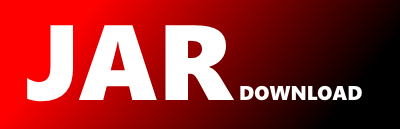
com.facebook.presto.verifier.framework.QueryConfiguration Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.facebook.presto.verifier.framework;
import com.google.common.collect.ImmutableMap;
import org.jdbi.v3.core.mapper.reflect.ColumnName;
import org.jdbi.v3.core.mapper.reflect.JdbiConstructor;
import java.util.HashMap;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import static com.facebook.presto.verifier.framework.QueryConfigurationOverrides.SessionPropertiesOverrideStrategy.OVERRIDE;
import static com.facebook.presto.verifier.framework.QueryConfigurationOverrides.SessionPropertiesOverrideStrategy.SUBSTITUTE;
import static com.google.common.base.MoreObjects.toStringHelper;
import static java.util.Objects.requireNonNull;
public class QueryConfiguration
{
private final String catalog;
private final String schema;
private final Optional username;
private final Optional password;
private final Map sessionProperties;
@JdbiConstructor
public QueryConfiguration(
@ColumnName("catalog") String catalog,
@ColumnName("schema") String schema,
@ColumnName("username") Optional username,
@ColumnName("password") Optional password,
@ColumnName("session_properties") Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy