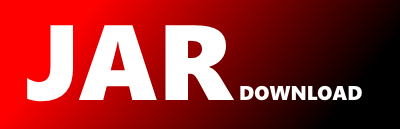
com.factset.sdk.FactSetGlobalPrices.models.Split Maven / Gradle / Ivy
/*
* FactSet Global Prices API
* The FactSet Global Prices API provides end of day market pricing content using cloud and microservices technology, encompassing both pricing as well as corporate actions and events data.
*
* The version of the OpenAPI document: 1.7.0
* Contact: [email protected]
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.factset.sdk.FactSetGlobalPrices.models;
import java.util.Objects;
import java.util.Arrays;
import java.util.Map;
import java.util.HashMap;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonTypeName;
import com.fasterxml.jackson.annotation.JsonValue;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.Serializable;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.factset.sdk.FactSetGlobalPrices.JSON;
/**
* Split
*/
@JsonPropertyOrder({
Split.JSON_PROPERTY_FSYM_ID,
Split.JSON_PROPERTY_EVENT_ID,
Split.JSON_PROPERTY_EVENT_TYPE_CODE,
Split.JSON_PROPERTY_EVENT_TYPE_DESC,
Split.JSON_PROPERTY_ANNOUNCEMENT_DATE,
Split.JSON_PROPERTY_RECORD_DATE,
Split.JSON_PROPERTY_PAY_DATE,
Split.JSON_PROPERTY_EFFECTIVE_DATE,
Split.JSON_PROPERTY_ADJ_FACTOR,
Split.JSON_PROPERTY_ADJ_FACTOR_COMBINED,
Split.JSON_PROPERTY_DIST_OLD_TERM,
Split.JSON_PROPERTY_DIST_NEW_TERM,
Split.JSON_PROPERTY_DIST_INST_FSYM_ID,
Split.JSON_PROPERTY_SHORT_DESC,
Split.JSON_PROPERTY_REQUEST_ID
})
@jakarta.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen")
public class Split implements Serializable {
private static final long serialVersionUID = 1L;
public static final String JSON_PROPERTY_FSYM_ID = "fsymId";
private String fsymId;
public static final String JSON_PROPERTY_EVENT_ID = "eventId";
private String eventId;
/**
* Character code that denotes the type of Event.
*/
public enum EventTypeCodeEnum {
FSP("FSP"),
RSP("RSP"),
SPL("SPL");
private String value;
EventTypeCodeEnum(String value) {
this.value = value;
}
@JsonValue
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
@JsonCreator
public static EventTypeCodeEnum fromValue(String value) {
for (EventTypeCodeEnum b : EventTypeCodeEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
throw new IllegalArgumentException("Unexpected value '" + value + "'");
}
}
public static final String JSON_PROPERTY_EVENT_TYPE_CODE = "eventTypeCode";
private EventTypeCodeEnum eventTypeCode;
public static final String JSON_PROPERTY_EVENT_TYPE_DESC = "eventTypeDesc";
private String eventTypeDesc;
public static final String JSON_PROPERTY_ANNOUNCEMENT_DATE = "announcementDate";
private String announcementDate;
public static final String JSON_PROPERTY_RECORD_DATE = "recordDate";
private String recordDate;
public static final String JSON_PROPERTY_PAY_DATE = "payDate";
private String payDate;
public static final String JSON_PROPERTY_EFFECTIVE_DATE = "effectiveDate";
private String effectiveDate;
public static final String JSON_PROPERTY_ADJ_FACTOR = "adjFactor";
private Double adjFactor;
public static final String JSON_PROPERTY_ADJ_FACTOR_COMBINED = "adjFactorCombined";
private Double adjFactorCombined;
public static final String JSON_PROPERTY_DIST_OLD_TERM = "distOldTerm";
private Double distOldTerm;
public static final String JSON_PROPERTY_DIST_NEW_TERM = "distNewTerm";
private Double distNewTerm;
public static final String JSON_PROPERTY_DIST_INST_FSYM_ID = "distInstFsymId";
private String distInstFsymId;
public static final String JSON_PROPERTY_SHORT_DESC = "shortDesc";
private String shortDesc;
public static final String JSON_PROPERTY_REQUEST_ID = "requestId";
private String requestId;
public Split() {
}
public Split fsymId(String fsymId) {
this.fsymId = fsymId;
return this;
}
/**
* Factset Regional Security Identifier. Six alpha-numeric characters, excluding vowels, with an -R suffix (XXXXXX-R). Identifies the security's best regional security data series per currency. For equities, all primary listings per region and currency are allocated a regional-level permanent identifier. The regional-level permanent identifier will be available once a SEDOL representing the region/currency has been allocated and the identifiers are on FactSet.
* @return fsymId
**/
@jakarta.annotation.Nullable
@ApiModelProperty(example = "SJY281-R", value = "Factset Regional Security Identifier. Six alpha-numeric characters, excluding vowels, with an -R suffix (XXXXXX-R). Identifies the security's best regional security data series per currency. For equities, all primary listings per region and currency are allocated a regional-level permanent identifier. The regional-level permanent identifier will be available once a SEDOL representing the region/currency has been allocated and the identifiers are on FactSet.")
@JsonProperty(JSON_PROPERTY_FSYM_ID)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getFsymId() {
return fsymId;
}
@JsonProperty(JSON_PROPERTY_FSYM_ID)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setFsymId(String fsymId) {
this.fsymId = fsymId;
}
public Split eventId(String eventId) {
this.eventId = eventId;
return this;
}
/**
* FactSet identifier that uniquely identifies the Event.
* @return eventId
**/
@jakarta.annotation.Nullable
@ApiModelProperty(example = "JTHPFN-A", value = "FactSet identifier that uniquely identifies the Event.")
@JsonProperty(JSON_PROPERTY_EVENT_ID)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getEventId() {
return eventId;
}
@JsonProperty(JSON_PROPERTY_EVENT_ID)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setEventId(String eventId) {
this.eventId = eventId;
}
public Split eventTypeCode(EventTypeCodeEnum eventTypeCode) {
this.eventTypeCode = eventTypeCode;
return this;
}
/**
* Character code that denotes the type of Event.
* @return eventTypeCode
**/
@jakarta.annotation.Nullable
@ApiModelProperty(value = "Character code that denotes the type of Event.")
@JsonProperty(JSON_PROPERTY_EVENT_TYPE_CODE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public EventTypeCodeEnum getEventTypeCode() {
return eventTypeCode;
}
@JsonProperty(JSON_PROPERTY_EVENT_TYPE_CODE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setEventTypeCode(EventTypeCodeEnum eventTypeCode) {
this.eventTypeCode = eventTypeCode;
}
public Split eventTypeDesc(String eventTypeDesc) {
this.eventTypeDesc = eventTypeDesc;
return this;
}
/**
* Corporate Actions Event type description.
* @return eventTypeDesc
**/
@jakarta.annotation.Nullable
@ApiModelProperty(example = "Forward Split", value = "Corporate Actions Event type description.")
@JsonProperty(JSON_PROPERTY_EVENT_TYPE_DESC)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getEventTypeDesc() {
return eventTypeDesc;
}
@JsonProperty(JSON_PROPERTY_EVENT_TYPE_DESC)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setEventTypeDesc(String eventTypeDesc) {
this.eventTypeDesc = eventTypeDesc;
}
public Split announcementDate(String announcementDate) {
this.announcementDate = announcementDate;
return this;
}
/**
* Date Event was announced in YYYY-MM-DD format.
* @return announcementDate
**/
@jakarta.annotation.Nullable
@ApiModelProperty(value = "Date Event was announced in YYYY-MM-DD format.")
@JsonProperty(JSON_PROPERTY_ANNOUNCEMENT_DATE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getAnnouncementDate() {
return announcementDate;
}
@JsonProperty(JSON_PROPERTY_ANNOUNCEMENT_DATE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setAnnouncementDate(String announcementDate) {
this.announcementDate = announcementDate;
}
public Split recordDate(String recordDate) {
this.recordDate = recordDate;
return this;
}
/**
* Date of Record for distribution in YYYY-MM-DD format.
* @return recordDate
**/
@jakarta.annotation.Nullable
@ApiModelProperty(value = "Date of Record for distribution in YYYY-MM-DD format.")
@JsonProperty(JSON_PROPERTY_RECORD_DATE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getRecordDate() {
return recordDate;
}
@JsonProperty(JSON_PROPERTY_RECORD_DATE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setRecordDate(String recordDate) {
this.recordDate = recordDate;
}
public Split payDate(String payDate) {
this.payDate = payDate;
return this;
}
/**
* Date of Payment for distribution in YYYY-MM-DD format.
* @return payDate
**/
@jakarta.annotation.Nullable
@ApiModelProperty(value = "Date of Payment for distribution in YYYY-MM-DD format.")
@JsonProperty(JSON_PROPERTY_PAY_DATE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getPayDate() {
return payDate;
}
@JsonProperty(JSON_PROPERTY_PAY_DATE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setPayDate(String payDate) {
this.payDate = payDate;
}
public Split effectiveDate(String effectiveDate) {
this.effectiveDate = effectiveDate;
return this;
}
/**
* Effective Date or Ex-Date of distribution in YYYY-MM-DD format.
* @return effectiveDate
**/
@jakarta.annotation.Nullable
@ApiModelProperty(value = "Effective Date or Ex-Date of distribution in YYYY-MM-DD format.")
@JsonProperty(JSON_PROPERTY_EFFECTIVE_DATE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getEffectiveDate() {
return effectiveDate;
}
@JsonProperty(JSON_PROPERTY_EFFECTIVE_DATE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setEffectiveDate(String effectiveDate) {
this.effectiveDate = effectiveDate;
}
public Split adjFactor(Double adjFactor) {
this.adjFactor = adjFactor;
return this;
}
/**
* Factor for adjusting price and shares. A 2-for-1 split returns .50, the number you would multiply the stock price by to adjust for the split.
* @return adjFactor
**/
@jakarta.annotation.Nullable
@ApiModelProperty(example = "0.997262", value = "Factor for adjusting price and shares. A 2-for-1 split returns .50, the number you would multiply the stock price by to adjust for the split. ")
@JsonProperty(JSON_PROPERTY_ADJ_FACTOR)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Double getAdjFactor() {
return adjFactor;
}
@JsonProperty(JSON_PROPERTY_ADJ_FACTOR)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setAdjFactor(Double adjFactor) {
this.adjFactor = adjFactor;
}
public Split adjFactorCombined(Double adjFactorCombined) {
this.adjFactorCombined = adjFactorCombined;
return this;
}
/**
* Combined adjustment factor for all distribution events on that day.
* @return adjFactorCombined
**/
@jakarta.annotation.Nullable
@ApiModelProperty(example = "0.997262", value = "Combined adjustment factor for all distribution events on that day.")
@JsonProperty(JSON_PROPERTY_ADJ_FACTOR_COMBINED)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Double getAdjFactorCombined() {
return adjFactorCombined;
}
@JsonProperty(JSON_PROPERTY_ADJ_FACTOR_COMBINED)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setAdjFactorCombined(Double adjFactorCombined) {
this.adjFactorCombined = adjFactorCombined;
}
public Split distOldTerm(Double distOldTerm) {
this.distOldTerm = distOldTerm;
return this;
}
/**
* Component of distribution ratio - Number of shares held.
* @return distOldTerm
**/
@jakarta.annotation.Nullable
@ApiModelProperty(example = "1", value = "Component of distribution ratio - Number of shares held.")
@JsonProperty(JSON_PROPERTY_DIST_OLD_TERM)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Double getDistOldTerm() {
return distOldTerm;
}
@JsonProperty(JSON_PROPERTY_DIST_OLD_TERM)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setDistOldTerm(Double distOldTerm) {
this.distOldTerm = distOldTerm;
}
public Split distNewTerm(Double distNewTerm) {
this.distNewTerm = distNewTerm;
return this;
}
/**
* Component of distribution ratio - Number of shares received.
* @return distNewTerm
**/
@jakarta.annotation.Nullable
@ApiModelProperty(example = "0.05", value = "Component of distribution ratio - Number of shares received.")
@JsonProperty(JSON_PROPERTY_DIST_NEW_TERM)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Double getDistNewTerm() {
return distNewTerm;
}
@JsonProperty(JSON_PROPERTY_DIST_NEW_TERM)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setDistNewTerm(Double distNewTerm) {
this.distNewTerm = distNewTerm;
}
public Split distInstFsymId(String distInstFsymId) {
this.distInstFsymId = distInstFsymId;
return this;
}
/**
* Parent Spin-Off for a company
* @return distInstFsymId
**/
@jakarta.annotation.Nullable
@ApiModelProperty(example = "RGW4H5-S", value = "Parent Spin-Off for a company")
@JsonProperty(JSON_PROPERTY_DIST_INST_FSYM_ID)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getDistInstFsymId() {
return distInstFsymId;
}
@JsonProperty(JSON_PROPERTY_DIST_INST_FSYM_ID)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setDistInstFsymId(String distInstFsymId) {
this.distInstFsymId = distInstFsymId;
}
public Split shortDesc(String shortDesc) {
this.shortDesc = shortDesc;
return this;
}
/**
* Textual description identifying the event.
* @return shortDesc
**/
@jakarta.annotation.Nullable
@ApiModelProperty(example = "Split (Mandatory): 3 for 1.", value = "Textual description identifying the event.")
@JsonProperty(JSON_PROPERTY_SHORT_DESC)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getShortDesc() {
return shortDesc;
}
@JsonProperty(JSON_PROPERTY_SHORT_DESC)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setShortDesc(String shortDesc) {
this.shortDesc = shortDesc;
}
public Split requestId(String requestId) {
this.requestId = requestId;
return this;
}
/**
* Identifier that was used for the request.
* @return requestId
**/
@jakarta.annotation.Nullable
@ApiModelProperty(example = "IBM-US", value = "Identifier that was used for the request.")
@JsonProperty(JSON_PROPERTY_REQUEST_ID)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getRequestId() {
return requestId;
}
@JsonProperty(JSON_PROPERTY_REQUEST_ID)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setRequestId(String requestId) {
this.requestId = requestId;
}
/**
* Return true if this Split object is equal to o.
*/
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
Split split = (Split) o;
return Objects.equals(this.fsymId, split.fsymId) &&
Objects.equals(this.eventId, split.eventId) &&
Objects.equals(this.eventTypeCode, split.eventTypeCode) &&
Objects.equals(this.eventTypeDesc, split.eventTypeDesc) &&
Objects.equals(this.announcementDate, split.announcementDate) &&
Objects.equals(this.recordDate, split.recordDate) &&
Objects.equals(this.payDate, split.payDate) &&
Objects.equals(this.effectiveDate, split.effectiveDate) &&
Objects.equals(this.adjFactor, split.adjFactor) &&
Objects.equals(this.adjFactorCombined, split.adjFactorCombined) &&
Objects.equals(this.distOldTerm, split.distOldTerm) &&
Objects.equals(this.distNewTerm, split.distNewTerm) &&
Objects.equals(this.distInstFsymId, split.distInstFsymId) &&
Objects.equals(this.shortDesc, split.shortDesc) &&
Objects.equals(this.requestId, split.requestId);
}
@Override
public int hashCode() {
return Objects.hash(fsymId, eventId, eventTypeCode, eventTypeDesc, announcementDate, recordDate, payDate, effectiveDate, adjFactor, adjFactorCombined, distOldTerm, distNewTerm, distInstFsymId, shortDesc, requestId);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class Split {\n");
sb.append(" fsymId: ").append(toIndentedString(fsymId)).append("\n");
sb.append(" eventId: ").append(toIndentedString(eventId)).append("\n");
sb.append(" eventTypeCode: ").append(toIndentedString(eventTypeCode)).append("\n");
sb.append(" eventTypeDesc: ").append(toIndentedString(eventTypeDesc)).append("\n");
sb.append(" announcementDate: ").append(toIndentedString(announcementDate)).append("\n");
sb.append(" recordDate: ").append(toIndentedString(recordDate)).append("\n");
sb.append(" payDate: ").append(toIndentedString(payDate)).append("\n");
sb.append(" effectiveDate: ").append(toIndentedString(effectiveDate)).append("\n");
sb.append(" adjFactor: ").append(toIndentedString(adjFactor)).append("\n");
sb.append(" adjFactorCombined: ").append(toIndentedString(adjFactorCombined)).append("\n");
sb.append(" distOldTerm: ").append(toIndentedString(distOldTerm)).append("\n");
sb.append(" distNewTerm: ").append(toIndentedString(distNewTerm)).append("\n");
sb.append(" distInstFsymId: ").append(toIndentedString(distInstFsymId)).append("\n");
sb.append(" shortDesc: ").append(toIndentedString(shortDesc)).append("\n");
sb.append(" requestId: ").append(toIndentedString(requestId)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy