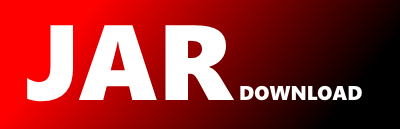
com.fastchar.slf4j.log4j2.provider.Log4jProvider Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of fastchar-slf4j Show documentation
Show all versions of fastchar-slf4j Show documentation
FastChar-SLF4J is a FastChar plugin.
package com.fastchar.slf4j.log4j2.provider;
import com.fastchar.annotation.AFastObserver;
import com.fastchar.core.FastChar;
import com.fastchar.core.FastLogger;
import com.fastchar.interfaces.IFastLog;
import com.fastchar.slf4j.FastLog4j2Config;
import com.fastchar.utils.FastFileUtils;
import com.fastchar.utils.FastStringUtils;
import org.apache.logging.log4j.LogManager;
import org.apache.logging.log4j.core.LoggerContext;
import org.slf4j.LoggerFactory;
import java.io.File;
import java.io.IOException;
import java.net.URL;
import java.nio.charset.StandardCharsets;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
/**
* @author 沈建(Janesen)
* @date 2021/12/25 14:53
*/
@AFastObserver
public class Log4jProvider implements IFastLog {
public synchronized void onWebReady() throws Exception {
initConfig();
}
public void initConfig() {
try {
FastLog4j2Config config = FastChar.getConfig(FastLog4j2Config.class);
if (config.isEnable()) {
File log4jConfigFile = new File(FastChar.getPath().getClassRootPath(), "fast-log4j2.xml");
if (!log4jConfigFile.exists()) {
Map params = new HashMap<>();
params.put("configRootLevel", config.getRootLevel().name());
params.put("configLogDirectory", config.getLogDirectory());
params.put("configAppenders", buildAppenders());
params.put("configLoggers", buildLoggers());
FastFileUtils.writeStringToFile(log4jConfigFile, getTemplateContent("template_log4j2.xml", params));
FastChar.getLog().info("已自动生成fast-log4j2.xml配置文件!");
}
LoggerContext context = (LoggerContext) LogManager.getContext(false);
context.setConfigLocation(log4jConfigFile.toURI());
FastChar.getLog().info("已加载fast-log4j2.xml配置文件!");
}
} catch (IOException e) {
e.printStackTrace();
}
}
private String buildAppenders() {
List appenders = new ArrayList<>();
FastLog4j2Config config = FastChar.getConfig(FastLog4j2Config.class);
for (Log4jSplitInfo logSplit : config.getLogSplits()) {
Map params = new HashMap<>();
params.put("configType", logSplit.getFileName());
params.put("configSize", String.valueOf(logSplit.getLogFileMaxSize()));
appenders.add(getTemplateContent("template_rollingfile.xml", params));
}
return FastStringUtils.join(appenders, "\n");
}
private String buildLoggers() {
FastLog4j2Config config = FastChar.getConfig(FastLog4j2Config.class);
List loggers = new ArrayList<>();
for (Log4jSplitInfo logSplit : config.getLogSplits()) {
Map params = new HashMap<>();
params.put("configType", logSplit.getFileName());
params.put("configTypePackageName", logSplit.getLogPattern());
params.put("configTypeLevel", logSplit.getLevel());
loggers.add(getTemplateContent("template_loggers.xml", params));
}
return FastStringUtils.join(loggers, "\n");
}
private String getTemplateContent(String fileName, Map params) {
try {
URL url = Log4jProvider.class.getResource(fileName);
if (url != null) {
List strings = FastFileUtils.readLines(url.openStream(), StandardCharsets.UTF_8);
String fileContent = FastStringUtils.join(strings, "\n");
for (String key : params.keySet()) {
fileContent = fileContent.replace("{{" + key + "}}", params.get(key));
}
return fileContent;
}
} catch (IOException e) {
e.printStackTrace();
}
return "";
}
private StackTraceElement findCaller() {
List baseClassNames = new ArrayList<>();
baseClassNames.add(Thread.class.getName());
baseClassNames.add(Log4jProvider.class.getName());
baseClassNames.add(FastLogger.class.getName());
StackTraceElement[] stackTrace = Thread.currentThread().getStackTrace();
for (StackTraceElement stackTraceElement : stackTrace) {
if (baseClassNames.contains(stackTraceElement.getClassName())) {
continue;
}
return stackTraceElement;
}
return new StackTraceElement(Log4jProvider.class.getName(), "", "", 0);
}
private void logMsg(String method, Class> targetClass, String message, Throwable throwable) {
StackTraceElement caller = findCaller();
String loggerName = caller.getClassName();
if (targetClass != null) {
loggerName = targetClass.getName();
}
if (loggerName.equals(caller.getClassName())) {
loggerName = loggerName + " - line:" + caller.getLineNumber();
} else {
loggerName = loggerName + " - [ " + caller.getClassName() + " - line:" + caller.getLineNumber() + " ] ";
}
if (method.equalsIgnoreCase("debug")) {
LoggerFactory.getLogger(loggerName).debug(message, throwable);
} else if (method.equalsIgnoreCase("info")) {
LoggerFactory.getLogger(loggerName).info(message, throwable);
} else if (method.equalsIgnoreCase("warn")) {
LoggerFactory.getLogger(loggerName).warn(message, throwable);
} else if (method.equalsIgnoreCase("error")) {
LoggerFactory.getLogger(loggerName).error(message, throwable);
}
}
@Override
public void debug(Class> targetClass, String message, Throwable throwable) {
logMsg("debug", targetClass, message, throwable);
}
@Override
public void info(Class> targetClass, String message, Throwable throwable) {
logMsg("info", targetClass, message, throwable);
}
@Override
public void error(Class> targetClass, String message, Throwable throwable) {
logMsg("error", targetClass, message, throwable);
}
@Override
public void warn(Class> targetClass, String message, Throwable throwable) {
logMsg("warn", targetClass, message, throwable);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy