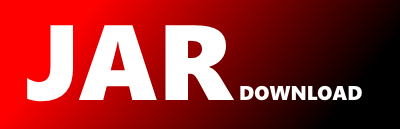
com.extjs.gxt.ui.client.data.BaseModel Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of gxt Show documentation
Show all versions of gxt Show documentation
Rich Internet Application Framework for GWT
The newest version!
/*
* Sencha GXT 2.3.1 - Sencha for GWT
* Copyright(c) 2007-2013, Sencha, Inc.
* [email protected]
*
* http://www.sencha.com/products/gxt/license/
*/
package com.extjs.gxt.ui.client.data;
import java.io.Serializable;
import java.util.List;
import java.util.Map;
import com.extjs.gxt.ui.client.util.Util;
/**
* Models
are generic data structures that notify listeners when
* changed. The structure allows a form of 'introspection' as all property names
* and values can be queried and retrieved at runtime.
*
*
* All events fired by the model will bubble to all parents.
*
*
*
* Model objects implement Serializable
and can therefore be used
* with GWT RPC. A model's children are not marked transient and will be passed
* in remote procedure calls.
*
*
*
* - Events:
*
* - Model.Add : (source, item)
* Fires after the button is selected.
*
* - source : this
* - item : add item
*
*
*
* - Model.Insert : (source, item)
* Fires after the button is selected.
*
* - source : this
* - item : insert item
* - index : insert index
*
*
*
* - Model.Update : (source, item)
* Fires after the button is selected.
*
* - source : this
* - item : this
*
*
*
*
* @see ChangeListener
* @see Serializable
*/
public class BaseModel extends BaseModelData implements Model, Serializable {
protected transient ChangeEventSupport changeEventSupport;
/**
* Creates a new base model.
*/
public BaseModel() {
changeEventSupport = new ChangeEventSupport();
}
/**
* Creates a new base model.
*
* @param properties the initial values
*/
public BaseModel(Map properties) {
this();
setProperties(properties);
}
/**
* Adds a listener to receive change events.
*
* @param listener the listener to be added
*/
public void addChangeListener(ChangeListener... listener) {
changeEventSupport.addChangeListener(listener);
}
/**
* Adds the listeners to receive change events.
*
* @param listeners the listeners to add
*/
public void addChangeListener(List listeners) {
for (ChangeListener listener : listeners) {
changeEventSupport.addChangeListener(listener);
}
}
/**
* Returns true if change events are disabled.
*
* @return true if silent
*/
public boolean isSilent() {
return changeEventSupport.isSilent();
}
public void notify(ChangeEvent evt) {
changeEventSupport.notify(evt);
}
@Override
@SuppressWarnings("unchecked")
public X remove(String name) {
if (map != null && map.containsKey(name)) {
X oldValue = (X) super.remove(name);
notifyPropertyChanged(name, null, oldValue);
return oldValue;
}
return null;
}
/**
* Removes a previously added change listener.
*
* @param listener the listener to be removed
*/
public void removeChangeListener(ChangeListener... listener) {
changeEventSupport.removeChangeListener(listener);
}
public void removeChangeListeners() {
changeEventSupport.removeChangeListeners();
}
@Override
public X set(String name, X value) {
X oldValue = super.set(name, value);
notifyPropertyChanged(name, value, oldValue);
return oldValue;
}
public void setSilent(boolean silent) {
changeEventSupport.setSilent(silent);
}
protected void fireEvent(int type) {
notify(new ChangeEvent(type, this));
}
protected void fireEvent(int type, Model item) {
notify(new ChangeEvent(type, this, item));
}
protected void notifyPropertyChanged(String name, Object value, Object oldValue) {
if (!Util.equalWithNull(value, oldValue)) {
notify(new PropertyChangeEvent(Update, this, name, oldValue, value));
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy