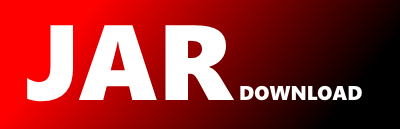
com.factset.sdk.SecurityModeling.models.SMCustomCashFlowFields Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of securitymodeling Show documentation
Show all versions of securitymodeling Show documentation
FactSet SDK for Java - securitymodeling
/*
* Security-Modeling API
* Allow clients to fetch Analytics through APIs.
*
* The version of the OpenAPI document: 3
* Contact: [email protected]
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.factset.sdk.SecurityModeling.models;
import java.util.Objects;
import java.util.Arrays;
import java.util.Map;
import java.util.HashMap;
import com.factset.sdk.SecurityModeling.models.SMFields;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonTypeName;
import com.fasterxml.jackson.annotation.JsonValue;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import org.openapitools.jackson.nullable.JsonNullable;
import com.fasterxml.jackson.annotation.JsonIgnore;
import org.openapitools.jackson.nullable.JsonNullable;
import java.util.NoSuchElementException;
import java.io.Serializable;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.factset.sdk.SecurityModeling.JSON;
/**
* SMCustomCashFlowFields
*/
@JsonPropertyOrder({
SMCustomCashFlowFields.JSON_PROPERTY_CASH_FLOW_AMOUNTS,
SMCustomCashFlowFields.JSON_PROPERTY_CASH_FLOW_DATES,
SMCustomCashFlowFields.JSON_PROPERTY_COUNTRY,
SMCustomCashFlowFields.JSON_PROPERTY_CURRENCY,
SMCustomCashFlowFields.JSON_PROPERTY_ISSUE_NAME,
SMCustomCashFlowFields.JSON_PROPERTY_PAR_AMT,
SMCustomCashFlowFields.JSON_PROPERTY_PARENT_NAME,
SMCustomCashFlowFields.JSON_PROPERTY_RATING_FITCH,
SMCustomCashFlowFields.JSON_PROPERTY_RATING_FITCH_DATES,
SMCustomCashFlowFields.JSON_PROPERTY_RATING_FITCH_VALUES,
SMCustomCashFlowFields.JSON_PROPERTY_RATING_MOODYS_DATES,
SMCustomCashFlowFields.JSON_PROPERTY_RATING_MOODYS_VALUES,
SMCustomCashFlowFields.JSON_PROPERTY_RATING_SP_DATES,
SMCustomCashFlowFields.JSON_PROPERTY_RATING_SP_VALUES,
SMCustomCashFlowFields.JSON_PROPERTY_SECONDARY_TO_VENDOR_FLAG,
SMCustomCashFlowFields.JSON_PROPERTY_SECTOR,
SMCustomCashFlowFields.JSON_PROPERTY_SECTOR_BARCLAY1,
SMCustomCashFlowFields.JSON_PROPERTY_SECTOR_BARCLAY2,
SMCustomCashFlowFields.JSON_PROPERTY_SECTOR_BARCLAY3,
SMCustomCashFlowFields.JSON_PROPERTY_SECTOR_BARCLAY4,
SMCustomCashFlowFields.JSON_PROPERTY_SECTOR_DEF,
SMCustomCashFlowFields.JSON_PROPERTY_SECTOR_INDUSTRY,
SMCustomCashFlowFields.JSON_PROPERTY_SECTOR_MAIN,
SMCustomCashFlowFields.JSON_PROPERTY_SECTOR_MERRILL1,
SMCustomCashFlowFields.JSON_PROPERTY_SECTOR_MERRILL2,
SMCustomCashFlowFields.JSON_PROPERTY_SECTOR_MERRILL3,
SMCustomCashFlowFields.JSON_PROPERTY_SECTOR_MERRILL4,
SMCustomCashFlowFields.JSON_PROPERTY_SECTOR_SUB_GROUP,
SMCustomCashFlowFields.JSON_PROPERTY_VENDOR_COVERAGE_DATE,
SMCustomCashFlowFields.JSON_PROPERTY_SECURITY_TYPE
})
@jakarta.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen")
public class SMCustomCashFlowFields implements Serializable {
private static final long serialVersionUID = 1L;
public static final String JSON_PROPERTY_CASH_FLOW_AMOUNTS = "cashFlowAmounts";
private JsonNullable> cashFlowAmounts = JsonNullable.>undefined();
public static final String JSON_PROPERTY_CASH_FLOW_DATES = "cashFlowDates";
private JsonNullable> cashFlowDates = JsonNullable.>undefined();
public static final String JSON_PROPERTY_COUNTRY = "country";
private JsonNullable country = JsonNullable.undefined();
public static final String JSON_PROPERTY_CURRENCY = "currency";
private JsonNullable currency = JsonNullable.undefined();
public static final String JSON_PROPERTY_ISSUE_NAME = "issueName";
private JsonNullable issueName = JsonNullable.undefined();
public static final String JSON_PROPERTY_PAR_AMT = "parAmt";
private JsonNullable parAmt = JsonNullable.undefined();
public static final String JSON_PROPERTY_PARENT_NAME = "parentName";
private JsonNullable parentName = JsonNullable.undefined();
public static final String JSON_PROPERTY_RATING_FITCH = "ratingFitch";
private JsonNullable ratingFitch = JsonNullable.undefined();
public static final String JSON_PROPERTY_RATING_FITCH_DATES = "ratingFitchDates";
private JsonNullable> ratingFitchDates = JsonNullable.>undefined();
public static final String JSON_PROPERTY_RATING_FITCH_VALUES = "ratingFitchValues";
private JsonNullable> ratingFitchValues = JsonNullable.>undefined();
public static final String JSON_PROPERTY_RATING_MOODYS_DATES = "ratingMoodysDates";
private JsonNullable> ratingMoodysDates = JsonNullable.>undefined();
public static final String JSON_PROPERTY_RATING_MOODYS_VALUES = "ratingMoodysValues";
private JsonNullable> ratingMoodysValues = JsonNullable.>undefined();
public static final String JSON_PROPERTY_RATING_SP_DATES = "ratingSpDates";
private JsonNullable> ratingSpDates = JsonNullable.>undefined();
public static final String JSON_PROPERTY_RATING_SP_VALUES = "ratingSpValues";
private JsonNullable> ratingSpValues = JsonNullable.>undefined();
public static final String JSON_PROPERTY_SECONDARY_TO_VENDOR_FLAG = "secondaryToVendorFlag";
private JsonNullable secondaryToVendorFlag = JsonNullable.undefined();
public static final String JSON_PROPERTY_SECTOR = "sector";
private JsonNullable sector = JsonNullable.undefined();
public static final String JSON_PROPERTY_SECTOR_BARCLAY1 = "sectorBarclay1";
private JsonNullable sectorBarclay1 = JsonNullable.undefined();
public static final String JSON_PROPERTY_SECTOR_BARCLAY2 = "sectorBarclay2";
private JsonNullable sectorBarclay2 = JsonNullable.undefined();
public static final String JSON_PROPERTY_SECTOR_BARCLAY3 = "sectorBarclay3";
private JsonNullable sectorBarclay3 = JsonNullable.undefined();
public static final String JSON_PROPERTY_SECTOR_BARCLAY4 = "sectorBarclay4";
private JsonNullable sectorBarclay4 = JsonNullable.undefined();
public static final String JSON_PROPERTY_SECTOR_DEF = "sectorDef";
private JsonNullable sectorDef = JsonNullable.undefined();
public static final String JSON_PROPERTY_SECTOR_INDUSTRY = "sectorIndustry";
private JsonNullable sectorIndustry = JsonNullable.undefined();
public static final String JSON_PROPERTY_SECTOR_MAIN = "sectorMain";
private JsonNullable sectorMain = JsonNullable.undefined();
public static final String JSON_PROPERTY_SECTOR_MERRILL1 = "sectorMerrill1";
private JsonNullable sectorMerrill1 = JsonNullable.undefined();
public static final String JSON_PROPERTY_SECTOR_MERRILL2 = "sectorMerrill2";
private JsonNullable sectorMerrill2 = JsonNullable.undefined();
public static final String JSON_PROPERTY_SECTOR_MERRILL3 = "sectorMerrill3";
private JsonNullable sectorMerrill3 = JsonNullable.undefined();
public static final String JSON_PROPERTY_SECTOR_MERRILL4 = "sectorMerrill4";
private JsonNullable sectorMerrill4 = JsonNullable.undefined();
public static final String JSON_PROPERTY_SECTOR_SUB_GROUP = "sectorSubGroup";
private JsonNullable sectorSubGroup = JsonNullable.undefined();
public static final String JSON_PROPERTY_VENDOR_COVERAGE_DATE = "vendorCoverageDate";
private JsonNullable vendorCoverageDate = JsonNullable.undefined();
/**
* Gets or Sets securityType
*/
public enum SecurityTypeEnum {
BOND("Bond"),
CCF("CCF");
private String value;
SecurityTypeEnum(String value) {
this.value = value;
}
@JsonValue
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
@JsonCreator
public static SecurityTypeEnum fromValue(String value) {
for (SecurityTypeEnum b : SecurityTypeEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
throw new IllegalArgumentException("Unexpected value '" + value + "'");
}
}
public static final String JSON_PROPERTY_SECURITY_TYPE = "securityType";
private SecurityTypeEnum securityType;
public SMCustomCashFlowFields() {
}
@JsonCreator
public SMCustomCashFlowFields(
@JsonProperty(value=JSON_PROPERTY_SECURITY_TYPE, required=true) SecurityTypeEnum securityType
) {
this();
this.securityType = securityType;
}
public SMCustomCashFlowFields cashFlowAmounts(java.util.List cashFlowAmounts) {
this.cashFlowAmounts = JsonNullable.>of(cashFlowAmounts);
return this;
}
public SMCustomCashFlowFields addCashFlowAmountsItem(Double cashFlowAmountsItem) {
if (this.cashFlowAmounts == null || !this.cashFlowAmounts.isPresent()) {
this.cashFlowAmounts = JsonNullable.>of(new java.util.ArrayList<>());
}
try {
this.cashFlowAmounts.get().add(cashFlowAmountsItem);
} catch (java.util.NoSuchElementException e) {
// this can never happen, as we make sure above that the value is present
}
return this;
}
/**
* Get cashFlowAmounts
* @return cashFlowAmounts
**/
@jakarta.annotation.Nullable
@ApiModelProperty(value = "")
@JsonIgnore
public java.util.List getCashFlowAmounts() {
return cashFlowAmounts.orElse(null);
}
@JsonProperty(JSON_PROPERTY_CASH_FLOW_AMOUNTS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable> getCashFlowAmounts_JsonNullable() {
return cashFlowAmounts;
}
@JsonProperty(JSON_PROPERTY_CASH_FLOW_AMOUNTS)
public void setCashFlowAmounts_JsonNullable(JsonNullable> cashFlowAmounts) {
this.cashFlowAmounts = cashFlowAmounts;
}
public void setCashFlowAmounts(java.util.List cashFlowAmounts) {
this.cashFlowAmounts = JsonNullable.>of(cashFlowAmounts);
}
public SMCustomCashFlowFields cashFlowDates(java.util.List cashFlowDates) {
this.cashFlowDates = JsonNullable.>of(cashFlowDates);
return this;
}
public SMCustomCashFlowFields addCashFlowDatesItem(String cashFlowDatesItem) {
if (this.cashFlowDates == null || !this.cashFlowDates.isPresent()) {
this.cashFlowDates = JsonNullable.>of(new java.util.ArrayList<>());
}
try {
this.cashFlowDates.get().add(cashFlowDatesItem);
} catch (java.util.NoSuchElementException e) {
// this can never happen, as we make sure above that the value is present
}
return this;
}
/**
* Get cashFlowDates
* @return cashFlowDates
**/
@jakarta.annotation.Nullable
@ApiModelProperty(value = "")
@JsonIgnore
public java.util.List getCashFlowDates() {
return cashFlowDates.orElse(null);
}
@JsonProperty(JSON_PROPERTY_CASH_FLOW_DATES)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable> getCashFlowDates_JsonNullable() {
return cashFlowDates;
}
@JsonProperty(JSON_PROPERTY_CASH_FLOW_DATES)
public void setCashFlowDates_JsonNullable(JsonNullable> cashFlowDates) {
this.cashFlowDates = cashFlowDates;
}
public void setCashFlowDates(java.util.List cashFlowDates) {
this.cashFlowDates = JsonNullable.>of(cashFlowDates);
}
public SMCustomCashFlowFields country(String country) {
this.country = JsonNullable.of(country);
return this;
}
/**
* Get country
* @return country
**/
@jakarta.annotation.Nullable
@ApiModelProperty(value = "")
@JsonIgnore
public String getCountry() {
return country.orElse(null);
}
@JsonProperty(JSON_PROPERTY_COUNTRY)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable getCountry_JsonNullable() {
return country;
}
@JsonProperty(JSON_PROPERTY_COUNTRY)
public void setCountry_JsonNullable(JsonNullable country) {
this.country = country;
}
public void setCountry(String country) {
this.country = JsonNullable.of(country);
}
public SMCustomCashFlowFields currency(String currency) {
this.currency = JsonNullable.of(currency);
return this;
}
/**
* Get currency
* @return currency
**/
@jakarta.annotation.Nullable
@ApiModelProperty(value = "")
@JsonIgnore
public String getCurrency() {
return currency.orElse(null);
}
@JsonProperty(JSON_PROPERTY_CURRENCY)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable getCurrency_JsonNullable() {
return currency;
}
@JsonProperty(JSON_PROPERTY_CURRENCY)
public void setCurrency_JsonNullable(JsonNullable currency) {
this.currency = currency;
}
public void setCurrency(String currency) {
this.currency = JsonNullable.of(currency);
}
public SMCustomCashFlowFields issueName(String issueName) {
this.issueName = JsonNullable.of(issueName);
return this;
}
/**
* Get issueName
* @return issueName
**/
@jakarta.annotation.Nullable
@ApiModelProperty(value = "")
@JsonIgnore
public String getIssueName() {
return issueName.orElse(null);
}
@JsonProperty(JSON_PROPERTY_ISSUE_NAME)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable getIssueName_JsonNullable() {
return issueName;
}
@JsonProperty(JSON_PROPERTY_ISSUE_NAME)
public void setIssueName_JsonNullable(JsonNullable issueName) {
this.issueName = issueName;
}
public void setIssueName(String issueName) {
this.issueName = JsonNullable.of(issueName);
}
public SMCustomCashFlowFields parAmt(Double parAmt) {
this.parAmt = JsonNullable.of(parAmt);
return this;
}
/**
* Get parAmt
* @return parAmt
**/
@jakarta.annotation.Nullable
@ApiModelProperty(value = "")
@JsonIgnore
public Double getParAmt() {
return parAmt.orElse(null);
}
@JsonProperty(JSON_PROPERTY_PAR_AMT)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable getParAmt_JsonNullable() {
return parAmt;
}
@JsonProperty(JSON_PROPERTY_PAR_AMT)
public void setParAmt_JsonNullable(JsonNullable parAmt) {
this.parAmt = parAmt;
}
public void setParAmt(Double parAmt) {
this.parAmt = JsonNullable.of(parAmt);
}
public SMCustomCashFlowFields parentName(String parentName) {
this.parentName = JsonNullable.of(parentName);
return this;
}
/**
* Get parentName
* @return parentName
**/
@jakarta.annotation.Nullable
@ApiModelProperty(value = "")
@JsonIgnore
public String getParentName() {
return parentName.orElse(null);
}
@JsonProperty(JSON_PROPERTY_PARENT_NAME)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable getParentName_JsonNullable() {
return parentName;
}
@JsonProperty(JSON_PROPERTY_PARENT_NAME)
public void setParentName_JsonNullable(JsonNullable parentName) {
this.parentName = parentName;
}
public void setParentName(String parentName) {
this.parentName = JsonNullable.of(parentName);
}
public SMCustomCashFlowFields ratingFitch(String ratingFitch) {
this.ratingFitch = JsonNullable.of(ratingFitch);
return this;
}
/**
* Get ratingFitch
* @return ratingFitch
**/
@jakarta.annotation.Nullable
@ApiModelProperty(value = "")
@JsonIgnore
public String getRatingFitch() {
return ratingFitch.orElse(null);
}
@JsonProperty(JSON_PROPERTY_RATING_FITCH)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable getRatingFitch_JsonNullable() {
return ratingFitch;
}
@JsonProperty(JSON_PROPERTY_RATING_FITCH)
public void setRatingFitch_JsonNullable(JsonNullable ratingFitch) {
this.ratingFitch = ratingFitch;
}
public void setRatingFitch(String ratingFitch) {
this.ratingFitch = JsonNullable.of(ratingFitch);
}
public SMCustomCashFlowFields ratingFitchDates(java.util.List ratingFitchDates) {
this.ratingFitchDates = JsonNullable.>of(ratingFitchDates);
return this;
}
public SMCustomCashFlowFields addRatingFitchDatesItem(String ratingFitchDatesItem) {
if (this.ratingFitchDates == null || !this.ratingFitchDates.isPresent()) {
this.ratingFitchDates = JsonNullable.>of(new java.util.ArrayList<>());
}
try {
this.ratingFitchDates.get().add(ratingFitchDatesItem);
} catch (java.util.NoSuchElementException e) {
// this can never happen, as we make sure above that the value is present
}
return this;
}
/**
* Get ratingFitchDates
* @return ratingFitchDates
**/
@jakarta.annotation.Nullable
@ApiModelProperty(value = "")
@JsonIgnore
public java.util.List getRatingFitchDates() {
return ratingFitchDates.orElse(null);
}
@JsonProperty(JSON_PROPERTY_RATING_FITCH_DATES)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable> getRatingFitchDates_JsonNullable() {
return ratingFitchDates;
}
@JsonProperty(JSON_PROPERTY_RATING_FITCH_DATES)
public void setRatingFitchDates_JsonNullable(JsonNullable> ratingFitchDates) {
this.ratingFitchDates = ratingFitchDates;
}
public void setRatingFitchDates(java.util.List ratingFitchDates) {
this.ratingFitchDates = JsonNullable.>of(ratingFitchDates);
}
public SMCustomCashFlowFields ratingFitchValues(java.util.List ratingFitchValues) {
this.ratingFitchValues = JsonNullable.>of(ratingFitchValues);
return this;
}
public SMCustomCashFlowFields addRatingFitchValuesItem(String ratingFitchValuesItem) {
if (this.ratingFitchValues == null || !this.ratingFitchValues.isPresent()) {
this.ratingFitchValues = JsonNullable.>of(new java.util.ArrayList<>());
}
try {
this.ratingFitchValues.get().add(ratingFitchValuesItem);
} catch (java.util.NoSuchElementException e) {
// this can never happen, as we make sure above that the value is present
}
return this;
}
/**
* Get ratingFitchValues
* @return ratingFitchValues
**/
@jakarta.annotation.Nullable
@ApiModelProperty(value = "")
@JsonIgnore
public java.util.List getRatingFitchValues() {
return ratingFitchValues.orElse(null);
}
@JsonProperty(JSON_PROPERTY_RATING_FITCH_VALUES)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable> getRatingFitchValues_JsonNullable() {
return ratingFitchValues;
}
@JsonProperty(JSON_PROPERTY_RATING_FITCH_VALUES)
public void setRatingFitchValues_JsonNullable(JsonNullable> ratingFitchValues) {
this.ratingFitchValues = ratingFitchValues;
}
public void setRatingFitchValues(java.util.List ratingFitchValues) {
this.ratingFitchValues = JsonNullable.>of(ratingFitchValues);
}
public SMCustomCashFlowFields ratingMoodysDates(java.util.List ratingMoodysDates) {
this.ratingMoodysDates = JsonNullable.>of(ratingMoodysDates);
return this;
}
public SMCustomCashFlowFields addRatingMoodysDatesItem(String ratingMoodysDatesItem) {
if (this.ratingMoodysDates == null || !this.ratingMoodysDates.isPresent()) {
this.ratingMoodysDates = JsonNullable.>of(new java.util.ArrayList<>());
}
try {
this.ratingMoodysDates.get().add(ratingMoodysDatesItem);
} catch (java.util.NoSuchElementException e) {
// this can never happen, as we make sure above that the value is present
}
return this;
}
/**
* Get ratingMoodysDates
* @return ratingMoodysDates
**/
@jakarta.annotation.Nullable
@ApiModelProperty(value = "")
@JsonIgnore
public java.util.List getRatingMoodysDates() {
return ratingMoodysDates.orElse(null);
}
@JsonProperty(JSON_PROPERTY_RATING_MOODYS_DATES)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable> getRatingMoodysDates_JsonNullable() {
return ratingMoodysDates;
}
@JsonProperty(JSON_PROPERTY_RATING_MOODYS_DATES)
public void setRatingMoodysDates_JsonNullable(JsonNullable> ratingMoodysDates) {
this.ratingMoodysDates = ratingMoodysDates;
}
public void setRatingMoodysDates(java.util.List ratingMoodysDates) {
this.ratingMoodysDates = JsonNullable.>of(ratingMoodysDates);
}
public SMCustomCashFlowFields ratingMoodysValues(java.util.List ratingMoodysValues) {
this.ratingMoodysValues = JsonNullable.>of(ratingMoodysValues);
return this;
}
public SMCustomCashFlowFields addRatingMoodysValuesItem(String ratingMoodysValuesItem) {
if (this.ratingMoodysValues == null || !this.ratingMoodysValues.isPresent()) {
this.ratingMoodysValues = JsonNullable.>of(new java.util.ArrayList<>());
}
try {
this.ratingMoodysValues.get().add(ratingMoodysValuesItem);
} catch (java.util.NoSuchElementException e) {
// this can never happen, as we make sure above that the value is present
}
return this;
}
/**
* Get ratingMoodysValues
* @return ratingMoodysValues
**/
@jakarta.annotation.Nullable
@ApiModelProperty(value = "")
@JsonIgnore
public java.util.List getRatingMoodysValues() {
return ratingMoodysValues.orElse(null);
}
@JsonProperty(JSON_PROPERTY_RATING_MOODYS_VALUES)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable> getRatingMoodysValues_JsonNullable() {
return ratingMoodysValues;
}
@JsonProperty(JSON_PROPERTY_RATING_MOODYS_VALUES)
public void setRatingMoodysValues_JsonNullable(JsonNullable> ratingMoodysValues) {
this.ratingMoodysValues = ratingMoodysValues;
}
public void setRatingMoodysValues(java.util.List ratingMoodysValues) {
this.ratingMoodysValues = JsonNullable.>of(ratingMoodysValues);
}
public SMCustomCashFlowFields ratingSpDates(java.util.List ratingSpDates) {
this.ratingSpDates = JsonNullable.>of(ratingSpDates);
return this;
}
public SMCustomCashFlowFields addRatingSpDatesItem(String ratingSpDatesItem) {
if (this.ratingSpDates == null || !this.ratingSpDates.isPresent()) {
this.ratingSpDates = JsonNullable.>of(new java.util.ArrayList<>());
}
try {
this.ratingSpDates.get().add(ratingSpDatesItem);
} catch (java.util.NoSuchElementException e) {
// this can never happen, as we make sure above that the value is present
}
return this;
}
/**
* Get ratingSpDates
* @return ratingSpDates
**/
@jakarta.annotation.Nullable
@ApiModelProperty(value = "")
@JsonIgnore
public java.util.List getRatingSpDates() {
return ratingSpDates.orElse(null);
}
@JsonProperty(JSON_PROPERTY_RATING_SP_DATES)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable> getRatingSpDates_JsonNullable() {
return ratingSpDates;
}
@JsonProperty(JSON_PROPERTY_RATING_SP_DATES)
public void setRatingSpDates_JsonNullable(JsonNullable> ratingSpDates) {
this.ratingSpDates = ratingSpDates;
}
public void setRatingSpDates(java.util.List ratingSpDates) {
this.ratingSpDates = JsonNullable.>of(ratingSpDates);
}
public SMCustomCashFlowFields ratingSpValues(java.util.List ratingSpValues) {
this.ratingSpValues = JsonNullable.>of(ratingSpValues);
return this;
}
public SMCustomCashFlowFields addRatingSpValuesItem(String ratingSpValuesItem) {
if (this.ratingSpValues == null || !this.ratingSpValues.isPresent()) {
this.ratingSpValues = JsonNullable.>of(new java.util.ArrayList<>());
}
try {
this.ratingSpValues.get().add(ratingSpValuesItem);
} catch (java.util.NoSuchElementException e) {
// this can never happen, as we make sure above that the value is present
}
return this;
}
/**
* Get ratingSpValues
* @return ratingSpValues
**/
@jakarta.annotation.Nullable
@ApiModelProperty(value = "")
@JsonIgnore
public java.util.List getRatingSpValues() {
return ratingSpValues.orElse(null);
}
@JsonProperty(JSON_PROPERTY_RATING_SP_VALUES)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable> getRatingSpValues_JsonNullable() {
return ratingSpValues;
}
@JsonProperty(JSON_PROPERTY_RATING_SP_VALUES)
public void setRatingSpValues_JsonNullable(JsonNullable> ratingSpValues) {
this.ratingSpValues = ratingSpValues;
}
public void setRatingSpValues(java.util.List ratingSpValues) {
this.ratingSpValues = JsonNullable.>of(ratingSpValues);
}
public SMCustomCashFlowFields secondaryToVendorFlag(Boolean secondaryToVendorFlag) {
this.secondaryToVendorFlag = JsonNullable.of(secondaryToVendorFlag);
return this;
}
/**
* Get secondaryToVendorFlag
* @return secondaryToVendorFlag
**/
@jakarta.annotation.Nullable
@ApiModelProperty(value = "")
@JsonIgnore
public Boolean getSecondaryToVendorFlag() {
return secondaryToVendorFlag.orElse(null);
}
@JsonProperty(JSON_PROPERTY_SECONDARY_TO_VENDOR_FLAG)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable getSecondaryToVendorFlag_JsonNullable() {
return secondaryToVendorFlag;
}
@JsonProperty(JSON_PROPERTY_SECONDARY_TO_VENDOR_FLAG)
public void setSecondaryToVendorFlag_JsonNullable(JsonNullable secondaryToVendorFlag) {
this.secondaryToVendorFlag = secondaryToVendorFlag;
}
public void setSecondaryToVendorFlag(Boolean secondaryToVendorFlag) {
this.secondaryToVendorFlag = JsonNullable.of(secondaryToVendorFlag);
}
public SMCustomCashFlowFields sector(String sector) {
this.sector = JsonNullable.of(sector);
return this;
}
/**
* Get sector
* @return sector
**/
@jakarta.annotation.Nullable
@ApiModelProperty(value = "")
@JsonIgnore
public String getSector() {
return sector.orElse(null);
}
@JsonProperty(JSON_PROPERTY_SECTOR)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable getSector_JsonNullable() {
return sector;
}
@JsonProperty(JSON_PROPERTY_SECTOR)
public void setSector_JsonNullable(JsonNullable sector) {
this.sector = sector;
}
public void setSector(String sector) {
this.sector = JsonNullable.of(sector);
}
public SMCustomCashFlowFields sectorBarclay1(String sectorBarclay1) {
this.sectorBarclay1 = JsonNullable.of(sectorBarclay1);
return this;
}
/**
* Get sectorBarclay1
* @return sectorBarclay1
**/
@jakarta.annotation.Nullable
@ApiModelProperty(value = "")
@JsonIgnore
public String getSectorBarclay1() {
return sectorBarclay1.orElse(null);
}
@JsonProperty(JSON_PROPERTY_SECTOR_BARCLAY1)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable getSectorBarclay1_JsonNullable() {
return sectorBarclay1;
}
@JsonProperty(JSON_PROPERTY_SECTOR_BARCLAY1)
public void setSectorBarclay1_JsonNullable(JsonNullable sectorBarclay1) {
this.sectorBarclay1 = sectorBarclay1;
}
public void setSectorBarclay1(String sectorBarclay1) {
this.sectorBarclay1 = JsonNullable.of(sectorBarclay1);
}
public SMCustomCashFlowFields sectorBarclay2(String sectorBarclay2) {
this.sectorBarclay2 = JsonNullable.of(sectorBarclay2);
return this;
}
/**
* Get sectorBarclay2
* @return sectorBarclay2
**/
@jakarta.annotation.Nullable
@ApiModelProperty(value = "")
@JsonIgnore
public String getSectorBarclay2() {
return sectorBarclay2.orElse(null);
}
@JsonProperty(JSON_PROPERTY_SECTOR_BARCLAY2)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable getSectorBarclay2_JsonNullable() {
return sectorBarclay2;
}
@JsonProperty(JSON_PROPERTY_SECTOR_BARCLAY2)
public void setSectorBarclay2_JsonNullable(JsonNullable sectorBarclay2) {
this.sectorBarclay2 = sectorBarclay2;
}
public void setSectorBarclay2(String sectorBarclay2) {
this.sectorBarclay2 = JsonNullable.of(sectorBarclay2);
}
public SMCustomCashFlowFields sectorBarclay3(String sectorBarclay3) {
this.sectorBarclay3 = JsonNullable.of(sectorBarclay3);
return this;
}
/**
* Get sectorBarclay3
* @return sectorBarclay3
**/
@jakarta.annotation.Nullable
@ApiModelProperty(value = "")
@JsonIgnore
public String getSectorBarclay3() {
return sectorBarclay3.orElse(null);
}
@JsonProperty(JSON_PROPERTY_SECTOR_BARCLAY3)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable getSectorBarclay3_JsonNullable() {
return sectorBarclay3;
}
@JsonProperty(JSON_PROPERTY_SECTOR_BARCLAY3)
public void setSectorBarclay3_JsonNullable(JsonNullable sectorBarclay3) {
this.sectorBarclay3 = sectorBarclay3;
}
public void setSectorBarclay3(String sectorBarclay3) {
this.sectorBarclay3 = JsonNullable.of(sectorBarclay3);
}
public SMCustomCashFlowFields sectorBarclay4(String sectorBarclay4) {
this.sectorBarclay4 = JsonNullable.of(sectorBarclay4);
return this;
}
/**
* Get sectorBarclay4
* @return sectorBarclay4
**/
@jakarta.annotation.Nullable
@ApiModelProperty(value = "")
@JsonIgnore
public String getSectorBarclay4() {
return sectorBarclay4.orElse(null);
}
@JsonProperty(JSON_PROPERTY_SECTOR_BARCLAY4)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable getSectorBarclay4_JsonNullable() {
return sectorBarclay4;
}
@JsonProperty(JSON_PROPERTY_SECTOR_BARCLAY4)
public void setSectorBarclay4_JsonNullable(JsonNullable sectorBarclay4) {
this.sectorBarclay4 = sectorBarclay4;
}
public void setSectorBarclay4(String sectorBarclay4) {
this.sectorBarclay4 = JsonNullable.of(sectorBarclay4);
}
public SMCustomCashFlowFields sectorDef(String sectorDef) {
this.sectorDef = JsonNullable.of(sectorDef);
return this;
}
/**
* Get sectorDef
* @return sectorDef
**/
@jakarta.annotation.Nullable
@ApiModelProperty(value = "")
@JsonIgnore
public String getSectorDef() {
return sectorDef.orElse(null);
}
@JsonProperty(JSON_PROPERTY_SECTOR_DEF)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable getSectorDef_JsonNullable() {
return sectorDef;
}
@JsonProperty(JSON_PROPERTY_SECTOR_DEF)
public void setSectorDef_JsonNullable(JsonNullable sectorDef) {
this.sectorDef = sectorDef;
}
public void setSectorDef(String sectorDef) {
this.sectorDef = JsonNullable.of(sectorDef);
}
public SMCustomCashFlowFields sectorIndustry(String sectorIndustry) {
this.sectorIndustry = JsonNullable.of(sectorIndustry);
return this;
}
/**
* Get sectorIndustry
* @return sectorIndustry
**/
@jakarta.annotation.Nullable
@ApiModelProperty(value = "")
@JsonIgnore
public String getSectorIndustry() {
return sectorIndustry.orElse(null);
}
@JsonProperty(JSON_PROPERTY_SECTOR_INDUSTRY)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable getSectorIndustry_JsonNullable() {
return sectorIndustry;
}
@JsonProperty(JSON_PROPERTY_SECTOR_INDUSTRY)
public void setSectorIndustry_JsonNullable(JsonNullable sectorIndustry) {
this.sectorIndustry = sectorIndustry;
}
public void setSectorIndustry(String sectorIndustry) {
this.sectorIndustry = JsonNullable.of(sectorIndustry);
}
public SMCustomCashFlowFields sectorMain(String sectorMain) {
this.sectorMain = JsonNullable.of(sectorMain);
return this;
}
/**
* Get sectorMain
* @return sectorMain
**/
@jakarta.annotation.Nullable
@ApiModelProperty(value = "")
@JsonIgnore
public String getSectorMain() {
return sectorMain.orElse(null);
}
@JsonProperty(JSON_PROPERTY_SECTOR_MAIN)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable getSectorMain_JsonNullable() {
return sectorMain;
}
@JsonProperty(JSON_PROPERTY_SECTOR_MAIN)
public void setSectorMain_JsonNullable(JsonNullable sectorMain) {
this.sectorMain = sectorMain;
}
public void setSectorMain(String sectorMain) {
this.sectorMain = JsonNullable.of(sectorMain);
}
public SMCustomCashFlowFields sectorMerrill1(String sectorMerrill1) {
this.sectorMerrill1 = JsonNullable.of(sectorMerrill1);
return this;
}
/**
* Get sectorMerrill1
* @return sectorMerrill1
**/
@jakarta.annotation.Nullable
@ApiModelProperty(value = "")
@JsonIgnore
public String getSectorMerrill1() {
return sectorMerrill1.orElse(null);
}
@JsonProperty(JSON_PROPERTY_SECTOR_MERRILL1)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable getSectorMerrill1_JsonNullable() {
return sectorMerrill1;
}
@JsonProperty(JSON_PROPERTY_SECTOR_MERRILL1)
public void setSectorMerrill1_JsonNullable(JsonNullable sectorMerrill1) {
this.sectorMerrill1 = sectorMerrill1;
}
public void setSectorMerrill1(String sectorMerrill1) {
this.sectorMerrill1 = JsonNullable.of(sectorMerrill1);
}
public SMCustomCashFlowFields sectorMerrill2(String sectorMerrill2) {
this.sectorMerrill2 = JsonNullable.of(sectorMerrill2);
return this;
}
/**
* Get sectorMerrill2
* @return sectorMerrill2
**/
@jakarta.annotation.Nullable
@ApiModelProperty(value = "")
@JsonIgnore
public String getSectorMerrill2() {
return sectorMerrill2.orElse(null);
}
@JsonProperty(JSON_PROPERTY_SECTOR_MERRILL2)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable getSectorMerrill2_JsonNullable() {
return sectorMerrill2;
}
@JsonProperty(JSON_PROPERTY_SECTOR_MERRILL2)
public void setSectorMerrill2_JsonNullable(JsonNullable sectorMerrill2) {
this.sectorMerrill2 = sectorMerrill2;
}
public void setSectorMerrill2(String sectorMerrill2) {
this.sectorMerrill2 = JsonNullable.of(sectorMerrill2);
}
public SMCustomCashFlowFields sectorMerrill3(String sectorMerrill3) {
this.sectorMerrill3 = JsonNullable.of(sectorMerrill3);
return this;
}
/**
* Get sectorMerrill3
* @return sectorMerrill3
**/
@jakarta.annotation.Nullable
@ApiModelProperty(value = "")
@JsonIgnore
public String getSectorMerrill3() {
return sectorMerrill3.orElse(null);
}
@JsonProperty(JSON_PROPERTY_SECTOR_MERRILL3)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable getSectorMerrill3_JsonNullable() {
return sectorMerrill3;
}
@JsonProperty(JSON_PROPERTY_SECTOR_MERRILL3)
public void setSectorMerrill3_JsonNullable(JsonNullable sectorMerrill3) {
this.sectorMerrill3 = sectorMerrill3;
}
public void setSectorMerrill3(String sectorMerrill3) {
this.sectorMerrill3 = JsonNullable.of(sectorMerrill3);
}
public SMCustomCashFlowFields sectorMerrill4(String sectorMerrill4) {
this.sectorMerrill4 = JsonNullable.of(sectorMerrill4);
return this;
}
/**
* Get sectorMerrill4
* @return sectorMerrill4
**/
@jakarta.annotation.Nullable
@ApiModelProperty(value = "")
@JsonIgnore
public String getSectorMerrill4() {
return sectorMerrill4.orElse(null);
}
@JsonProperty(JSON_PROPERTY_SECTOR_MERRILL4)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable getSectorMerrill4_JsonNullable() {
return sectorMerrill4;
}
@JsonProperty(JSON_PROPERTY_SECTOR_MERRILL4)
public void setSectorMerrill4_JsonNullable(JsonNullable sectorMerrill4) {
this.sectorMerrill4 = sectorMerrill4;
}
public void setSectorMerrill4(String sectorMerrill4) {
this.sectorMerrill4 = JsonNullable.of(sectorMerrill4);
}
public SMCustomCashFlowFields sectorSubGroup(String sectorSubGroup) {
this.sectorSubGroup = JsonNullable.of(sectorSubGroup);
return this;
}
/**
* Get sectorSubGroup
* @return sectorSubGroup
**/
@jakarta.annotation.Nullable
@ApiModelProperty(value = "")
@JsonIgnore
public String getSectorSubGroup() {
return sectorSubGroup.orElse(null);
}
@JsonProperty(JSON_PROPERTY_SECTOR_SUB_GROUP)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable getSectorSubGroup_JsonNullable() {
return sectorSubGroup;
}
@JsonProperty(JSON_PROPERTY_SECTOR_SUB_GROUP)
public void setSectorSubGroup_JsonNullable(JsonNullable sectorSubGroup) {
this.sectorSubGroup = sectorSubGroup;
}
public void setSectorSubGroup(String sectorSubGroup) {
this.sectorSubGroup = JsonNullable.of(sectorSubGroup);
}
public SMCustomCashFlowFields vendorCoverageDate(String vendorCoverageDate) {
this.vendorCoverageDate = JsonNullable.of(vendorCoverageDate);
return this;
}
/**
* Get vendorCoverageDate
* @return vendorCoverageDate
**/
@jakarta.annotation.Nullable
@ApiModelProperty(value = "")
@JsonIgnore
public String getVendorCoverageDate() {
return vendorCoverageDate.orElse(null);
}
@JsonProperty(JSON_PROPERTY_VENDOR_COVERAGE_DATE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable getVendorCoverageDate_JsonNullable() {
return vendorCoverageDate;
}
@JsonProperty(JSON_PROPERTY_VENDOR_COVERAGE_DATE)
public void setVendorCoverageDate_JsonNullable(JsonNullable vendorCoverageDate) {
this.vendorCoverageDate = vendorCoverageDate;
}
public void setVendorCoverageDate(String vendorCoverageDate) {
this.vendorCoverageDate = JsonNullable.of(vendorCoverageDate);
}
public SMCustomCashFlowFields securityType(SecurityTypeEnum securityType) {
this.securityType = securityType;
return this;
}
/**
* Get securityType
* @return securityType
**/
@jakarta.annotation.Nonnull
@ApiModelProperty(required = true, value = "")
@JsonProperty(JSON_PROPERTY_SECURITY_TYPE)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public SecurityTypeEnum getSecurityType() {
return securityType;
}
@JsonProperty(JSON_PROPERTY_SECURITY_TYPE)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setSecurityType(SecurityTypeEnum securityType) {
this.securityType = securityType;
}
/**
* Return true if this SMCustomCashFlowFields object is equal to o.
*/
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
SMCustomCashFlowFields smCustomCashFlowFields = (SMCustomCashFlowFields) o;
return equalsNullable(this.cashFlowAmounts, smCustomCashFlowFields.cashFlowAmounts) &&
equalsNullable(this.cashFlowDates, smCustomCashFlowFields.cashFlowDates) &&
equalsNullable(this.country, smCustomCashFlowFields.country) &&
equalsNullable(this.currency, smCustomCashFlowFields.currency) &&
equalsNullable(this.issueName, smCustomCashFlowFields.issueName) &&
equalsNullable(this.parAmt, smCustomCashFlowFields.parAmt) &&
equalsNullable(this.parentName, smCustomCashFlowFields.parentName) &&
equalsNullable(this.ratingFitch, smCustomCashFlowFields.ratingFitch) &&
equalsNullable(this.ratingFitchDates, smCustomCashFlowFields.ratingFitchDates) &&
equalsNullable(this.ratingFitchValues, smCustomCashFlowFields.ratingFitchValues) &&
equalsNullable(this.ratingMoodysDates, smCustomCashFlowFields.ratingMoodysDates) &&
equalsNullable(this.ratingMoodysValues, smCustomCashFlowFields.ratingMoodysValues) &&
equalsNullable(this.ratingSpDates, smCustomCashFlowFields.ratingSpDates) &&
equalsNullable(this.ratingSpValues, smCustomCashFlowFields.ratingSpValues) &&
equalsNullable(this.secondaryToVendorFlag, smCustomCashFlowFields.secondaryToVendorFlag) &&
equalsNullable(this.sector, smCustomCashFlowFields.sector) &&
equalsNullable(this.sectorBarclay1, smCustomCashFlowFields.sectorBarclay1) &&
equalsNullable(this.sectorBarclay2, smCustomCashFlowFields.sectorBarclay2) &&
equalsNullable(this.sectorBarclay3, smCustomCashFlowFields.sectorBarclay3) &&
equalsNullable(this.sectorBarclay4, smCustomCashFlowFields.sectorBarclay4) &&
equalsNullable(this.sectorDef, smCustomCashFlowFields.sectorDef) &&
equalsNullable(this.sectorIndustry, smCustomCashFlowFields.sectorIndustry) &&
equalsNullable(this.sectorMain, smCustomCashFlowFields.sectorMain) &&
equalsNullable(this.sectorMerrill1, smCustomCashFlowFields.sectorMerrill1) &&
equalsNullable(this.sectorMerrill2, smCustomCashFlowFields.sectorMerrill2) &&
equalsNullable(this.sectorMerrill3, smCustomCashFlowFields.sectorMerrill3) &&
equalsNullable(this.sectorMerrill4, smCustomCashFlowFields.sectorMerrill4) &&
equalsNullable(this.sectorSubGroup, smCustomCashFlowFields.sectorSubGroup) &&
equalsNullable(this.vendorCoverageDate, smCustomCashFlowFields.vendorCoverageDate) &&
Objects.equals(this.securityType, smCustomCashFlowFields.securityType);
}
private static boolean equalsNullable(JsonNullable a, JsonNullable b) {
return a == b || (a != null && b != null && a.isPresent() && b.isPresent() && Objects.deepEquals(a.get(), b.get()));
}
@Override
public int hashCode() {
return Objects.hash(hashCodeNullable(cashFlowAmounts), hashCodeNullable(cashFlowDates), hashCodeNullable(country), hashCodeNullable(currency), hashCodeNullable(issueName), hashCodeNullable(parAmt), hashCodeNullable(parentName), hashCodeNullable(ratingFitch), hashCodeNullable(ratingFitchDates), hashCodeNullable(ratingFitchValues), hashCodeNullable(ratingMoodysDates), hashCodeNullable(ratingMoodysValues), hashCodeNullable(ratingSpDates), hashCodeNullable(ratingSpValues), hashCodeNullable(secondaryToVendorFlag), hashCodeNullable(sector), hashCodeNullable(sectorBarclay1), hashCodeNullable(sectorBarclay2), hashCodeNullable(sectorBarclay3), hashCodeNullable(sectorBarclay4), hashCodeNullable(sectorDef), hashCodeNullable(sectorIndustry), hashCodeNullable(sectorMain), hashCodeNullable(sectorMerrill1), hashCodeNullable(sectorMerrill2), hashCodeNullable(sectorMerrill3), hashCodeNullable(sectorMerrill4), hashCodeNullable(sectorSubGroup), hashCodeNullable(vendorCoverageDate), securityType);
}
private static int hashCodeNullable(JsonNullable a) {
if (a == null) {
return 1;
}
return a.isPresent() ? Arrays.deepHashCode(new Object[]{a.get()}) : 31;
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class SMCustomCashFlowFields {\n");
sb.append(" cashFlowAmounts: ").append(toIndentedString(cashFlowAmounts)).append("\n");
sb.append(" cashFlowDates: ").append(toIndentedString(cashFlowDates)).append("\n");
sb.append(" country: ").append(toIndentedString(country)).append("\n");
sb.append(" currency: ").append(toIndentedString(currency)).append("\n");
sb.append(" issueName: ").append(toIndentedString(issueName)).append("\n");
sb.append(" parAmt: ").append(toIndentedString(parAmt)).append("\n");
sb.append(" parentName: ").append(toIndentedString(parentName)).append("\n");
sb.append(" ratingFitch: ").append(toIndentedString(ratingFitch)).append("\n");
sb.append(" ratingFitchDates: ").append(toIndentedString(ratingFitchDates)).append("\n");
sb.append(" ratingFitchValues: ").append(toIndentedString(ratingFitchValues)).append("\n");
sb.append(" ratingMoodysDates: ").append(toIndentedString(ratingMoodysDates)).append("\n");
sb.append(" ratingMoodysValues: ").append(toIndentedString(ratingMoodysValues)).append("\n");
sb.append(" ratingSpDates: ").append(toIndentedString(ratingSpDates)).append("\n");
sb.append(" ratingSpValues: ").append(toIndentedString(ratingSpValues)).append("\n");
sb.append(" secondaryToVendorFlag: ").append(toIndentedString(secondaryToVendorFlag)).append("\n");
sb.append(" sector: ").append(toIndentedString(sector)).append("\n");
sb.append(" sectorBarclay1: ").append(toIndentedString(sectorBarclay1)).append("\n");
sb.append(" sectorBarclay2: ").append(toIndentedString(sectorBarclay2)).append("\n");
sb.append(" sectorBarclay3: ").append(toIndentedString(sectorBarclay3)).append("\n");
sb.append(" sectorBarclay4: ").append(toIndentedString(sectorBarclay4)).append("\n");
sb.append(" sectorDef: ").append(toIndentedString(sectorDef)).append("\n");
sb.append(" sectorIndustry: ").append(toIndentedString(sectorIndustry)).append("\n");
sb.append(" sectorMain: ").append(toIndentedString(sectorMain)).append("\n");
sb.append(" sectorMerrill1: ").append(toIndentedString(sectorMerrill1)).append("\n");
sb.append(" sectorMerrill2: ").append(toIndentedString(sectorMerrill2)).append("\n");
sb.append(" sectorMerrill3: ").append(toIndentedString(sectorMerrill3)).append("\n");
sb.append(" sectorMerrill4: ").append(toIndentedString(sectorMerrill4)).append("\n");
sb.append(" sectorSubGroup: ").append(toIndentedString(sectorSubGroup)).append("\n");
sb.append(" vendorCoverageDate: ").append(toIndentedString(vendorCoverageDate)).append("\n");
sb.append(" securityType: ").append(toIndentedString(securityType)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy