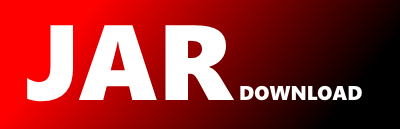
com.fastchar.extjs.core.FastExtEntities Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of fastchar-extjs Show documentation
Show all versions of fastchar-extjs Show documentation
FastChar-ExtJs is a Java Web framework that uses extjs libraries.Quickly build a background management system
package com.fastchar.extjs.core;
import com.fastchar.annotation.AFastPriority;
import com.fastchar.core.FastChar;
import com.fastchar.core.FastEntity;
import com.fastchar.database.info.FastColumnInfo;
import com.fastchar.database.info.FastTableInfo;
import com.fastchar.extjs.FastExtConfig;
import com.fastchar.extjs.core.database.FastExtColumnInfo;
import com.fastchar.extjs.core.database.FastExtTableInfo;
import com.fastchar.extjs.core.database.FastExtTableSplit;
import com.fastchar.extjs.exception.FastExtEntityException;
import com.fastchar.interfaces.IFastMethodRead;
import com.fastchar.utils.FastClassUtils;
import com.fastchar.utils.FastStringUtils;
import java.util.*;
public class FastExtEntities {
private final Map>> entityMap = new HashMap<>();
public FastExtEntities addEntity(Class extends FastExtEntity>> targetClass) throws Exception {
if (!FastExtEntity.class.isAssignableFrom(targetClass)) {
FastChar.getLogger().warn(this.getClass(), FastChar.getLocal().getInfo("ExtEntity_Error1", targetClass));
return this;
}
if (!FastClassUtils.checkNewInstance(targetClass)) {
return this;
}
FastExtEntity> fastExtEntity = FastClassUtils.newInstance(targetClass);
if (fastExtEntity != null) {
//跳过无效的Entity
if (!fastExtEntity.isValidExtEntity()) {
return this;
}
String entityCode = fastExtEntity.getEntityCode();
if (FastStringUtils.isEmpty(entityCode)) {
return this;
}
if (entityMap.containsKey(entityCode)) {
if (FastClassUtils.isSameClass(targetClass, entityMap.get(entityCode))) {
entityMap.put(entityCode, targetClass);
return this;
}
int existPriority = 0;
int currPriority = 0;
IFastMethodRead fastMethodRead = FastChar.getOverrides().newInstance(IFastMethodRead.class);
Class extends FastExtEntity>> existClass = entityMap.get(entityCode);
if (existClass.equals(targetClass)) {
FastChar.getLogger().warn(this.getClass(), FastChar.getLocal().getInfo("ExtEntity_Error4", targetClass));
return this;
}
List existLineNumber = fastMethodRead.getMethodLineNumber(existClass, "getEntityCode");
List targetLineNumber = fastMethodRead.getMethodLineNumber(targetClass, "getEntityCode");
StackTraceElement existStack = new StackTraceElement(existClass.getName(), "getEntityCode",
existClass.getSimpleName() + ".java", existLineNumber.get(0).getLastLine());
StackTraceElement currStack = new StackTraceElement(targetClass.getName(), "getEntityCode",
targetClass.getSimpleName() + ".java", targetLineNumber.get(0).getLastLine());
if (existClass.isAnnotationPresent(AFastPriority.class)) {
AFastPriority aFastPriority = existClass.getAnnotation(AFastPriority.class);
existPriority = aFastPriority.value();
}
if (targetClass.isAnnotationPresent(AFastPriority.class)) {
AFastPriority aFastPriority = targetClass.getAnnotation(AFastPriority.class);
currPriority = aFastPriority.value();
}
if (currPriority == existPriority) {
throw new FastExtEntityException("the entity code '" + entityCode + "' already exists!" +
"\n\tat " + existStack +
"\n\tat " + currStack);
} else if (currPriority < existPriority) {
return this;
}
}
entityMap.put(entityCode, targetClass);
}
return this;
}
public Class extends FastExtEntity>> getExtEntity(String entityCode) {
return entityMap.get(entityCode);
}
public List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy