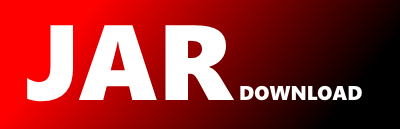
com.fastchar.server.undertow.FastServerUndertow Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of fastchar-server-undertow Show documentation
Show all versions of fastchar-server-undertow Show documentation
FastChar-Server-Undertow is server container for undertow.
package com.fastchar.server.undertow;
import com.fastchar.core.FastChar;
import com.fastchar.server.ServerStartHandler;
import com.fastchar.servlet.FastJavaxServletContainerInitializer;
import io.undertow.Undertow;
import io.undertow.server.HttpHandler;
import io.undertow.server.session.SessionManager;
import io.undertow.servlet.Servlets;
import io.undertow.servlet.api.DeploymentInfo;
import io.undertow.servlet.api.DeploymentManager;
import io.undertow.servlet.api.ServletContainerInitializerInfo;
import io.undertow.servlet.api.ServletStackTraces;
import io.undertow.servlet.handlers.DefaultServlet;
import javax.servlet.ServletException;
import java.io.File;
import java.util.ArrayList;
import java.util.Collections;
import java.util.List;
public class FastServerUndertow {
public static FastServerUndertow getInstance() {
return new FastServerUndertow().initServer();
}
private FastServerUndertow() {
}
private Undertow undertow;
private DeploymentManager deploymentManager;
private final ServerStartHandler starterHandler = new ServerStartHandler();
private FastServerUndertow initServer() {
starterHandler.setStartRunnable(() -> {
if (undertow != null) {
undertow.start();
}
}).setStopRunnable(this::stop);
return this;
}
public synchronized void start(FastUndertowConfig undertowConfig) {
try {
if (undertow != null) {
return;
}
DeploymentInfo deployment = Servlets.deployment();
ServletContainerInitializerInfo servletContainerInitializerInfo = new ServletContainerInitializerInfo(
FastJavaxServletContainerInitializer.class
, Collections.emptySet());
deployment.addServletContainerInitializer(servletContainerInitializerInfo);
deployment.setContextPath(undertowConfig.getContextPath());
deployment.setClassLoader(FastServerUndertow.class.getClassLoader());
deployment.setServletStackTraces(ServletStackTraces.NONE);
deployment.setDefaultRequestEncoding("utf-8");
deployment.setDefaultResponseEncoding("utf-8");
deployment.setDefaultSessionTimeout(FastChar.getConstant().getSessionMaxInterval());
deployment.setDefaultEncoding("utf-8");
deployment.setDeploymentName("fastchar-server");
deployment.setTempDir(new File(undertowConfig.getDocBase(), "/undertow_temp"));
deployment.setEagerFilterInit(undertowConfig.isEagerFilterInit());
deployment.setPreservePathOnForward(undertowConfig.isPreservePathOnForward());
deployment.addServlet(Servlets.servlet("default", DefaultServlet.class));
undertowConfig.configureResource(deployment);
undertowConfig.configureWebListeners(deployment);
undertowConfig.configureSession(deployment);
deploymentManager = Servlets.newContainer().addDeployment(deployment);
deploymentManager.deploy();
SessionManager sessionManager = deploymentManager.getDeployment().getSessionManager();
sessionManager.setDefaultSessionTimeout(FastChar.getConstant().getSessionMaxInterval());
List handlers = new ArrayList<>();
handlers.add(deploymentManager.start());
handlers.add(new CookieSameSiteHandler(undertowConfig));
handlers.addAll(undertowConfig.getHandlers());
undertow = undertowConfig.getBuilder().setHandler(new HttpHandlerCollection(handlers)).build();
this.starterHandler
.setPort(undertowConfig.getPort())
.setHost(undertowConfig.getHost())
.setContextPath(undertowConfig.getContextPath())
.start();
} catch (Exception e) {
e.printStackTrace();
stop();
}
}
public synchronized void stop() {
try {
if (this.deploymentManager != null) {
this.deploymentManager.stop();
this.deploymentManager.undeploy();
this.deploymentManager = null;
}
if (this.undertow != null) {
this.undertow.stop();
}
} catch (ServletException e) {
throw new RuntimeException(e);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy