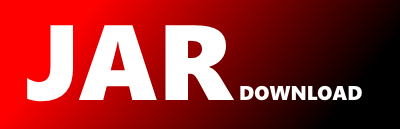
com.factset.sdk.Signals.models.EventsEntitiesPost Maven / Gradle / Ivy
/*
* Signals API
* Collection of endpoints for providing Signal Events, Definitions and Metadata
*
* The version of the OpenAPI document: 2.6.0
* Contact: [email protected]
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.factset.sdk.Signals.models;
import java.util.Objects;
import java.util.Arrays;
import java.util.Map;
import java.util.HashMap;
import com.factset.sdk.Signals.models.DateTimeInterval;
import com.factset.sdk.Signals.models.RelevanceScoreRange;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonTypeName;
import com.fasterxml.jackson.annotation.JsonValue;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import org.openapitools.jackson.nullable.JsonNullable;
import com.fasterxml.jackson.annotation.JsonIgnore;
import org.openapitools.jackson.nullable.JsonNullable;
import java.util.NoSuchElementException;
import java.io.Serializable;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.factset.sdk.Signals.JSON;
/**
* Contains list of entities that matches the request criterion
*/
@ApiModel(description = "Contains list of entities that matches the request criterion")
@JsonPropertyOrder({
EventsEntitiesPost.JSON_PROPERTY_CREATED,
EventsEntitiesPost.JSON_PROPERTY_UPDATED,
EventsEntitiesPost.JSON_PROPERTY_SIGNAL_IDS,
EventsEntitiesPost.JSON_PROPERTY_THEMES,
EventsEntitiesPost.JSON_PROPERTY_CATEGORIES,
EventsEntitiesPost.JSON_PROPERTY_USER_RELEVANCE_SCORE
})
@jakarta.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen")
public class EventsEntitiesPost implements Serializable {
private static final long serialVersionUID = 1L;
public static final String JSON_PROPERTY_CREATED = "created";
private JsonNullable created = JsonNullable.undefined();
public static final String JSON_PROPERTY_UPDATED = "updated";
private JsonNullable updated = JsonNullable.undefined();
public static final String JSON_PROPERTY_SIGNAL_IDS = "signalIds";
private String signalIds;
public static final String JSON_PROPERTY_THEMES = "themes";
private String themes;
public static final String JSON_PROPERTY_CATEGORIES = "categories";
private String categories;
public static final String JSON_PROPERTY_USER_RELEVANCE_SCORE = "userRelevanceScore";
private JsonNullable userRelevanceScore = JsonNullable.undefined();
public EventsEntitiesPost() {
}
public EventsEntitiesPost created(DateTimeInterval created) {
this.created = JsonNullable.of(created);
return this;
}
/**
* A date/time (UTC) interval for filtering signal events based on their creation date. Defaults to NOW - 7 days if omitted. Users with limited access can only provide the default or a smaller date window.
* @return created
**/
@jakarta.annotation.Nullable
@ApiModelProperty(value = "A date/time (UTC) interval for filtering signal events based on their creation date. Defaults to NOW - 7 days if omitted. Users with limited access can only provide the default or a smaller date window.")
@JsonIgnore
public DateTimeInterval getCreated() {
return created.orElse(null);
}
@JsonProperty(JSON_PROPERTY_CREATED)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable getCreated_JsonNullable() {
return created;
}
@JsonProperty(JSON_PROPERTY_CREATED)
public void setCreated_JsonNullable(JsonNullable created) {
this.created = created;
}
public void setCreated(DateTimeInterval created) {
this.created = JsonNullable.of(created);
}
public EventsEntitiesPost updated(DateTimeInterval updated) {
this.updated = JsonNullable.of(updated);
return this;
}
/**
* A date/time (UTC) interval for filtering signal events based on their last updated date. Defaults to NOW - 7 days if omitted. Users with limited access can only provide the default or a smaller date window.
* @return updated
**/
@jakarta.annotation.Nullable
@ApiModelProperty(value = "A date/time (UTC) interval for filtering signal events based on their last updated date. Defaults to NOW - 7 days if omitted. Users with limited access can only provide the default or a smaller date window.")
@JsonIgnore
public DateTimeInterval getUpdated() {
return updated.orElse(null);
}
@JsonProperty(JSON_PROPERTY_UPDATED)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable getUpdated_JsonNullable() {
return updated;
}
@JsonProperty(JSON_PROPERTY_UPDATED)
public void setUpdated_JsonNullable(JsonNullable updated) {
this.updated = updated;
}
public void setUpdated(DateTimeInterval updated) {
this.updated = JsonNullable.of(updated);
}
public EventsEntitiesPost signalIds(String signalIds) {
this.signalIds = signalIds;
return this;
}
/**
* Comma delimited string of signalIds
* @return signalIds
**/
@jakarta.annotation.Nullable
@ApiModelProperty(example = "dilutionTrigger,freeCashFlow", value = "Comma delimited string of signalIds")
@JsonProperty(JSON_PROPERTY_SIGNAL_IDS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getSignalIds() {
return signalIds;
}
@JsonProperty(JSON_PROPERTY_SIGNAL_IDS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setSignalIds(String signalIds) {
this.signalIds = signalIds;
}
public EventsEntitiesPost themes(String themes) {
this.themes = themes;
return this;
}
/**
* Comma delimited string of theme ids. Full list of signal themes can be viewed at /themes.
* @return themes
**/
@jakarta.annotation.Nullable
@ApiModelProperty(value = "Comma delimited string of theme ids. Full list of signal themes can be viewed at /themes.")
@JsonProperty(JSON_PROPERTY_THEMES)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getThemes() {
return themes;
}
@JsonProperty(JSON_PROPERTY_THEMES)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setThemes(String themes) {
this.themes = themes;
}
public EventsEntitiesPost categories(String categories) {
this.categories = categories;
return this;
}
/**
* Comma delimited string of category ids. Full list of signal categories can be viewed at /categories.
* @return categories
**/
@jakarta.annotation.Nullable
@ApiModelProperty(value = "Comma delimited string of category ids. Full list of signal categories can be viewed at /categories.")
@JsonProperty(JSON_PROPERTY_CATEGORIES)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getCategories() {
return categories;
}
@JsonProperty(JSON_PROPERTY_CATEGORIES)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setCategories(String categories) {
this.categories = categories;
}
public EventsEntitiesPost userRelevanceScore(RelevanceScoreRange userRelevanceScore) {
this.userRelevanceScore = JsonNullable.of(userRelevanceScore);
return this;
}
/**
* A range for filtering signal events based on their relevancy score.
* @return userRelevanceScore
**/
@jakarta.annotation.Nullable
@ApiModelProperty(value = "A range for filtering signal events based on their relevancy score.")
@JsonIgnore
public RelevanceScoreRange getUserRelevanceScore() {
return userRelevanceScore.orElse(null);
}
@JsonProperty(JSON_PROPERTY_USER_RELEVANCE_SCORE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable getUserRelevanceScore_JsonNullable() {
return userRelevanceScore;
}
@JsonProperty(JSON_PROPERTY_USER_RELEVANCE_SCORE)
public void setUserRelevanceScore_JsonNullable(JsonNullable userRelevanceScore) {
this.userRelevanceScore = userRelevanceScore;
}
public void setUserRelevanceScore(RelevanceScoreRange userRelevanceScore) {
this.userRelevanceScore = JsonNullable.of(userRelevanceScore);
}
/**
* Return true if this eventsEntitiesPost object is equal to o.
*/
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
EventsEntitiesPost eventsEntitiesPost = (EventsEntitiesPost) o;
return equalsNullable(this.created, eventsEntitiesPost.created) &&
equalsNullable(this.updated, eventsEntitiesPost.updated) &&
Objects.equals(this.signalIds, eventsEntitiesPost.signalIds) &&
Objects.equals(this.themes, eventsEntitiesPost.themes) &&
Objects.equals(this.categories, eventsEntitiesPost.categories) &&
equalsNullable(this.userRelevanceScore, eventsEntitiesPost.userRelevanceScore);
}
private static boolean equalsNullable(JsonNullable a, JsonNullable b) {
return a == b || (a != null && b != null && a.isPresent() && b.isPresent() && Objects.deepEquals(a.get(), b.get()));
}
@Override
public int hashCode() {
return Objects.hash(hashCodeNullable(created), hashCodeNullable(updated), signalIds, themes, categories, hashCodeNullable(userRelevanceScore));
}
private static int hashCodeNullable(JsonNullable a) {
if (a == null) {
return 1;
}
return a.isPresent() ? Arrays.deepHashCode(new Object[]{a.get()}) : 31;
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class EventsEntitiesPost {\n");
sb.append(" created: ").append(toIndentedString(created)).append("\n");
sb.append(" updated: ").append(toIndentedString(updated)).append("\n");
sb.append(" signalIds: ").append(toIndentedString(signalIds)).append("\n");
sb.append(" themes: ").append(toIndentedString(themes)).append("\n");
sb.append(" categories: ").append(toIndentedString(categories)).append("\n");
sb.append(" userRelevanceScore: ").append(toIndentedString(userRelevanceScore)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy