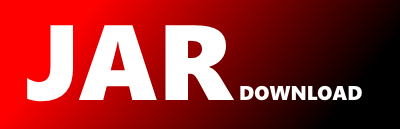
com.facebook.drift.codec.internal.ProtocolReader Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of drift-codec Show documentation
Show all versions of drift-codec Show documentation
Annotation based encoder and decoder for Thrift
/*
* Copyright (C) 2012 Facebook, Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.facebook.drift.codec.internal;
import com.facebook.drift.TException;
import com.facebook.drift.codec.ThriftCodec;
import com.facebook.drift.protocol.TField;
import com.facebook.drift.protocol.TList;
import com.facebook.drift.protocol.TMap;
import com.facebook.drift.protocol.TProtocolReader;
import com.facebook.drift.protocol.TProtocolUtil;
import com.facebook.drift.protocol.TSet;
import com.facebook.drift.protocol.TType;
import javax.annotation.concurrent.NotThreadSafe;
import java.nio.ByteBuffer;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.HashSet;
import java.util.List;
import java.util.Map;
import java.util.NoSuchElementException;
import java.util.Set;
import static com.google.common.base.MoreObjects.toStringHelper;
import static com.google.common.base.Preconditions.checkState;
@NotThreadSafe
public class ProtocolReader
{
private final TProtocolReader protocol;
private TField currentField;
public ProtocolReader(TProtocolReader protocol)
{
this.protocol = protocol;
}
public void readStructBegin()
throws TException
{
protocol.readStructBegin();
currentField = null;
}
public void readStructEnd()
throws TException
{
if (currentField == null || currentField.getId() != TType.STOP) {
throw new IllegalStateException("Some fields have not been consumed");
}
currentField = null;
protocol.readStructEnd();
}
public boolean nextField()
throws TException
{
// if the current field is a stop record, the caller must call readStructEnd.
if (currentField != null && currentField.getId() == TType.STOP) {
throw new NoSuchElementException();
}
checkState(currentField == null, "Current field was not read");
// advance to the next field
currentField = protocol.readFieldBegin();
return currentField.getType() != TType.STOP;
}
public short getFieldId()
{
checkState(currentField != null, "No current field");
return currentField.getId();
}
public byte getFieldType()
{
checkState(currentField != null, "No current field");
return currentField.getType();
}
public void skipFieldData()
throws TException
{
TProtocolUtil.skip(protocol, currentField.getType());
protocol.readFieldEnd();
currentField = null;
}
public Object readField(ThriftCodec> codec)
throws Exception
{
if (!checkReadState(codec.getType().getProtocolType().getType())) {
return null;
}
currentField = null;
Object fieldValue = codec.read(protocol);
protocol.readFieldEnd();
return fieldValue;
}
public ByteBuffer readBinaryField()
throws TException
{
if (!checkReadState(TType.STRING)) {
return null;
}
currentField = null;
ByteBuffer fieldValue = protocol.readBinary();
protocol.readFieldEnd();
return fieldValue;
}
public int readBinaryField(byte[] buf, int offset)
throws TException
{
if (!checkReadState(TType.STRING)) {
return 0;
}
currentField = null;
int length = protocol.readBinary(buf, offset);
protocol.readFieldEnd();
return length;
}
public boolean readBoolField()
throws TException
{
if (!checkReadState(TType.BOOL)) {
return false;
}
currentField = null;
boolean fieldValue = protocol.readBool();
protocol.readFieldEnd();
return fieldValue;
}
public byte readByteField()
throws TException
{
if (!checkReadState(TType.BYTE)) {
return 0;
}
currentField = null;
byte fieldValue = protocol.readByte();
protocol.readFieldEnd();
return fieldValue;
}
public double readDoubleField()
throws TException
{
if (!checkReadState(TType.DOUBLE)) {
return 0;
}
currentField = null;
double fieldValue = protocol.readDouble();
protocol.readFieldEnd();
return fieldValue;
}
public short readI16Field()
throws TException
{
if (!checkReadState(TType.I16)) {
return 0;
}
currentField = null;
short fieldValue = protocol.readI16();
protocol.readFieldEnd();
return fieldValue;
}
public int readI32Field()
throws TException
{
if (!checkReadState(TType.I32)) {
return 0;
}
currentField = null;
int fieldValue = protocol.readI32();
protocol.readFieldEnd();
return fieldValue;
}
public long readI64Field()
throws TException
{
if (!checkReadState(TType.I64)) {
return 0;
}
currentField = null;
long fieldValue = protocol.readI64();
protocol.readFieldEnd();
return fieldValue;
}
public String readStringField()
throws TException
{
if (!checkReadState(TType.STRING)) {
return null;
}
currentField = null;
String fieldValue = protocol.readString();
protocol.readFieldEnd();
return fieldValue;
}
public T readStructField(ThriftCodec codec)
throws Exception
{
if (!checkReadState(TType.STRUCT)) {
return null;
}
currentField = null;
T fieldValue = codec.read(protocol);
protocol.readFieldEnd();
return fieldValue;
}
public boolean[] readBoolArrayField()
throws TException
{
if (!checkReadState(TType.LIST)) {
return null;
}
currentField = null;
boolean[] fieldValue = readBoolArray();
protocol.readFieldEnd();
return fieldValue;
}
public short[] readI16ArrayField()
throws TException
{
if (!checkReadState(TType.LIST)) {
return null;
}
currentField = null;
short[] fieldValue = readI16Array();
protocol.readFieldEnd();
return fieldValue;
}
public int[] readI32ArrayField()
throws TException
{
if (!checkReadState(TType.LIST)) {
return null;
}
currentField = null;
int[] fieldValue = readI32Array();
protocol.readFieldEnd();
return fieldValue;
}
public long[] readI64ArrayField()
throws TException
{
if (!checkReadState(TType.LIST)) {
return null;
}
currentField = null;
long[] fieldValue = readI64Array();
protocol.readFieldEnd();
return fieldValue;
}
public double[] readDoubleArrayField()
throws TException
{
if (!checkReadState(TType.LIST)) {
return null;
}
currentField = null;
double[] fieldValue = readDoubleArray();
protocol.readFieldEnd();
return fieldValue;
}
public Set readSetField(ThriftCodec> setCodec)
throws Exception
{
if (!checkReadState(TType.SET)) {
return null;
}
currentField = null;
Set fieldValue = setCodec.read(protocol);
protocol.readFieldEnd();
return fieldValue;
}
public List readListField(ThriftCodec> listCodec)
throws Exception
{
if (!checkReadState(TType.LIST)) {
return null;
}
currentField = null;
List read = listCodec.read(protocol);
protocol.readFieldEnd();
return read;
}
public Map readMapField(ThriftCodec
© 2015 - 2025 Weber Informatics LLC | Privacy Policy