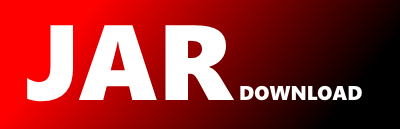
com.farao_community.farao.cse.data.target_ch.TargetChDocument Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of gridcapa-cse-data Show documentation
Show all versions of gridcapa-cse-data Show documentation
Module that manages specific data format for CSE processes
The newest version!
/*
* Copyright (c) 2021, RTE (http://www.rte-france.com)
* This Source Code Form is subject to the terms of the Mozilla Public
* License, v. 2.0. If a copy of the MPL was not distributed with this
* file, You can obtain one at http://mozilla.org/MPL/2.0/.
*/
package com.farao_community.farao.cse.data.target_ch;
import com.farao_community.farao.cse.data.DataUtil;
import com.farao_community.farao.cse.data.xsd.target_ch.*;
import javax.xml.bind.JAXBException;
import java.io.InputStream;
import java.time.OffsetDateTime;
import java.util.*;
import static com.farao_community.farao.cse.data.DataUtil.toOptional;
final class TargetChDocument {
private final TargetsDocument targetsDocument;
static TargetChDocument create(InputStream targetChInputStream) throws JAXBException {
return new TargetChDocument(DataUtil.unmarshalFromInputStream(targetChInputStream, TargetsDocument.class));
}
private TargetChDocument(TargetsDocument targetsDocument) {
this.targetsDocument = targetsDocument;
}
Map> getOutagesInformationPerLineId(OffsetDateTime targetDateTime) {
Map> outagesInformationPerLineId = new HashMap<>();
Set lines = getLines(targetsDocument);
lines.forEach(line -> addOutagesInformation(outagesInformationPerLineId, targetDateTime, line));
return outagesInformationPerLineId;
}
private static Set getLines(TargetsDocument targetsDocument) {
List tTargetData = targetsDocument.getTargetData();
Set lines = new HashSet<>();
tTargetData.forEach(data -> {
TSpecialLines tSpecialLines = data.getSpecialLines();
if (tSpecialLines != null && tSpecialLines.getLine() != null) {
lines.add(tSpecialLines.getLine());
}
});
return lines;
}
private void addOutagesInformation(Map> outagesInformationPerLineId, OffsetDateTime targetDateTime, TLine tLine) {
List outagesInformation = new ArrayList<>();
TargetChUtil.getMatchingPeriod(targetDateTime, tLine.getPeriod())
.map(TPeriod::getOutages)
.ifPresent(tOutages -> tOutages.getOutage().forEach(tOutage ->
addOutageInformation(outagesInformation, targetDateTime, tOutage)));
outagesInformationPerLineId.put(tLine.getCode(), outagesInformation);
}
private void addOutageInformation(List outagesInformation, OffsetDateTime targetDateTime, TOutage tOutage) {
TargetChUtil.getFixedFlowFromDaysOfWeek(targetDateTime, tOutage.getDayOfWeek()).stream()
.collect(toOptional())
.ifPresent(fixedFlow -> outagesInformation.add(new OutageInformation(tOutage, fixedFlow)));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy