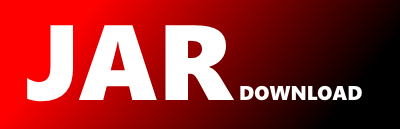
com.fasc.open.api.v5_1.client.SealClient Maven / Gradle / Ivy
The newest version!
package com.fasc.open.api.v5_1.client;
import com.fasc.open.api.bean.base.BaseRes;
import com.fasc.open.api.v5_1.req.seal.*;
import com.fasc.open.api.v5_1.req.template.CancelPersonalSealFreeSignReq;
import com.fasc.open.api.v5_1.req.template.CancelSealFreeSignReq;
import com.fasc.open.api.v5_1.res.seal.*;
import com.fasc.open.api.constants.OpenApiUrlConstants;
import com.fasc.open.api.exception.ApiException;
import java.util.List;
/**
* @author gongj
* @date 2022/7/12
*/
public class SealClient {
private OpenApiClient openApiClient;
public SealClient(OpenApiClient openApiClient) {
this.openApiClient = openApiClient;
}
public BaseRes createSealByTemplate(CreateSealByTemplateReq req) throws ApiException {
return openApiClient.invokeApi(req, OpenApiUrlConstants.SEAL_CREATE_BY_TEMPLATE, CreateSealByTemplateRes.class);
}
public BaseRes createSealByImage(CreateSealByImageReq req) throws ApiException {
return openApiClient.invokeApi(req, OpenApiUrlConstants.SEAL_CREATE_BY_IMAGE, CreateSealByImageRes.class);
}
public BaseRes createLegalRepresentativeSealByTemplate(CreateLegalRepresentativeSealByTemplateReq req) throws ApiException {
return openApiClient.invokeApi(req, OpenApiUrlConstants.SEAL_CREATE_LEGAL_REPRESENTATIVE_BY_TEMPLATE, CreateLegalRepresentativeSealByTemplateRes.class);
}
public BaseRes createLegalRepresentativeSealByImage(CreateLegalRepresentativeSealByImageReq req) throws ApiException {
return openApiClient.invokeApi(req, OpenApiUrlConstants.SEAL_CREATE_LEGAL_REPRESENTATIVE_BY_IMAGE, CreateLegalRepresentativeSealByImageRes.class);
}
public BaseRes getSealList(GetSealListReq req) throws ApiException {
return openApiClient.invokeApi(req, OpenApiUrlConstants.SEAL_GET_LIST, GetSealListRes.class);
}
public BaseRes getSealDetail(GetSealDetailReq req) throws ApiException {
return openApiClient.invokeApi(req, OpenApiUrlConstants.SEAL_GET_DETAIL, GetSealDetailRes.class);
}
public BaseRes getAppointedSealUrl(GetAppointedSealUrlReq req) throws ApiException {
return openApiClient.invokeApi(req, OpenApiUrlConstants.SEAL_GET_APPOINTED_URL, GetAppointedSealUrlRes.class);
}
public BaseRes getSealUserList(GetSealUserListReq req) throws ApiException {
return openApiClient.invokeApi(req, OpenApiUrlConstants.SEAL_GET_USER_LIST, GetSealUserListRes.class);
}
public BaseRes getUserSealList(GetUserSealListReq req) throws ApiException {
return openApiClient.invokeApi(req, OpenApiUrlConstants.GET_USER_SEAL_LIST, GetUserSealListRes.class);
}
public BaseRes getSealCreateUrl(GetSealCreateUrlReq req) throws ApiException {
return openApiClient.invokeApi(req, OpenApiUrlConstants.SEAL_GET_CREATE_URL, GetSealCreateUrlRes.class);
}
public BaseRes getSealVerifyList(GetSealVerifyListReq req) throws ApiException {
return openApiClient.invokeApi(req, OpenApiUrlConstants.SEAL_GET_VERIFY_LIST, GetSealVerifyListRes.class);
}
public BaseRes modifySeal(ModifySealReq req) throws ApiException {
return openApiClient.invokeApi(req, OpenApiUrlConstants.SEAL_MODIFY, Void.class);
}
public BaseRes getSealGrantUrl(GetSealGrantUrlReq req) throws ApiException {
return openApiClient.invokeApi(req, OpenApiUrlConstants.SEAL_GET_GRANT_URL, GetSealGrantUrlRes.class);
}
public BaseRes cancelSealGrant(CancelSealGrantReq req) throws ApiException {
return openApiClient.invokeApi(req, OpenApiUrlConstants.SEAL_CANCEL_GRANT, Void.class);
}
public BaseRes setSealStatus(SetSealStatusReq req) throws ApiException {
return openApiClient.invokeApi(req, OpenApiUrlConstants.SEAL_SET_STATUS, Void.class);
}
public BaseRes deleteSeal(SealDeleteReq req) throws ApiException {
return openApiClient.invokeApi(req, OpenApiUrlConstants.SEAL_DELETE, Void.class);
}
public BaseRes getSealManageUrl(GetSealManageUrlReq req) throws ApiException {
return openApiClient.invokeApi(req, OpenApiUrlConstants.SEAL_GET_MANAGE_URL, GetSealManageUrlRes.class);
}
public BaseRes getSealFreeSignUrl(GetSealFreeSignUrlReq req) throws ApiException {
return openApiClient.invokeApi(req, OpenApiUrlConstants.SEAL_GET_FREE_SIGN_URL, GetSealFreeSignUrlRes.class);
}
/**
* 获取印章授权模板免验证签链接
*/
public BaseRes getSealFreeSignToTemplateUrl(GetSealFreeSignToTemplateUrlReq req) throws ApiException {
return openApiClient.invokeApi(req, OpenApiUrlConstants.SEAL_FREE_SIGN_TO_TEMPLATE_URL, GetSealFreeSignToTemplateUrlRes.class);
}
/**
*查询授权模板免验证签的印章列表
*/
public BaseRes> getListSealFreeSignToTemplate(GetListSealFreeSignToTemplateReq req) throws ApiException {
return openApiClient.invokeApiList(req, OpenApiUrlConstants.SEAL_FREE_SIGN_TO_TEMPLATE_LIST_URL, GetListSealFreeSignToTemplateData.class);
}
public BaseRes createPersonalSealByTemplate(CreatePersonalSealByTemplateReq req) throws ApiException {
return openApiClient.invokeApi(req, OpenApiUrlConstants.PERSONAL_SEAL_CREATE_BY_TEMPLATE, CreatePersonalSealByTemplateRes.class);
}
public BaseRes createPersonalSealByImage(CreatePersonalSealByImageReq req) throws ApiException {
return openApiClient.invokeApi(req, OpenApiUrlConstants.PERSONAL_SEAL_CREATE_BY_IMAGE, CreatePersonalSealByImageRes.class);
}
public BaseRes getPersonalSealList(GetPersonalSealListReq req) throws ApiException {
return openApiClient.invokeApi(req, OpenApiUrlConstants.PERSONAL_SEAL_GET_LIST, GetPersonalSealListRes.class);
}
public BaseRes getPersonalSealFreeSignUrl(GetPersonalSealFreeSignUrlReq req) throws ApiException {
return openApiClient.invokeApi(req, OpenApiUrlConstants.PERSONAL_SEAL_GET_FREE_SIGN_URL, GetSealFreeSignUrlRes.class);
}
/**解除印章免验证签**/
public BaseRes cancelSealFreeSign(CancelSealFreeSignReq req) throws ApiException {
return openApiClient.invokeApi(req, OpenApiUrlConstants.CANCEL_SEAL_FREE_SIGN, Void.class);
}
/**解除签名免验证签**/
public BaseRes cancelPersonalSealFreeSign(CancelPersonalSealFreeSignReq req) throws ApiException {
return openApiClient.invokeApi(req, OpenApiUrlConstants.CANCEL_PERSONAL_SEAL_FREE_SIGN, Void.class);
}
/**获取签名管理链接**/
public BaseRes getPersonalSealManageUrl(GetPersonalSealManageUrlReq req) throws ApiException {
return openApiClient.invokeApi(req, OpenApiUrlConstants.PERSONAL_SEAL_GET_MANAGE_URL, GetPersonalSealManageUrlRes.class);
}
/**获取签名创建链接**/
public BaseRes getPersonalSealCreateUrl(GetPersonalSealCreateUrlReq req) throws ApiException {
return openApiClient.invokeApi(req, OpenApiUrlConstants.PERSONAL_SEAL_GET_CREATE_URL, GetPersonalSealCreateUrlRes.class);
}
/**删除个人签名**/
public BaseRes deletePersonalSeal(DeletePersonalSealReq req) throws ApiException {
return openApiClient.invokeApi(req, OpenApiUrlConstants.PERSONAL_SEAL_DELETE, Void.class);
}
/**查询印章标签列表**/
public BaseRes> getSealTagList(GetSealTagListReq req) throws ApiException {
return openApiClient.invokeApiList(req, OpenApiUrlConstants.SEAL_TAG_GET_LIST, String.class);
}
public BaseRes> getSealCertInfo(GetSeaCertInfoReq req) throws ApiException {
return openApiClient.invokeApiList(req, OpenApiUrlConstants.SEAL_GET_CERT_INFO, GetSeaCertInfoRes.class);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy