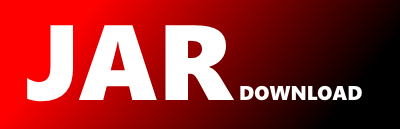
com.facebook.presto.jdbc.internal.common.block.VariableWidthBlock Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.facebook.presto.jdbc.internal.common.block;
import com.facebook.presto.jdbc.internal.io.airlift.slice.Slice;
import com.facebook.presto.jdbc.internal.io.airlift.slice.SliceOutput;
import com.facebook.presto.jdbc.internal.io.airlift.slice.Slices;
import com.facebook.presto.jdbc.internal.io.airlift.slice.UnsafeSlice;
import com.facebook.presto.jdbc.internal.jol.info.ClassLayout;
import com.facebook.presto.jdbc.internal.javax.annotation.Nullable;
import java.util.Arrays;
import java.util.Objects;
import java.util.Optional;
import java.util.OptionalInt;
import java.util.function.ObjLongConsumer;
import static com.facebook.presto.jdbc.internal.common.block.BlockUtil.appendNullToIsNullArray;
import static com.facebook.presto.jdbc.internal.common.block.BlockUtil.appendNullToOffsetsArray;
import static com.facebook.presto.jdbc.internal.common.block.BlockUtil.checkArrayRange;
import static com.facebook.presto.jdbc.internal.common.block.BlockUtil.checkValidPositions;
import static com.facebook.presto.jdbc.internal.common.block.BlockUtil.checkValidRegion;
import static com.facebook.presto.jdbc.internal.common.block.BlockUtil.compactArray;
import static com.facebook.presto.jdbc.internal.common.block.BlockUtil.compactOffsets;
import static com.facebook.presto.jdbc.internal.common.block.BlockUtil.compactSlice;
import static com.facebook.presto.jdbc.internal.common.block.BlockUtil.internalPositionInRange;
import static com.facebook.presto.jdbc.internal.io.airlift.slice.SizeOf.sizeOf;
import static com.facebook.presto.jdbc.internal.io.airlift.slice.Slices.EMPTY_SLICE;
import static java.lang.String.format;
public class VariableWidthBlock
extends AbstractVariableWidthBlock
{
private static final int INSTANCE_SIZE = ClassLayout.parseClass(VariableWidthBlock.class).instanceSize();
private final int arrayOffset;
private final int positionCount;
private final Slice slice;
private final int[] offsets;
@Nullable
private final boolean[] valueIsNull;
private final long retainedSizeInBytes;
private final long sizeInBytes;
public VariableWidthBlock(int positionCount, Slice slice, int[] offsets, Optional valueIsNull)
{
this(0, positionCount, slice, offsets, valueIsNull.orElse(null));
}
VariableWidthBlock(int arrayOffset, int positionCount, Slice slice, int[] offsets, boolean[] valueIsNull)
{
if (arrayOffset < 0) {
throw new IllegalArgumentException("arrayOffset is negative");
}
this.arrayOffset = arrayOffset;
if (positionCount < 0) {
throw new IllegalArgumentException("positionCount is negative");
}
this.positionCount = positionCount;
if (slice == null) {
throw new IllegalArgumentException("slice is null");
}
this.slice = slice;
if (offsets.length - arrayOffset < (positionCount + 1)) {
throw new IllegalArgumentException("offsets length is less than positionCount");
}
this.offsets = offsets;
if (valueIsNull != null && valueIsNull.length - arrayOffset < positionCount) {
throw new IllegalArgumentException("valueIsNull length is less than positionCount");
}
this.valueIsNull = valueIsNull;
sizeInBytes = offsets[arrayOffset + positionCount] - offsets[arrayOffset] + ((Integer.BYTES + Byte.BYTES) * (long) positionCount);
retainedSizeInBytes = INSTANCE_SIZE + slice.getRetainedSize() + sizeOf(valueIsNull) + sizeOf(offsets);
}
@Override
public final int getPositionOffset(int position)
{
return offsets[position + arrayOffset];
}
@Override
public int getSliceLength(int position)
{
checkReadablePosition(position);
return getSliceLengthUnchecked(position + arrayOffset);
}
@Override
public boolean mayHaveNull()
{
return valueIsNull != null;
}
@Override
protected boolean isEntryNull(int position)
{
return valueIsNull != null && valueIsNull[position + arrayOffset];
}
@Override
public int getPositionCount()
{
return positionCount;
}
@Override
public long getSizeInBytes()
{
return sizeInBytes;
}
@Override
public OptionalInt fixedSizeInBytesPerPosition()
{
return OptionalInt.empty(); // size is variable based on the per element length
}
@Override
public long getRegionSizeInBytes(int position, int length)
{
return offsets[arrayOffset + position + length] - offsets[arrayOffset + position] + ((Integer.BYTES + Byte.BYTES) * (long) length);
}
@Override
public long getPositionsSizeInBytes(boolean[] positions, int usedPositionCount)
{
checkValidPositions(positions, positionCount);
if (usedPositionCount == 0) {
return 0;
}
if (usedPositionCount == positionCount) {
return getSizeInBytes();
}
int sizeInBytes = 0;
for (int i = 0; i < positions.length; ++i) {
if (positions[i]) {
sizeInBytes += (offsets[arrayOffset + i + 1] - offsets[arrayOffset + i]);
}
}
return sizeInBytes + ((Integer.BYTES + Byte.BYTES) * (long) usedPositionCount);
}
@Override
public long getRetainedSizeInBytes()
{
return retainedSizeInBytes;
}
@Override
public void retainedBytesForEachPart(ObjLongConsumer
© 2015 - 2024 Weber Informatics LLC | Privacy Policy