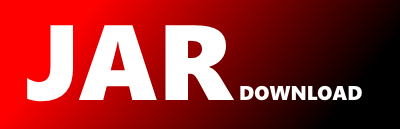
com.extjs.gxt.ui.client.widget.Dialog Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of gxt Show documentation
Show all versions of gxt Show documentation
Rich Internet Application Framework for GWT
/*
* Sencha GXT 2.3.1a - Sencha for GWT
* Copyright(c) 2007-2013, Sencha, Inc.
* [email protected]
*
* http://www.sencha.com/products/gxt/license/
*/
package com.extjs.gxt.ui.client.widget;
import com.extjs.gxt.ui.client.GXT;
import com.extjs.gxt.ui.client.event.ButtonEvent;
import com.extjs.gxt.ui.client.event.SelectionListener;
import com.extjs.gxt.ui.client.widget.button.Button;
/**
* A Window
with specialized support for buttons. Defaults to a
* dialog with an 'ok' button.
*
* Code snippet:
*
*
* Dialog d = new Dialog();
* d.setHeading("Exit Warning!");
* d.addText("Do you wish to save before exiting?");
* d.setBodyStyle("fontWeight:bold;padding:13px;");
* d.setSize(300, 100);
* d.setHideOnButtonClick(true);
* d.setButtons(Dialog.YESNOCANCEL);
* d.show();
*
*
*
* The internal buttons can be retrieved from the button bar using their
* respective ids ('ok', 'cancel', 'yes', 'no', 'cancel') or by index. The
* method {@link #getButtonBar()} creates the buttons, so any call before the
* dialog is rendered will cause the buttons to be created based on the
* {@link #setButtons(String)} value.
*
*
* - Inherited Events:
* - Window Activate
* - Window Deactivate
* - Window Minimize
* - Window Maximize
* - Window Restore
* - Window Resize
* - ContentPanel BeforeExpand
* - ContentPanel Expand
* - ContentPanel BeforeCollapse
* - ContentPanel Collapse
* - ContentPanel BeforeClose
* - ContentPanel Close
* - LayoutContainer AfterLayout
* - ScrollContainer Scroll
* - Container BeforeAdd
* - Container Add
* - Container BeforeRemove
* - Container Remove
* - BoxComponent Move
* - BoxComponent Resize
* - Component Enable
* - Component Disable
* - Component BeforeHide
* - Component Hide
* - Component BeforeShow
* - Component Show
* - Component Attach
* - Component Detach
* - Component BeforeRender
* - Component Render
* - Component BrowserEvent
* - Component BeforeStateRestore
* - Component StateRestore
* - Component BeforeStateSave
* - Component SaveState
*
*/
public class Dialog extends Window {
/**
* Button constant that displays a single OK button.
*/
public static final String OK = "ok";
/**
* Button constant that displays a single CANCEL button.
*/
public static final String CANCEL = "cancel";
/**
* Button constant that displays a single CLOSE button.
*/
public static final String CLOSE = "close";
/**
* Button constant that displays a OK and CANCEL button.
*/
public static final String OKCANCEL = "okcancel";
/**
* Button constant that displays a YES and NO button.
*/
public static final String YESNO = "yesno";
/**
* Button constant for the itemId of a NO button.
*/
public static final String NO = "no";
/**
* Button constant for the itemId of a YES button.
*/
public static final String YES = "yes";
/**
* Button constant that displays a YES, NO, and CANCEL button.
*/
public static final String YESNOCANCEL = "yesnocancel";
/**
* The OK button text (defaults to 'OK');
*/
public String okText = GXT.MESSAGES.messageBox_ok();
/**
* The Close button text (defaults to 'Close').
*/
public String closeText = GXT.MESSAGES.messageBox_close();
/**
* The Cancel button text (defaults to 'Cancel').
*/
public String cancelText = GXT.MESSAGES.messageBox_cancel();
/**
* The Yes button text (defaults to 'Yes').
*/
public String yesText = GXT.MESSAGES.messageBox_yes();
/**
* The No button text (defaults to 'No').
*/
public String noText = GXT.MESSAGES.messageBox_no();
private boolean hideOnButtonClick = false;
private String buttons;
private SelectionListener l = new SelectionListener() {
@Override
public void componentSelected(ButtonEvent ce) {
onButtonPressed(ce.getButton());
}
};
public Dialog() {
setButtons(OK);
}
/**
* Returns the button's.
*
* @return the buttons the buttons
*/
public String getButtons() {
return buttons;
}
public Button getButtonById(String string) {
return (Button) fbar.getItemByItemId(string);
}
/**
* Returns true if the dialog will be hidden on any button click.
*
* @return the hide on button click state
*/
public boolean isHideOnButtonClick() {
return hideOnButtonClick;
}
/**
* Sets the buttons to display (defaults to OK). Must be one of:
*
*
* Dialog.OK
* Dialog.CANCEL
* Dialog.OKCANCEL
* Dialog.YESNO
* Dialog.YESNOCANCEL
*
*/
public void setButtons(String buttons) {
this.buttons = buttons;
createButtons();
}
/**
* True to hide the dialog on any button click.
*
* @param hideOnButtonClick true to hide
*/
public void setHideOnButtonClick(boolean hideOnButtonClick) {
this.hideOnButtonClick = hideOnButtonClick;
}
/**
* Creates the buttons based on button creation constant
*/
protected void createButtons() {
getButtonBar().removeAll();
setFocusWidget(null);
if (buttons.indexOf(OK) != -1) {
Button okBtn = new Button(okText);
okBtn.setItemId(OK);
okBtn.addSelectionListener(l);
setFocusWidget(okBtn);
addButton(okBtn);
}
if (buttons.indexOf(YES) != -1) {
Button yesBtn = new Button(yesText);
yesBtn.setItemId(YES);
yesBtn.addSelectionListener(l);
setFocusWidget(yesBtn);
addButton(yesBtn);
}
if (buttons.indexOf(NO) != -1) {
Button noBtn = new Button(noText);
noBtn.setItemId(NO);
noBtn.addSelectionListener(l);
setFocusWidget(noBtn);
addButton(noBtn);
}
if (buttons.indexOf(CANCEL) != -1) {
Button cancelBtn = new Button(cancelText);
cancelBtn.setItemId(CANCEL);
cancelBtn.addSelectionListener(l);
setFocusWidget(cancelBtn);
addButton(cancelBtn);
}
if (buttons.indexOf(CLOSE) != -1) {
Button closeBtn = new Button(closeText);
closeBtn.setItemId(CLOSE);
closeBtn.addSelectionListener(l);
addButton(closeBtn);
}
}
/**
* Called after a button in the button bar is selected. If
* {@link #setHideOnButtonClick(boolean)} is true, hides the dialog
* when any button is pressed.
*
* @param button the button
*/
protected void onButtonPressed(Button button) {
if (button == getButtonBar().getItemByItemId(CLOSE) || hideOnButtonClick) {
hide(button);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy