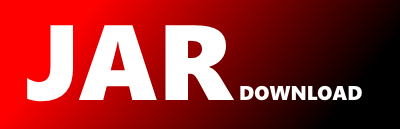
com.farao_community.farao.cse.data.xsd.TLine Maven / Gradle / Ivy
Show all versions of gridcapa-cse-data Show documentation
//
// This file was generated by the JavaTM Architecture for XML Binding(JAXB) Reference Implementation, v2.3.0
// See https://javaee.github.io/jaxb-v2/
// Any modifications to this file will be lost upon recompilation of the source schema.
// Generated on: 2024.12.17 at 02:20:10 PM UTC
//
package com.farao_community.farao.cse.data.xsd;
import java.util.ArrayList;
import java.util.List;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlAttribute;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlType;
/**
* Java class for T_Line complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType name="T_Line">
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="Period" type="{}T_Period" maxOccurs="unbounded" minOccurs="0"/>
* </sequence>
* <attribute name="name" use="required" type="{http://www.w3.org/2001/XMLSchema}string" />
* <attribute name="n_to" use="required" type="{}T_CountryList" />
* <attribute name="n_from" use="required" type="{}T_CountryList" />
* <attribute name="modelized" use="required" type="{http://www.w3.org/2001/XMLSchema}boolean" />
* <attribute name="fixed_flow" use="required" type="{http://www.w3.org/2001/XMLSchema}boolean" />
* <attribute name="code" use="required" type="{http://www.w3.org/2001/XMLSchema}string" />
* <attribute name="c_ntc" use="required" type="{}T_CountryList" />
* <attribute name="merchant_line" use="required" type="{http://www.w3.org/2001/XMLSchema}boolean" />
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "T_Line", namespace = "", propOrder = {
"period"
})
public class TLine {
@XmlElement(name = "Period")
protected List period;
@XmlAttribute(name = "name", required = true)
protected String name;
@XmlAttribute(name = "n_to", required = true)
protected TCountryList nTo;
@XmlAttribute(name = "n_from", required = true)
protected TCountryList nFrom;
@XmlAttribute(name = "modelized", required = true)
protected boolean modelized;
@XmlAttribute(name = "fixed_flow", required = true)
protected boolean fixedFlow;
@XmlAttribute(name = "code", required = true)
protected String code;
@XmlAttribute(name = "c_ntc", required = true)
protected TCountryList cNtc;
@XmlAttribute(name = "merchant_line", required = true)
protected boolean merchantLine;
/**
* Gets the value of the period property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the period property.
*
*
* For example, to add a new item, do as follows:
*
* getPeriod().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link TPeriod }
*
*
*/
public List getPeriod() {
if (period == null) {
period = new ArrayList();
}
return this.period;
}
/**
* Gets the value of the name property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getName() {
return name;
}
/**
* Sets the value of the name property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setName(String value) {
this.name = value;
}
/**
* Gets the value of the nTo property.
*
* @return
* possible object is
* {@link TCountryList }
*
*/
public TCountryList getNTo() {
return nTo;
}
/**
* Sets the value of the nTo property.
*
* @param value
* allowed object is
* {@link TCountryList }
*
*/
public void setNTo(TCountryList value) {
this.nTo = value;
}
/**
* Gets the value of the nFrom property.
*
* @return
* possible object is
* {@link TCountryList }
*
*/
public TCountryList getNFrom() {
return nFrom;
}
/**
* Sets the value of the nFrom property.
*
* @param value
* allowed object is
* {@link TCountryList }
*
*/
public void setNFrom(TCountryList value) {
this.nFrom = value;
}
/**
* Gets the value of the modelized property.
*
*/
public boolean isModelized() {
return modelized;
}
/**
* Sets the value of the modelized property.
*
*/
public void setModelized(boolean value) {
this.modelized = value;
}
/**
* Gets the value of the fixedFlow property.
*
*/
public boolean isFixedFlow() {
return fixedFlow;
}
/**
* Sets the value of the fixedFlow property.
*
*/
public void setFixedFlow(boolean value) {
this.fixedFlow = value;
}
/**
* Gets the value of the code property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getCode() {
return code;
}
/**
* Sets the value of the code property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setCode(String value) {
this.code = value;
}
/**
* Gets the value of the cNtc property.
*
* @return
* possible object is
* {@link TCountryList }
*
*/
public TCountryList getCNtc() {
return cNtc;
}
/**
* Sets the value of the cNtc property.
*
* @param value
* allowed object is
* {@link TCountryList }
*
*/
public void setCNtc(TCountryList value) {
this.cNtc = value;
}
/**
* Gets the value of the merchantLine property.
*
*/
public boolean isMerchantLine() {
return merchantLine;
}
/**
* Sets the value of the merchantLine property.
*
*/
public void setMerchantLine(boolean value) {
this.merchantLine = value;
}
}