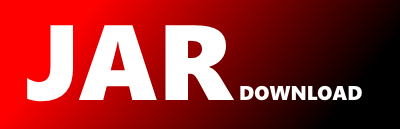
com.factual.driver.JsonUtil Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of factual-java-driver Show documentation
Show all versions of factual-java-driver Show documentation
Factual's officially supported Java driver
The newest version!
package com.factual.driver;
import java.io.IOException;
import java.util.Iterator;
import java.util.List;
import java.util.Map;
import org.codehaus.jackson.JsonGenerationException;
import org.codehaus.jackson.map.JsonMappingException;
import org.codehaus.jackson.map.ObjectMapper;
import org.json.JSONArray;
import org.json.JSONException;
import org.json.JSONObject;
import com.google.common.collect.Lists;
import com.google.common.collect.Maps;
public class JsonUtil {
/**
* Takes a JSONArray of records, where each record is a dictionary, and
* returns the translated List of Maps.
*/
public static List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy