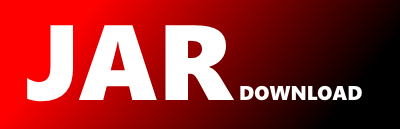
com.extjs.gxt.ui.client.widget.grid.GroupSummaryView Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of gxt Show documentation
Show all versions of gxt Show documentation
Rich Internet Application Framework for GWT
Please wait ...
© 2015 - 2025 Weber Informatics LLC | Privacy Policy