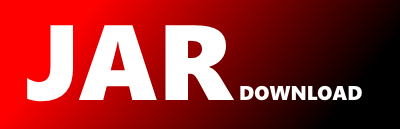
com.extjs.gxt.ui.client.widget.button.IconButton Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of gxt Show documentation
Show all versions of gxt Show documentation
Rich Internet Application Framework for GWT
The newest version!
/*
* Sencha GXT 2.3.1 - Sencha for GWT
* Copyright(c) 2007-2013, Sencha, Inc.
* [email protected]
*
* http://www.sencha.com/products/gxt/license/
*/
package com.extjs.gxt.ui.client.widget.button;
import com.extjs.gxt.ui.client.GXT;
import com.extjs.gxt.ui.client.aria.FocusFrame;
import com.extjs.gxt.ui.client.event.ComponentEvent;
import com.extjs.gxt.ui.client.event.Events;
import com.extjs.gxt.ui.client.event.IconButtonEvent;
import com.extjs.gxt.ui.client.event.SelectionListener;
import com.extjs.gxt.ui.client.widget.BoxComponent;
import com.google.gwt.event.dom.client.KeyCodes;
import com.google.gwt.user.client.DOM;
import com.google.gwt.user.client.Element;
import com.google.gwt.user.client.Event;
import com.google.gwt.user.client.ui.Accessibility;
/**
* A simple css styled button with 3 states: normal, over, and disabled.
*
*
* Note: To change the icon style after construction use
* {@link #changeStyle(String)}.
*
*
* - Events:
*
* - Select : IconButtonEvent(iconButton, event)
* Fires after the item is selected.
*
* - iconButton : this
* - event : the dom event
*
*
*
*/
public class IconButton extends BoxComponent {
protected String style;
protected boolean cancelBubble = true;
/**
* Creates a new icon button. The 'over' style and 'disabled' style names
* determined by adding '-over' and '-disabled' to the base style name.
*
* @param style the base style
*/
public IconButton(String style) {
this.style = style;
}
/**
* Creates a new icon button. The 'over' style and 'disabled' style names
* determined by adding '-over' and '-disabled' to the base style name.
*
* @param style the base style
* @param listener the click listener
*/
public IconButton(String style, SelectionListener listener) {
this(style);
addSelectionListener(listener);
}
/**
* @param listener
*/
public void addSelectionListener(SelectionListener listener) {
addListener(Events.Select, listener);
}
/**
* Changes the icon style.
*
* @param style the new icon style
*/
public void changeStyle(String style) {
removeStyleName(this.style);
removeStyleName(this.style + "-over");
removeStyleName(this.style + "-disabled");
addStyleName(style);
this.style = style;
}
public void onComponentEvent(ComponentEvent ce) {
switch (ce.getEventTypeInt()) {
case Event.ONMOUSEOVER:
addStyleName(style + "-over");
break;
case Event.ONMOUSEOUT:
removeStyleName(style + "-over");
break;
case Event.ONCLICK:
onClick(ce);
break;
case Event.ONFOCUS:
onFocus(ce);
break;
case Event.ONBLUR:
onBlur(ce);
break;
case Event.ONKEYUP:
onKeyPress(ce);
break;
}
}
/**
* Removes a previously added listener.
*
* @param listener the listener to be removed
*/
public void removeSelectionListener(SelectionListener listener) {
removeListener(Events.Select, listener);
}
@Override
protected ComponentEvent createComponentEvent(Event event) {
return new IconButtonEvent(this, event);
}
protected void onBlur(ComponentEvent ce) {
if (GXT.isFocusManagerEnabled()) {
FocusFrame.get().unframe();
}
}
protected void onClick(ComponentEvent ce) {
if (cancelBubble) {
ce.cancelBubble();
}
removeStyleName(style + "-over");
fireEvent(Events.Select, ce);
}
protected void onDisable() {
addStyleName(style + "-disabled");
}
protected void onEnable() {
removeStyleName(style + "-disabled");
}
protected void onFocus(ComponentEvent ce) {
if (GXT.isFocusManagerEnabled() && !GXT.isIE) {
FocusFrame.get().frame(this);
}
}
protected void onKeyPress(ComponentEvent ce) {
int code = ce.getKeyCode();
if (code == KeyCodes.KEY_ENTER || code == 32) {
onClick(ce);
}
}
protected void onRender(Element target, int index) {
setElement(DOM.createDiv(), target, index);
addStyleName("x-icon-btn");
addStyleName("x-nodrag");
addStyleName(style);
sinkEvents(Event.ONCLICK | Event.MOUSEEVENTS | Event.FOCUSEVENTS | Event.ONKEYUP);
super.onRender(target, index);
if (GXT.isHighContrastMode) {
getElement().setInnerHTML(" ");
}
if (GXT.isFocusManagerEnabled()) {
el().setTabIndex(0);
Accessibility.setRole(getElement(), Accessibility.ROLE_BUTTON);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy