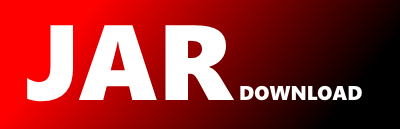
com.facebook.api.schema.DailyMetrics Maven / Gradle / Ivy
//
// This file was generated by the JavaTM Architecture for XML Binding(JAXB) Reference Implementation, vJAXB 2.1.3 in JDK 1.6
// See http://java.sun.com/xml/jaxb
// Any modifications to this file will be lost upon recompilation of the source schema.
// Generated on: 2008.07.20 at 05:29:33 PM PDT
//
package com.facebook.api.schema;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlType;
/**
*
* Java class for daily_metrics complex type.
*
*
* The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType name="daily_metrics">
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <all>
* <element name="date" type="{http://api.facebook.com/1.0/}time" minOccurs="0"/>
* <element name="daily_active_users" type="{http://www.w3.org/2001/XMLSchema}int" minOccurs="0"/>
* <element name="unique_adds" type="{http://www.w3.org/2001/XMLSchema}int" minOccurs="0"/>
* <element name="unique_removes" type="{http://www.w3.org/2001/XMLSchema}int" minOccurs="0"/>
* <element name="unique_blocks" type="{http://www.w3.org/2001/XMLSchema}int" minOccurs="0"/>
* <element name="unique_unblocks" type="{http://www.w3.org/2001/XMLSchema}int" minOccurs="0"/>
* <element name="api_calls" type="{http://www.w3.org/2001/XMLSchema}int" minOccurs="0"/>
* <element name="unique_api_calls" type="{http://www.w3.org/2001/XMLSchema}int" minOccurs="0"/>
* <element name="canvas_page_views" type="{http://www.w3.org/2001/XMLSchema}int" minOccurs="0"/>
* <element name="unique_canvas_page_views" type="{http://www.w3.org/2001/XMLSchema}int" minOccurs="0"/>
* <element name="canvas_http_request_time_avg" type="{http://www.w3.org/2001/XMLSchema}int" minOccurs="0"/>
* <element name="canvas_fbml_render_time_avg" type="{http://www.w3.org/2001/XMLSchema}int" minOccurs="0"/>
* </all>
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "daily_metrics", propOrder = {
})
public class DailyMetrics {
protected Integer date;
@XmlElement(name = "daily_active_users")
protected Integer dailyActiveUsers;
@XmlElement(name = "unique_adds")
protected Integer uniqueAdds;
@XmlElement(name = "unique_removes")
protected Integer uniqueRemoves;
@XmlElement(name = "unique_blocks")
protected Integer uniqueBlocks;
@XmlElement(name = "unique_unblocks")
protected Integer uniqueUnblocks;
@XmlElement(name = "api_calls")
protected Integer apiCalls;
@XmlElement(name = "unique_api_calls")
protected Integer uniqueApiCalls;
@XmlElement(name = "canvas_page_views")
protected Integer canvasPageViews;
@XmlElement(name = "unique_canvas_page_views")
protected Integer uniqueCanvasPageViews;
@XmlElement(name = "canvas_http_request_time_avg")
protected Integer canvasHttpRequestTimeAvg;
@XmlElement(name = "canvas_fbml_render_time_avg")
protected Integer canvasFbmlRenderTimeAvg;
/**
* Gets the value of the date property.
*
* @return possible object is {@link Integer }
*
*/
public Integer getDate() {
return date;
}
/**
* Sets the value of the date property.
*
* @param value
* allowed object is {@link Integer }
*
*/
public void setDate( Integer value ) {
this.date = value;
}
/**
* Gets the value of the dailyActiveUsers property.
*
* @return possible object is {@link Integer }
*
*/
public Integer getDailyActiveUsers() {
return dailyActiveUsers;
}
/**
* Sets the value of the dailyActiveUsers property.
*
* @param value
* allowed object is {@link Integer }
*
*/
public void setDailyActiveUsers( Integer value ) {
this.dailyActiveUsers = value;
}
/**
* Gets the value of the uniqueAdds property.
*
* @return possible object is {@link Integer }
*
*/
public Integer getUniqueAdds() {
return uniqueAdds;
}
/**
* Sets the value of the uniqueAdds property.
*
* @param value
* allowed object is {@link Integer }
*
*/
public void setUniqueAdds( Integer value ) {
this.uniqueAdds = value;
}
/**
* Gets the value of the uniqueRemoves property.
*
* @return possible object is {@link Integer }
*
*/
public Integer getUniqueRemoves() {
return uniqueRemoves;
}
/**
* Sets the value of the uniqueRemoves property.
*
* @param value
* allowed object is {@link Integer }
*
*/
public void setUniqueRemoves( Integer value ) {
this.uniqueRemoves = value;
}
/**
* Gets the value of the uniqueBlocks property.
*
* @return possible object is {@link Integer }
*
*/
public Integer getUniqueBlocks() {
return uniqueBlocks;
}
/**
* Sets the value of the uniqueBlocks property.
*
* @param value
* allowed object is {@link Integer }
*
*/
public void setUniqueBlocks( Integer value ) {
this.uniqueBlocks = value;
}
/**
* Gets the value of the uniqueUnblocks property.
*
* @return possible object is {@link Integer }
*
*/
public Integer getUniqueUnblocks() {
return uniqueUnblocks;
}
/**
* Sets the value of the uniqueUnblocks property.
*
* @param value
* allowed object is {@link Integer }
*
*/
public void setUniqueUnblocks( Integer value ) {
this.uniqueUnblocks = value;
}
/**
* Gets the value of the apiCalls property.
*
* @return possible object is {@link Integer }
*
*/
public Integer getApiCalls() {
return apiCalls;
}
/**
* Sets the value of the apiCalls property.
*
* @param value
* allowed object is {@link Integer }
*
*/
public void setApiCalls( Integer value ) {
this.apiCalls = value;
}
/**
* Gets the value of the uniqueApiCalls property.
*
* @return possible object is {@link Integer }
*
*/
public Integer getUniqueApiCalls() {
return uniqueApiCalls;
}
/**
* Sets the value of the uniqueApiCalls property.
*
* @param value
* allowed object is {@link Integer }
*
*/
public void setUniqueApiCalls( Integer value ) {
this.uniqueApiCalls = value;
}
/**
* Gets the value of the canvasPageViews property.
*
* @return possible object is {@link Integer }
*
*/
public Integer getCanvasPageViews() {
return canvasPageViews;
}
/**
* Sets the value of the canvasPageViews property.
*
* @param value
* allowed object is {@link Integer }
*
*/
public void setCanvasPageViews( Integer value ) {
this.canvasPageViews = value;
}
/**
* Gets the value of the uniqueCanvasPageViews property.
*
* @return possible object is {@link Integer }
*
*/
public Integer getUniqueCanvasPageViews() {
return uniqueCanvasPageViews;
}
/**
* Sets the value of the uniqueCanvasPageViews property.
*
* @param value
* allowed object is {@link Integer }
*
*/
public void setUniqueCanvasPageViews( Integer value ) {
this.uniqueCanvasPageViews = value;
}
/**
* Gets the value of the canvasHttpRequestTimeAvg property.
*
* @return possible object is {@link Integer }
*
*/
public Integer getCanvasHttpRequestTimeAvg() {
return canvasHttpRequestTimeAvg;
}
/**
* Sets the value of the canvasHttpRequestTimeAvg property.
*
* @param value
* allowed object is {@link Integer }
*
*/
public void setCanvasHttpRequestTimeAvg( Integer value ) {
this.canvasHttpRequestTimeAvg = value;
}
/**
* Gets the value of the canvasFbmlRenderTimeAvg property.
*
* @return possible object is {@link Integer }
*
*/
public Integer getCanvasFbmlRenderTimeAvg() {
return canvasFbmlRenderTimeAvg;
}
/**
* Sets the value of the canvasFbmlRenderTimeAvg property.
*
* @param value
* allowed object is {@link Integer }
*
*/
public void setCanvasFbmlRenderTimeAvg( Integer value ) {
this.canvasFbmlRenderTimeAvg = value;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy