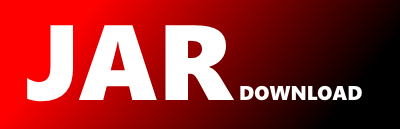
com.farao_community.farao.cse.data.ntc.Ntc Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of gridcapa-cse-data Show documentation
Show all versions of gridcapa-cse-data Show documentation
Module that manages specific data format for CSE processes
The newest version!
/*
* Copyright (c) 2023, RTE (http://www.rte-france.com)
* This Source Code Form is subject to the terms of the Mozilla Public
* License, v. 2.0. If a copy of the MPL was not distributed with this
* file, You can obtain one at http://mozilla.org/MPL/2.0/.
*/
package com.farao_community.farao.cse.data.ntc;
import com.farao_community.farao.cse.data.xsd.TLine;
import java.util.HashMap;
import java.util.Map;
import java.util.Optional;
import java.util.function.Predicate;
import java.util.stream.Collectors;
public final class Ntc {
private final YearlyNtcDocument yearlyNtcDocument;
private final DailyNtcDocument dailyNtcDocument;
private final YearlyNtcDocumentAdapted yearlyNtcDocumentAdapted;
private final DailyNtcDocumentAdapted dailyNtcDocumentAdapted;
private final boolean isImportEcProcess;
public Ntc(YearlyNtcDocument yearlyNtcDocument, DailyNtcDocument dailyNtcDocument, boolean isImportEcProcess) {
this.yearlyNtcDocument = yearlyNtcDocument;
this.dailyNtcDocument = dailyNtcDocument;
this.yearlyNtcDocumentAdapted = null;
this.dailyNtcDocumentAdapted = null;
this.isImportEcProcess = isImportEcProcess;
}
public Ntc(YearlyNtcDocumentAdapted yearlyNtcDocumentAdapted, DailyNtcDocumentAdapted dailyNtcDocumentAdapted, boolean isImportEcProcess) {
this.yearlyNtcDocument = null;
this.dailyNtcDocument = null;
this.yearlyNtcDocumentAdapted = yearlyNtcDocumentAdapted;
this.dailyNtcDocumentAdapted = dailyNtcDocumentAdapted;
this.isImportEcProcess = isImportEcProcess;
}
public Double computeMniiOffset() {
Map flowOnNotModeledLinesPerCountry = isImportEcProcess ?
getFlowPerCountryAdapted(Predicate.not(com.farao_community.farao.cse.data.xsd.ntc_adapted.TLine::isModelized)) :
getFlowPerCountry(Predicate.not(TLine::isModelized));
return flowOnNotModeledLinesPerCountry.values().stream().reduce(0., Double::sum);
}
public Map getFlowPerCountryOnMerchantLines() {
return isImportEcProcess ?
getFlowPerCountryAdapted(com.farao_community.farao.cse.data.xsd.ntc_adapted.TLine::isMerchantLine) :
getFlowPerCountry(TLine::isMerchantLine);
}
public Map computeReducedSplittingFactors() {
Map ntcsByCountry = getNtcPerCountry();
Map flowOnMerchantLinesPerCountry = getFlowPerCountryOnMerchantLines();
Double totalNtc = ntcsByCountry.values().stream().reduce(0., Double::sum);
Double totalFlowOnMerchantLines = flowOnMerchantLinesPerCountry.values().stream().reduce(0., Double::sum);
return getReducedSplittingFactors(ntcsByCountry, flowOnMerchantLinesPerCountry, totalNtc, totalFlowOnMerchantLines);
}
public Map getFlowOnFixedFlowLines() {
if (isImportEcProcess) {
Predicate fixedFlowLines = tLine -> tLine.isFixedFlow() && tLine.isModelized();
Map yearlyLineInformationPerLineId = yearlyNtcDocumentAdapted.getLineInformationPerLineId(fixedFlowLines);
Map dailyLineInformationPerLineId = dailyNtcDocumentAdapted != null ? dailyNtcDocumentAdapted.getLineInformationPerLineId(fixedFlowLines) : Map.of();
return getFlowPerLineId(yearlyLineInformationPerLineId, dailyLineInformationPerLineId);
} else {
Predicate fixedFlowLines = tLine -> tLine.isFixedFlow() && tLine.isModelized();
Map yearlyLineInformationPerLineId = yearlyNtcDocument.getLineInformationPerLineId(fixedFlowLines);
Map dailyLineInformationPerLineId = dailyNtcDocument != null ? dailyNtcDocument.getLineInformationPerLineId(fixedFlowLines) : Map.of();
return getFlowPerLineId(yearlyLineInformationPerLineId, dailyLineInformationPerLineId);
}
}
public Map getFlowPerCountryOnNotModelizedLines() {
return isImportEcProcess ?
getFlowPerCountryAdapted(t -> !t.isModelized()) :
getFlowPerCountry(t -> !t.isModelized());
}
Map getFlowPerCountry(Predicate lineSelector) {
Map yearlyLineInformationPerLineId = yearlyNtcDocument.getLineInformationPerLineId(lineSelector);
Map dailyLineInformationPerLineId = dailyNtcDocument != null ? dailyNtcDocument.getLineInformationPerLineId(lineSelector) : Map.of();
Map flowPerLineId = getFlowPerLineId(yearlyLineInformationPerLineId, dailyLineInformationPerLineId);
Map flowPerCountry = new HashMap<>();
flowPerLineId.forEach((lineId, flow) -> {
String country = Optional.ofNullable(yearlyLineInformationPerLineId.get(lineId))
.map(LineInformation::getCountry)
.orElseGet(() -> dailyLineInformationPerLineId.get(lineId).getCountry());
double initialFlow = Optional.ofNullable(flowPerCountry.get(country)).orElse(0.);
flowPerCountry.put(country, initialFlow + flow);
});
return flowPerCountry;
}
Map getFlowPerCountryAdapted(Predicate lineSelector) {
Map yearlyLineInformationPerLineId = yearlyNtcDocumentAdapted.getLineInformationPerLineId(lineSelector);
Map dailyLineInformationPerLineId = dailyNtcDocumentAdapted != null ? dailyNtcDocumentAdapted.getLineInformationPerLineId(lineSelector) : Map.of();
Map flowPerLineId = getFlowPerLineId(yearlyLineInformationPerLineId, dailyLineInformationPerLineId);
Map flowPerCountry = new HashMap<>();
flowPerLineId.forEach((lineId, flow) -> {
String country = Optional.ofNullable(yearlyLineInformationPerLineId.get(lineId))
.map(LineInformation::getCountry)
.orElseGet(() -> dailyLineInformationPerLineId.get(lineId).getCountry());
double initialFlow = Optional.ofNullable(flowPerCountry.get(country)).orElse(0.);
flowPerCountry.put(country, initialFlow + flow);
});
return flowPerCountry;
}
public Map getNtcPerCountry() {
return isImportEcProcess ? getNtcPerCountryAdapted() : getNtcPerCountryNotAdapted();
}
private Map getNtcPerCountryNotAdapted() {
Map ntcPerCountry = yearlyNtcDocument.getNtcInformationPerCountry().entrySet().stream()
.collect(Collectors.toMap(
Map.Entry::getKey,
entry -> entry.getValue().getFlow()
));
if (dailyNtcDocument != null) {
dailyNtcDocument.getNtcInformationPerCountry().forEach((country, ntcInformation) -> {
if (ntcInformation.getVariationType().equalsIgnoreCase(NtcUtil.ABSOLUTE)) {
ntcPerCountry.put(country, ntcInformation.getFlow());
} else {
ntcPerCountry.put(country, ntcPerCountry.get(country) + ntcInformation.getFlow());
}
});
}
return ntcPerCountry;
}
private Map getNtcPerCountryAdapted() {
Map ntcPerCountry = yearlyNtcDocumentAdapted.getNtcInformationPerCountry().entrySet().stream()
.collect(Collectors.toMap(
Map.Entry::getKey,
entry -> entry.getValue().getFlow()
));
if (dailyNtcDocumentAdapted != null) {
dailyNtcDocumentAdapted.getNtcInformationPerCountry().forEach((country, ntcInformation) -> {
if (ntcInformation.getVariationType().equalsIgnoreCase(NtcUtilAdapted.ABSOLUTE)) {
ntcPerCountry.put(country, ntcInformation.getFlow());
} else {
ntcPerCountry.put(country, ntcPerCountry.get(country) + ntcInformation.getFlow());
}
});
}
return ntcPerCountry;
}
private static Map getFlowPerLineId(Map yearlyLinePerId, Map dailyLinePerId) {
Map flowPerLine = yearlyLinePerId.entrySet().stream()
.collect(Collectors.toMap(
Map.Entry::getKey,
entry -> entry.getValue().getFlow()
));
dailyLinePerId.forEach((lineId, lineInformation) -> {
if (lineInformation.getVariationType().equalsIgnoreCase(NtcUtil.ABSOLUTE)) {
flowPerLine.put(lineId, lineInformation.getFlow());
} else {
double initialFlow = Optional.ofNullable(flowPerLine.get(lineId)).orElse(0.);
flowPerLine.put(lineId, initialFlow + lineInformation.getFlow());
}
});
return flowPerLine;
}
private static Map getReducedSplittingFactors(Map ntcPerCountry,
Map flowOnMerchantLinesPerCountry,
Double totalNtc,
Double totalFlowOnMerchantLines) {
return ntcPerCountry.entrySet().stream()
.collect(Collectors.toMap(
Map.Entry::getKey,
entry -> {
Double flowOnMerchantLines = Optional
.ofNullable(flowOnMerchantLinesPerCountry.get(entry.getKey()))
.orElse(0.);
return (entry.getValue() - flowOnMerchantLines) / (totalNtc - totalFlowOnMerchantLines);
}));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy