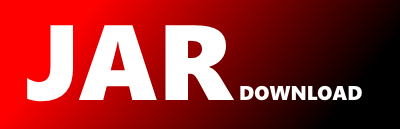
com.fastchar.extjs.entity.ExtSystemDataEntity Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of fastchar-extjs Show documentation
Show all versions of fastchar-extjs Show documentation
FastChar-ExtJs is a Java Web framework that uses extjs libraries.Quickly build a background management system
package com.fastchar.extjs.entity;
import com.fastchar.core.FastChar;
import com.fastchar.database.FastPage;
import com.fastchar.database.info.FastSqlInfo;
import com.fastchar.extjs.core.FastExtEntity;
import com.fastchar.extjs.databoard.FastDataboardDataInfo;
import com.fastchar.extjs.databoard.FastDataboardDataTextInfo;
import com.fastchar.extjs.databoard.IFastDataboardData;
import com.fastchar.utils.FastDateUtils;
import com.fastchar.utils.FastMD5Utils;
import java.util.ArrayList;
import java.util.Calendar;
import java.util.Date;
import java.util.List;
public class ExtSystemDataEntity extends FastExtEntity {
private static final long serialVersionUID = 1L;
public static ExtSystemDataEntity dao() {
return FastChar.getOverrides().singleInstance(ExtSystemDataEntity.class);
}
public static ExtSystemDataEntity newInstance() {
return FastChar.getOverrides().newInstance(ExtSystemDataEntity.class);
}
@Override
public String getTableName() {
return "ext_system_data";
}
@Override
public FastPage showList(int page, int pageSize) {
String sqlStr = "select t.* from ext_system_data as t " +
" where 1=1 ";
FastSqlInfo sqlInfo = toSelectSql(sqlStr);
return selectBySql(page, pageSize, sqlInfo.getSql(), sqlInfo.toParams());
}
@Override
public void setDefaultValue() {
}
/**
* 保存指定日期的数据统计选项值
* @param dateRang 日期区间
*/
public void saveYesterdayDataboardTextInfo(int dateRang) {
Calendar instance = Calendar.getInstance();
for (int i = 0; i < dateRang; i++) {
instance.add(Calendar.DATE, -1);
List select = ExtManagerEntity.dao().select();
for (ExtManagerEntity extManagerEntity : select) {
ExtSystemDataEntity.dao().saveData(extManagerEntity, instance.getTime());
}
}
}
private void saveData(ExtManagerEntity managerEntity, Date date) {
if (managerEntity == null) {
return;
}
List> data = getData(managerEntity, date);
saveData(managerEntity, date, data);
}
private void saveData(ExtManagerEntity managerEntity, Date date, List> data) {
for (FastDataboardDataInfo> fastDataInfo : data) {
if (fastDataInfo instanceof FastDataboardDataTextInfo) {
FastDataboardDataTextInfo textInfo = (FastDataboardDataTextInfo) fastDataInfo;
ExtSystemDataEntity dataEntity = ExtSystemDataEntity.newInstance();
dataEntity.set("managerId", managerEntity.getId());
dataEntity.set("dataCode", FastMD5Utils.MD5To16(managerEntity.getId() +
textInfo.getId() + FastDateUtils.format(date, "yyyy-MM-dd")));
dataEntity.set("dataType", textInfo.getId());
dataEntity.set("dataValue", fastDataInfo.getValue());
dataEntity.set("dataDateTime", FastDateUtils.format(date, "yyyy-MM-dd HH:mm:ss"));
dataEntity.push("dataCode");
}
}
}
private List> getData(ExtManagerEntity managerEntity, Date date) {
String dateFormat = FastDateUtils.format(date, "yyyy-MM-dd");
Date begin = FastDateUtils.parse(dateFormat + " 00:00:00", "yyyy-MM-dd HH:mm:ss");
Date end = FastDateUtils.parse(dateFormat + " 23:59:59", "yyyy-MM-dd HH:mm:ss");
List> allInfos = new ArrayList<>();
List iFastDataBoards = FastChar.getOverrides().newInstances(false, IFastDataboardData.class);
for (IFastDataboardData iFastDataBoard : iFastDataBoards) {
List> dataInfo = iFastDataBoard.getDataInfo(managerEntity, null, begin, end);
if (dataInfo == null) {
continue;
}
allInfos.addAll(dataInfo);
}
return allInfos;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy