
if (!isRendered()) {
render((Element) grid.getView().getEditorParent());
}
ComponentHelper.doAttach(this);
if (!initialized) {
initFields();
}
ColumnModel cm = grid.getColumnModel();
for (int i = 0, len = cm.getColumnCount(); i < len; i++) {
Field
© 2015 - 2025 Weber Informatics LLC | Privacy Policy