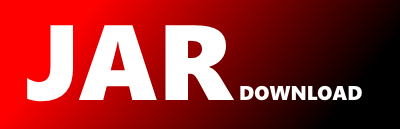
com.fastchar.extjs.core.configjson.FastExtConfigJson Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of fastchar-extjs Show documentation
Show all versions of fastchar-extjs Show documentation
FastChar-ExtJs is a Java Web framework that uses extjs libraries.Quickly build a background management system
package com.fastchar.extjs.core.configjson;
import com.fastchar.core.FastChar;
import com.fastchar.core.FastMapWrap;
import com.fastchar.core.FastResource;
import com.fastchar.extjs.FastExtConfig;
import com.fastchar.utils.FastFileUtils;
import com.fastchar.utils.FastNumberUtils;
import com.fastchar.utils.FastStringUtils;
import com.google.gson.JsonObject;
import java.io.IOException;
import java.nio.charset.StandardCharsets;
import java.util.ArrayList;
import java.util.Comparator;
import java.util.List;
import java.util.Map;
public class FastExtConfigJson {
public static FastExtConfigJson getInstance() {
return FastChar.getOverrides().singleInstance(FastExtConfigJson.class);
}
private final List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy