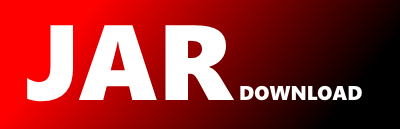
com.facebook.presto.druid.DruidConnectorPlanOptimizer Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.facebook.presto.druid;
import com.facebook.presto.expressions.LogicalRowExpressions;
import com.facebook.presto.spi.ColumnHandle;
import com.facebook.presto.spi.ConnectorPlanOptimizer;
import com.facebook.presto.spi.ConnectorSession;
import com.facebook.presto.spi.ConnectorTableHandle;
import com.facebook.presto.spi.PrestoException;
import com.facebook.presto.spi.TableHandle;
import com.facebook.presto.spi.VariableAllocator;
import com.facebook.presto.spi.function.FunctionMetadataManager;
import com.facebook.presto.spi.function.StandardFunctionResolution;
import com.facebook.presto.spi.plan.FilterNode;
import com.facebook.presto.spi.plan.PlanNode;
import com.facebook.presto.spi.plan.PlanNodeIdAllocator;
import com.facebook.presto.spi.plan.PlanVisitor;
import com.facebook.presto.spi.plan.TableScanNode;
import com.facebook.presto.spi.relation.DeterminismEvaluator;
import com.facebook.presto.spi.relation.RowExpression;
import com.facebook.presto.spi.relation.VariableReferenceExpression;
import com.facebook.presto.spi.type.TypeManager;
import com.google.common.collect.ImmutableList;
import javax.inject.Inject;
import java.util.ArrayList;
import java.util.IdentityHashMap;
import java.util.List;
import java.util.Map;
import java.util.Optional;
import static com.facebook.presto.druid.DruidErrorCode.DRUID_QUERY_GENERATOR_FAILURE;
import static com.facebook.presto.spi.StandardErrorCode.GENERIC_INTERNAL_ERROR;
import static com.google.common.collect.ImmutableList.toImmutableList;
import static com.google.common.collect.ImmutableMap.toImmutableMap;
import static java.util.Objects.requireNonNull;
public class DruidConnectorPlanOptimizer
implements ConnectorPlanOptimizer
{
private final DruidQueryGenerator druidQueryGenerator;
private final TypeManager typeManager;
private final FunctionMetadataManager functionMetadataManager;
private final LogicalRowExpressions logicalRowExpressions;
private final StandardFunctionResolution standardFunctionResolution;
@Inject
public DruidConnectorPlanOptimizer(
DruidQueryGenerator druidQueryGenerator,
TypeManager typeManager,
DeterminismEvaluator determinismEvaluator,
FunctionMetadataManager functionMetadataManager,
StandardFunctionResolution standardFunctionResolution)
{
this.druidQueryGenerator = requireNonNull(druidQueryGenerator, "pinot query generator is null");
this.typeManager = requireNonNull(typeManager, "type manager is null");
this.functionMetadataManager = requireNonNull(functionMetadataManager, "function manager is null");
this.standardFunctionResolution = requireNonNull(standardFunctionResolution, "standard function resolution is null");
this.logicalRowExpressions = new LogicalRowExpressions(
determinismEvaluator,
standardFunctionResolution,
functionMetadataManager);
}
@Override
public PlanNode optimize(PlanNode maxSubplan,
ConnectorSession session,
VariableAllocator variableAllocator,
PlanNodeIdAllocator idAllocator)
{
Map scanNodes = maxSubplan.accept(new TableFindingVisitor(), null);
TableScanNode druidTableScanNode = getOnlyDruidTable(scanNodes).orElseThrow(() -> new PrestoException(
GENERIC_INTERNAL_ERROR,
"Expected to find druid table handle for the scan node"));
return maxSubplan.accept(new Visitor(druidTableScanNode, session, idAllocator), null);
}
private static Optional getDruidTableHandle(TableScanNode tableScanNode)
{
TableHandle table = tableScanNode.getTable();
if (table != null) {
ConnectorTableHandle connectorHandle = table.getConnectorHandle();
if (connectorHandle instanceof DruidTableHandle) {
return Optional.of((DruidTableHandle) connectorHandle);
}
}
return Optional.empty();
}
private static Optional getOnlyDruidTable(Map scanNodes)
{
if (scanNodes.size() == 1) {
TableScanNode tableScanNode = scanNodes.keySet().iterator().next();
if (getDruidTableHandle(tableScanNode).isPresent()) {
return Optional.of(tableScanNode);
}
}
return Optional.empty();
}
private static PlanNode replaceChildren(PlanNode node, List children)
{
for (int i = 0; i < node.getSources().size(); i++) {
if (children.get(i) != node.getSources().get(i)) {
return node.replaceChildren(children);
}
}
return node;
}
private static class TableFindingVisitor
extends PlanVisitor
© 2015 - 2025 Weber Informatics LLC | Privacy Policy