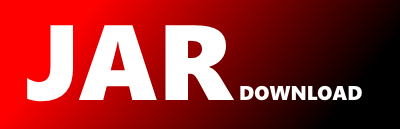
com.factset.sdk.IssueTracker.models.Comment Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of issuetracker Show documentation
Show all versions of issuetracker Show documentation
FactSet SDK for Java - issuetracker
/*
* Issue Tracker API
* This API is used to file issues
*
* The version of the OpenAPI document: 1.1.0
* Contact: [email protected]
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.factset.sdk.IssueTracker.models;
import java.util.Objects;
import java.util.Arrays;
import java.util.Map;
import java.util.HashMap;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonTypeName;
import com.fasterxml.jackson.annotation.JsonValue;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.time.OffsetDateTime;
import java.io.Serializable;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.factset.sdk.IssueTracker.JSON;
/**
* Comment
*/
@JsonPropertyOrder({
Comment.JSON_PROPERTY_ID,
Comment.JSON_PROPERTY_CONTENT,
Comment.JSON_PROPERTY_CREATED_AT,
Comment.JSON_PROPERTY_AUTHOR
})
@jakarta.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen")
public class Comment implements Serializable {
private static final long serialVersionUID = 1L;
public static final String JSON_PROPERTY_ID = "id";
private String id;
public static final String JSON_PROPERTY_CONTENT = "content";
private String content;
public static final String JSON_PROPERTY_CREATED_AT = "createdAt";
private OffsetDateTime createdAt;
public static final String JSON_PROPERTY_AUTHOR = "author";
private String author;
public Comment() {
}
public Comment id(String id) {
this.id = id;
return this;
}
/**
* generated comment id
* @return id
**/
@jakarta.annotation.Nullable
@ApiModelProperty(value = "generated comment id")
@JsonProperty(JSON_PROPERTY_ID)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getId() {
return id;
}
@JsonProperty(JSON_PROPERTY_ID)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setId(String id) {
this.id = id;
}
public Comment content(String content) {
this.content = content;
return this;
}
/**
* cotent of comment
* @return content
**/
@jakarta.annotation.Nullable
@ApiModelProperty(value = "cotent of comment")
@JsonProperty(JSON_PROPERTY_CONTENT)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getContent() {
return content;
}
@JsonProperty(JSON_PROPERTY_CONTENT)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setContent(String content) {
this.content = content;
}
public Comment createdAt(OffsetDateTime createdAt) {
this.createdAt = createdAt;
return this;
}
/**
* comment creation time
* @return createdAt
**/
@jakarta.annotation.Nullable
@ApiModelProperty(value = "comment creation time")
@JsonProperty(JSON_PROPERTY_CREATED_AT)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public OffsetDateTime getCreatedAt() {
return createdAt;
}
@JsonProperty(JSON_PROPERTY_CREATED_AT)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setCreatedAt(OffsetDateTime createdAt) {
this.createdAt = createdAt;
}
public Comment author(String author) {
this.author = author;
return this;
}
/**
* author of the comment.
* @return author
**/
@jakarta.annotation.Nullable
@ApiModelProperty(value = "author of the comment.")
@JsonProperty(JSON_PROPERTY_AUTHOR)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getAuthor() {
return author;
}
@JsonProperty(JSON_PROPERTY_AUTHOR)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setAuthor(String author) {
this.author = author;
}
/**
* Return true if this comment object is equal to o.
*/
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
Comment comment = (Comment) o;
return Objects.equals(this.id, comment.id) &&
Objects.equals(this.content, comment.content) &&
Objects.equals(this.createdAt, comment.createdAt) &&
Objects.equals(this.author, comment.author);
}
@Override
public int hashCode() {
return Objects.hash(id, content, createdAt, author);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class Comment {\n");
sb.append(" id: ").append(toIndentedString(id)).append("\n");
sb.append(" content: ").append(toIndentedString(content)).append("\n");
sb.append(" createdAt: ").append(toIndentedString(createdAt)).append("\n");
sb.append(" author: ").append(toIndentedString(author)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy