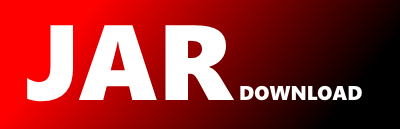
com.facebook.presto.hive.metastore.file.TableMetadata Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.facebook.presto.hive.metastore.file;
import com.facebook.presto.hive.HiveBucketProperty;
import com.facebook.presto.hive.HiveStorageFormat;
import com.facebook.presto.hive.metastore.Column;
import com.facebook.presto.hive.metastore.HiveColumnStatistics;
import com.facebook.presto.hive.metastore.PrestoTableType;
import com.facebook.presto.hive.metastore.Storage;
import com.facebook.presto.hive.metastore.StorageFormat;
import com.facebook.presto.hive.metastore.Table;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.databind.annotation.JsonDeserialize;
import com.google.common.collect.ImmutableList;
import com.google.common.collect.ImmutableMap;
import java.util.List;
import java.util.Map;
import java.util.Optional;
import static com.facebook.presto.hive.HiveStorageFormat.getHiveStorageFormat;
import static com.facebook.presto.hive.metastore.PrestoTableType.EXTERNAL_TABLE;
import static com.facebook.presto.hive.metastore.StorageFormat.VIEW_STORAGE_FORMAT;
import static com.google.common.base.MoreObjects.firstNonNull;
import static com.google.common.base.Preconditions.checkArgument;
import static java.util.Objects.requireNonNull;
public class TableMetadata
{
private final String owner;
private final PrestoTableType tableType;
private final List dataColumns;
private final List partitionColumns;
private final Map parameters;
private final StorageFormat storageFormat;
private final Optional bucketProperty;
private final Map storageParameters;
private final Map serdeParameters;
private final Optional externalLocation;
private final Optional viewOriginalText;
private final Optional viewExpandedText;
private final Map columnStatistics;
@JsonCreator
public TableMetadata(
@JsonProperty("owner") String owner,
@JsonProperty("tableType") PrestoTableType tableType,
@JsonProperty("dataColumns") List dataColumns,
@JsonProperty("partitionColumns") List partitionColumns,
@JsonProperty("parameters") Map parameters,
@JsonDeserialize(using = StorageFormatCompatDeserializer.class)
@JsonProperty("storageFormat") StorageFormat storageFormat,
@JsonProperty("bucketProperty") Optional bucketProperty,
@JsonProperty("storageParameters") Map storageParameters,
@JsonProperty("serdeParameters") Map serdeParameters,
@JsonProperty("externalLocation") Optional externalLocation,
@JsonProperty("viewOriginalText") Optional viewOriginalText,
@JsonProperty("viewExpandedText") Optional viewExpandedText,
@JsonProperty("columnStatistics") Map columnStatistics)
{
this.owner = requireNonNull(owner, "owner is null");
this.tableType = requireNonNull(tableType, "tableType is null");
this.dataColumns = ImmutableList.copyOf(requireNonNull(dataColumns, "dataColumns is null"));
this.partitionColumns = ImmutableList.copyOf(requireNonNull(partitionColumns, "partitionColumns is null"));
this.parameters = ImmutableMap.copyOf(requireNonNull(parameters, "parameters is null"));
this.storageParameters = ImmutableMap.copyOf(firstNonNull(storageParameters, ImmutableMap.of()));
this.storageFormat = storageFormat == null ? VIEW_STORAGE_FORMAT : storageFormat;
this.bucketProperty = requireNonNull(bucketProperty, "bucketProperty is null");
this.serdeParameters = requireNonNull(serdeParameters, "serdeParameters is null");
this.externalLocation = requireNonNull(externalLocation, "externalLocation is null");
if (tableType.equals(EXTERNAL_TABLE)) {
checkArgument(externalLocation.isPresent(), "External location is required for external tables");
}
else {
checkArgument(!externalLocation.isPresent(), "External location is only allowed for external tables");
}
this.viewOriginalText = requireNonNull(viewOriginalText, "viewOriginalText is null");
this.viewExpandedText = requireNonNull(viewExpandedText, "viewExpandedText is null");
this.columnStatistics = ImmutableMap.copyOf(requireNonNull(columnStatistics, "columnStatistics is null"));
checkArgument(partitionColumns.isEmpty() || columnStatistics.isEmpty(), "column statistics cannot be set for partitioned table");
}
@Deprecated
public TableMetadata(
String owner,
PrestoTableType tableType,
List dataColumns,
List partitionColumns,
Map parameters,
Optional storageFormat,
Optional bucketProperty,
Map storageParameters,
Map serdeParameters,
Optional externalLocation,
Optional viewOriginalText,
Optional viewExpandedText,
Map columnStatistics)
{
this(
owner,
tableType,
dataColumns,
partitionColumns,
parameters,
storageFormat.map(StorageFormat::fromHiveStorageFormat).orElse(VIEW_STORAGE_FORMAT),
bucketProperty,
storageParameters,
serdeParameters,
externalLocation,
viewOriginalText,
viewExpandedText,
columnStatistics);
}
public TableMetadata(Table table)
{
this(table, ImmutableMap.of());
}
public TableMetadata(Table table, Map columnStatistics)
{
owner = table.getOwner();
tableType = table.getTableType();
dataColumns = table.getDataColumns();
partitionColumns = table.getPartitionColumns();
parameters = table.getParameters();
storageFormat = table.getStorage().getStorageFormat();
bucketProperty = table.getStorage().getBucketProperty();
storageParameters = table.getStorage().getParameters();
serdeParameters = table.getStorage().getSerdeParameters();
if (tableType.equals(EXTERNAL_TABLE)) {
externalLocation = Optional.of(table.getStorage().getLocation());
}
else {
externalLocation = Optional.empty();
}
viewOriginalText = table.getViewOriginalText();
viewExpandedText = table.getViewExpandedText();
this.columnStatistics = ImmutableMap.copyOf(requireNonNull(columnStatistics, "columnStatistics is null"));
}
@JsonProperty
public String getOwner()
{
return owner;
}
@JsonProperty
public PrestoTableType getTableType()
{
return tableType;
}
@JsonProperty
public List getDataColumns()
{
return dataColumns;
}
@JsonProperty
public List getPartitionColumns()
{
return partitionColumns;
}
public Optional getColumn(String name)
{
for (Column partitionColumn : partitionColumns) {
if (partitionColumn.getName().equals(name)) {
return Optional.of(partitionColumn);
}
}
for (Column dataColumn : dataColumns) {
if (dataColumn.getName().equals(name)) {
return Optional.of(dataColumn);
}
}
return Optional.empty();
}
@JsonProperty
public Map getParameters()
{
return parameters;
}
@Deprecated
@JsonIgnore
public Optional getStorageFormat()
{
return getHiveStorageFormat(storageFormat);
}
@JsonProperty("storageFormat")
public StorageFormat getTableStorageFormat()
{
return storageFormat;
}
@JsonProperty
public Optional getBucketProperty()
{
return bucketProperty;
}
@JsonProperty
public Map getStorageParameters()
{
return storageParameters;
}
@JsonProperty
public Map getSerdeParameters()
{
return serdeParameters;
}
@JsonProperty
public Optional getExternalLocation()
{
return externalLocation;
}
@JsonProperty
public Optional getViewOriginalText()
{
return viewOriginalText;
}
@JsonProperty
public Optional getViewExpandedText()
{
return viewExpandedText;
}
@JsonProperty
public Map getColumnStatistics()
{
return columnStatistics;
}
public TableMetadata withDataColumns(List dataColumns)
{
return new TableMetadata(
owner,
tableType,
dataColumns,
partitionColumns,
parameters,
storageFormat,
bucketProperty,
storageParameters,
serdeParameters,
externalLocation,
viewOriginalText,
viewExpandedText,
columnStatistics);
}
public TableMetadata withParameters(Map parameters)
{
return new TableMetadata(
owner,
tableType,
dataColumns,
partitionColumns,
parameters,
storageFormat,
bucketProperty,
storageParameters,
serdeParameters,
externalLocation,
viewOriginalText,
viewExpandedText,
columnStatistics);
}
public TableMetadata withColumnStatistics(Map columnStatistics)
{
return new TableMetadata(
owner,
tableType,
dataColumns,
partitionColumns,
parameters,
storageFormat,
bucketProperty,
storageParameters,
serdeParameters,
externalLocation,
viewOriginalText,
viewExpandedText,
columnStatistics);
}
public Table toTable(String databaseName, String tableName, String location)
{
return new Table(
databaseName,
tableName,
owner,
tableType,
Storage.builder()
.setLocation(externalLocation.orElse(location))
.setStorageFormat(storageFormat)
.setBucketProperty(bucketProperty)
.setParameters(storageParameters)
.setSerdeParameters(serdeParameters)
.build(),
dataColumns,
partitionColumns,
parameters,
viewOriginalText,
viewExpandedText);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy