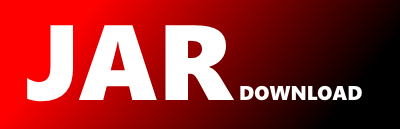
com.factset.sdk.SecurityModeling.models.SMRetrieveParameters Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of securitymodeling Show documentation
Show all versions of securitymodeling Show documentation
FactSet SDK for Java - securitymodeling
/*
* Security-Modeling API
* Allow clients to fetch Analytics through APIs.
*
* The version of the OpenAPI document: 3
* Contact: [email protected]
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.factset.sdk.SecurityModeling.models;
import java.util.Objects;
import java.util.Arrays;
import java.util.Map;
import java.util.HashMap;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonTypeName;
import com.fasterxml.jackson.annotation.JsonValue;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import org.openapitools.jackson.nullable.JsonNullable;
import com.fasterxml.jackson.annotation.JsonIgnore;
import org.openapitools.jackson.nullable.JsonNullable;
import java.util.NoSuchElementException;
import java.io.Serializable;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.factset.sdk.SecurityModeling.JSON;
/**
* SMRetrieveParameters
*/
@JsonPropertyOrder({
SMRetrieveParameters.JSON_PROPERTY_SECURITY_NAME,
SMRetrieveParameters.JSON_PROPERTY_LOCATION,
SMRetrieveParameters.JSON_PROPERTY_ASOFDATE,
SMRetrieveParameters.JSON_PROPERTY_SECURITY_TYPE
})
@jakarta.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen")
public class SMRetrieveParameters implements Serializable {
private static final long serialVersionUID = 1L;
public static final String JSON_PROPERTY_SECURITY_NAME = "securityName";
private String securityName;
public static final String JSON_PROPERTY_LOCATION = "location";
private JsonNullable location = JsonNullable.undefined();
public static final String JSON_PROPERTY_ASOFDATE = "asofdate";
private JsonNullable asofdate = JsonNullable.undefined();
/**
* Gets or Sets securityType
*/
public enum SecurityTypeEnum {
BOND("Bond"),
CCF("CCF");
private String value;
SecurityTypeEnum(String value) {
this.value = value;
}
@JsonValue
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
@JsonCreator
public static SecurityTypeEnum fromValue(String value) {
for (SecurityTypeEnum b : SecurityTypeEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
return null;
}
}
public static final String JSON_PROPERTY_SECURITY_TYPE = "securityType";
private JsonNullable securityType = JsonNullable.undefined();
public SMRetrieveParameters() {
}
@JsonCreator
public SMRetrieveParameters(
@JsonProperty(value=JSON_PROPERTY_SECURITY_NAME, required=true) String securityName
) {
this();
this.securityName = securityName;
}
public SMRetrieveParameters securityName(String securityName) {
this.securityName = securityName;
return this;
}
/**
* Get securityName
* @return securityName
**/
@jakarta.annotation.Nonnull
@ApiModelProperty(required = true, value = "")
@JsonProperty(JSON_PROPERTY_SECURITY_NAME)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public String getSecurityName() {
return securityName;
}
@JsonProperty(JSON_PROPERTY_SECURITY_NAME)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setSecurityName(String securityName) {
this.securityName = securityName;
}
public SMRetrieveParameters location(String location) {
this.location = JsonNullable.of(location);
return this;
}
/**
* Get location
* @return location
**/
@jakarta.annotation.Nullable
@ApiModelProperty(value = "")
@JsonIgnore
public String getLocation() {
return location.orElse(null);
}
@JsonProperty(JSON_PROPERTY_LOCATION)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable getLocation_JsonNullable() {
return location;
}
@JsonProperty(JSON_PROPERTY_LOCATION)
public void setLocation_JsonNullable(JsonNullable location) {
this.location = location;
}
public void setLocation(String location) {
this.location = JsonNullable.of(location);
}
public SMRetrieveParameters asofdate(String asofdate) {
this.asofdate = JsonNullable.of(asofdate);
return this;
}
/**
* Get asofdate
* @return asofdate
**/
@jakarta.annotation.Nullable
@ApiModelProperty(value = "")
@JsonIgnore
public String getAsofdate() {
return asofdate.orElse(null);
}
@JsonProperty(JSON_PROPERTY_ASOFDATE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable getAsofdate_JsonNullable() {
return asofdate;
}
@JsonProperty(JSON_PROPERTY_ASOFDATE)
public void setAsofdate_JsonNullable(JsonNullable asofdate) {
this.asofdate = asofdate;
}
public void setAsofdate(String asofdate) {
this.asofdate = JsonNullable.of(asofdate);
}
public SMRetrieveParameters securityType(SecurityTypeEnum securityType) {
this.securityType = JsonNullable.of(securityType);
return this;
}
/**
* Get securityType
* @return securityType
**/
@jakarta.annotation.Nullable
@ApiModelProperty(value = "")
@JsonIgnore
public SecurityTypeEnum getSecurityType() {
return securityType.orElse(null);
}
@JsonProperty(JSON_PROPERTY_SECURITY_TYPE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable getSecurityType_JsonNullable() {
return securityType;
}
@JsonProperty(JSON_PROPERTY_SECURITY_TYPE)
public void setSecurityType_JsonNullable(JsonNullable securityType) {
this.securityType = securityType;
}
public void setSecurityType(SecurityTypeEnum securityType) {
this.securityType = JsonNullable.of(securityType);
}
/**
* Return true if this SMRetrieveParameters object is equal to o.
*/
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
SMRetrieveParameters smRetrieveParameters = (SMRetrieveParameters) o;
return Objects.equals(this.securityName, smRetrieveParameters.securityName) &&
equalsNullable(this.location, smRetrieveParameters.location) &&
equalsNullable(this.asofdate, smRetrieveParameters.asofdate) &&
equalsNullable(this.securityType, smRetrieveParameters.securityType);
}
private static boolean equalsNullable(JsonNullable a, JsonNullable b) {
return a == b || (a != null && b != null && a.isPresent() && b.isPresent() && Objects.deepEquals(a.get(), b.get()));
}
@Override
public int hashCode() {
return Objects.hash(securityName, hashCodeNullable(location), hashCodeNullable(asofdate), hashCodeNullable(securityType));
}
private static int hashCodeNullable(JsonNullable a) {
if (a == null) {
return 1;
}
return a.isPresent() ? Arrays.deepHashCode(new Object[]{a.get()}) : 31;
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class SMRetrieveParameters {\n");
sb.append(" securityName: ").append(toIndentedString(securityName)).append("\n");
sb.append(" location: ").append(toIndentedString(location)).append("\n");
sb.append(" asofdate: ").append(toIndentedString(asofdate)).append("\n");
sb.append(" securityType: ").append(toIndentedString(securityType)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy