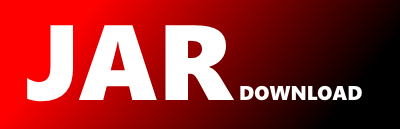
com.extjs.gxt.ui.client.widget.grid.AggregationRowConfig Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of gxt Show documentation
Show all versions of gxt Show documentation
Rich Internet Application Framework for GWT
/*
* Sencha GXT 2.3.1 - Sencha for GWT
* Copyright(c) 2007-2013, Sencha, Inc.
* [email protected]
*
* http://www.sencha.com/products/gxt/license/
*/
package com.extjs.gxt.ui.client.widget.grid;
import java.util.HashMap;
import java.util.Map;
import com.extjs.gxt.ui.client.data.ModelData;
import com.google.gwt.i18n.client.NumberFormat;
import com.google.gwt.user.client.ui.Widget;
/**
* Defines the configuration information for an aggregation row. The values for
* each column can be configured and calculated in several ways. The following
* methods are supported in order of precedence.
*
*
* - A static HTML string can be specified.
* - A widget can be specified.
* - A
SummaryType
can be used to "calculate" a value based on
* the data. When using a summary type, EITHER a
* NumberFormat
or a AggregationRenderer
can be used
* to "format" the value.
*
*
*
* In some cases, the values will not be calculated by the data stored in the
* store, for example, with paging. In this situation, there are 2 ways to
* provide values.
*
*
* The first method is to specify a AggregationRenderer
for the
* relevant columns. This renderer can any data as needed. The second method is
* to provide a GXT Model
that contains the values for the
* aggregation row. In this case, the value will be retrieved from the model
* using the property name associated with the column. With this method, you are
* responsible for ensuring the model is updated any time the aggregation values
* are updated.
*
* @param the model type
*/
public class AggregationRowConfig {
private Map> renderers;
private Map> types;
private Map formats;
private Map widgets;
private Map texts;
private Map cellStyle;
private ModelData model;
/**
* Creates a new aggregation row config.s
*/
public AggregationRowConfig() {
renderers = new HashMap>();
types = new HashMap>();
formats = new HashMap();
texts = new HashMap();
widgets = new HashMap();
cellStyle = new HashMap();
}
/**
* Returns the cell style for the given column.
*
* @param id the column id
* @return the CSS style name
*/
public String getCellStyle(String id) {
return cellStyle.get(id);
}
/**
* Returns the html for the given column.
*
* @param id the column id
* @return the column
*/
public String getHtml(String id) {
return texts.get(id);
}
/**
* Returns the model.
*
* @return the model
*/
public ModelData getModel() {
return model;
}
/**
* Returns the aggregation renderer for the given column.
*
* @param id the column id
* @return the aggregation renderer
*/
public AggregationRenderer getRenderer(String id) {
return renderers.get(id);
}
/**
* Returns the summary format for the given column.
*
* @param id the column id
* @return the summary format
*/
public NumberFormat getSummaryFormat(String id) {
return formats.get(id);
}
/**
* Returns the summary type for the given column.
*
* @param id the column id
* @return the summary type
*/
public SummaryType> getSummaryType(String id) {
return types.get(id);
}
/**
* Returns the widget for the given column.
*
* @param id the column id
* @return the widget
*/
public Widget getWidget(String id) {
return widgets.get(id);
}
/**
* Sets the cell style for the given column.
*
* @param id the column id
* @param style the CSS style name
*/
public void setCellStyle(String id, String style) {
cellStyle.put(id, style);
}
/**
* Sets the static HTML for the given column.
*
* @param id the column id
* @param html the html
*/
public void setHtml(String id, String html) {
texts.put(id, html);
}
/**
* Sets the model that contains the values for the aggregation row. It is
* expected, the model contains a value for the properties which match the
* column configuration data index. If the value is a Number, the number
* format and renderer will be used if specified. toString will be called on
* any other type.
*
* @param model the model
*/
public void setModel(ModelData model) {
this.model = model;
}
/**
* Sets the aggregation renderer for the given column.
*
* @param id the column id
* @param renderer the renderer
*/
public void setRenderer(String id, AggregationRenderer renderer) {
renderers.put(id, renderer);
}
/**
* Sets the number format for the given column. Only applies when specifying a
* SummaryType
.
*
* @param id the column id
* @param format the number format
*/
public void setSummaryFormat(String id, NumberFormat format) {
formats.put(id, format);
}
/**
* Sets the summary type for the given column.
*
* @param id the column id
* @param type the summary type
*/
public void setSummaryType(String id, SummaryType> type) {
types.put(id, type);
}
/**
* Sets the widget for the given column.
*
* @param id the column id
* @param widget the widget
*/
public void setWidget(String id, Widget widget) {
widgets.put(id, widget);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy