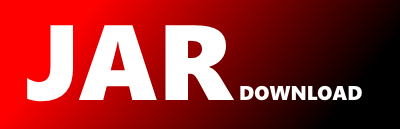
com.factset.sdk.Signals.models.EventDetails Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of signals Show documentation
Show all versions of signals Show documentation
FactSet SDK for Java - signals
The newest version!
/*
* Signals API
* Collection of endpoints for providing Signal Events, Definitions and Metadata
*
* The version of the OpenAPI document: 2.6.0
* Contact: [email protected]
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.factset.sdk.Signals.models;
import java.util.Objects;
import java.util.Arrays;
import java.util.Map;
import java.util.HashMap;
import com.factset.sdk.Signals.models.ErrorItem;
import com.factset.sdk.Signals.models.EventDetailDataItem;
import com.factset.sdk.Signals.models.EventMeta;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonTypeName;
import com.fasterxml.jackson.annotation.JsonValue;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.Serializable;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.factset.sdk.Signals.JSON;
/**
* Multiple Signals event headlines plus all additional event details
*/
@ApiModel(description = "Multiple Signals event headlines plus all additional event details")
@JsonPropertyOrder({
EventDetails.JSON_PROPERTY_DATA,
EventDetails.JSON_PROPERTY_META,
EventDetails.JSON_PROPERTY_ERRORS
})
@jakarta.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen")
public class EventDetails implements Serializable {
private static final long serialVersionUID = 1L;
public static final String JSON_PROPERTY_DATA = "data";
private java.util.List data = new java.util.ArrayList<>();
public static final String JSON_PROPERTY_META = "meta";
private EventMeta meta;
public static final String JSON_PROPERTY_ERRORS = "errors";
private java.util.List errors = null;
public EventDetails() {
}
@JsonCreator
public EventDetails(
@JsonProperty(value=JSON_PROPERTY_DATA, required=true) java.util.List data,
@JsonProperty(value=JSON_PROPERTY_META, required=true) EventMeta meta
) {
this();
this.data = data;
this.meta = meta;
}
public EventDetails data(java.util.List data) {
this.data = data;
return this;
}
public EventDetails addDataItem(EventDetailDataItem dataItem) {
this.data.add(dataItem);
return this;
}
/**
* Get data
* @return data
**/
@jakarta.annotation.Nonnull
@ApiModelProperty(required = true, value = "")
@JsonProperty(JSON_PROPERTY_DATA)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public java.util.List getData() {
return data;
}
@JsonProperty(JSON_PROPERTY_DATA)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setData(java.util.List data) {
this.data = data;
}
public EventDetails meta(EventMeta meta) {
this.meta = meta;
return this;
}
/**
* Get meta
* @return meta
**/
@jakarta.annotation.Nonnull
@ApiModelProperty(required = true, value = "")
@JsonProperty(JSON_PROPERTY_META)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public EventMeta getMeta() {
return meta;
}
@JsonProperty(JSON_PROPERTY_META)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setMeta(EventMeta meta) {
this.meta = meta;
}
public EventDetails errors(java.util.List errors) {
this.errors = errors;
return this;
}
public EventDetails addErrorsItem(ErrorItem errorsItem) {
if (this.errors == null) {
this.errors = new java.util.ArrayList<>();
}
this.errors.add(errorsItem);
return this;
}
/**
* Get errors
* @return errors
**/
@jakarta.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_ERRORS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public java.util.List getErrors() {
return errors;
}
@JsonProperty(JSON_PROPERTY_ERRORS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setErrors(java.util.List errors) {
this.errors = errors;
}
/**
* Return true if this eventDetails object is equal to o.
*/
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
EventDetails eventDetails = (EventDetails) o;
return Objects.equals(this.data, eventDetails.data) &&
Objects.equals(this.meta, eventDetails.meta) &&
Objects.equals(this.errors, eventDetails.errors);
}
@Override
public int hashCode() {
return Objects.hash(data, meta, errors);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class EventDetails {\n");
sb.append(" data: ").append(toIndentedString(data)).append("\n");
sb.append(" meta: ").append(toIndentedString(meta)).append("\n");
sb.append(" errors: ").append(toIndentedString(errors)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy