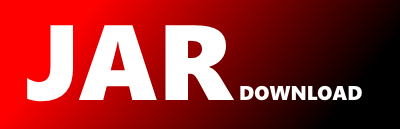
com.fastchar.database.FastDb Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of fastchar Show documentation
Show all versions of fastchar Show documentation
FastChar is Web+ORM Framework in Java.
package com.fastchar.database;
import com.fastchar.core.FastChar;
import com.fastchar.core.FastEntity;
import com.fastchar.database.info.FastDatabaseInfo;
import com.fastchar.database.info.FastSqlInfo;
import com.fastchar.database.sql.FastSql;
import com.fastchar.interfaces.IFastCache;
import com.fastchar.utils.FastArrayUtils;
import com.fastchar.utils.FastDateUtils;
import com.fastchar.utils.FastNumberUtils;
import com.fastchar.utils.FastStringUtils;
import javax.sql.DataSource;
import java.sql.*;
import java.util.*;
/**
* 数据库sql操作
*/
public class FastDb {
private static final ThreadLocal TRANSACTION_THREAD_LOCAL = new ThreadLocal();
public static boolean isTransaction() {
FastTransaction fastTransaction = TRANSACTION_THREAD_LOCAL.get();
return fastTransaction != null && fastTransaction.isValid();
}
public static FastTransaction doTransaction() {
FastTransaction fastTransaction = new FastTransaction();
TRANSACTION_THREAD_LOCAL.set(fastTransaction);
return fastTransaction;
}
private boolean log = true;
private boolean useCache = true;
private String database;
private long inTime;
private FastDatabaseInfo getDatabaseInfo() {
return FastChar.getDatabases().get(database);
}
public Connection getConnection() throws SQLException {
FastDatabaseInfo databaseInfo = getDatabaseInfo();
DataSource dataSource = databaseInfo.getDataSource();
if (dataSource == null) {
return null;
}
FastTransaction fastTransaction = TRANSACTION_THREAD_LOCAL.get();
if (fastTransaction != null) {
if (fastTransaction.isValid()) {
if (!fastTransaction.contains(databaseInfo.getName())) {
fastTransaction.setConnection(databaseInfo.getName(), dataSource.getConnection());
}
return fastTransaction.getConnection(databaseInfo.getName());
}
TRANSACTION_THREAD_LOCAL.remove();
}
return dataSource.getConnection();
}
private String buildCacheKeyBySql(String sqlStr, Object... params) {
FastDatabaseInfo fastDatabaseInfo = getDatabaseInfo();
return FastChar.getSecurity().MD5_Encrypt(fastDatabaseInfo.getName() + sqlStr + Arrays.toString(params));
}
private String buildCacheTag(String... tableNames) {
FastDatabaseInfo fastDatabaseInfo = getDatabaseInfo();
for (int i = 0; i < tableNames.length; i++) {
tableNames[i] = fastDatabaseInfo.getName() + "@" + tableNames[i];
}
return "@" + FastStringUtils.join(tableNames, "@") + "@";
}
private String buildCacheTagBySql(String sqlStr) {
return buildCacheTag(getDatabaseInfo().getAllTables(sqlStr).toArray(new String[]{}));
}
/**
* 执行查询(带参数)
* @param sqlStr
* @param params
* @return
*/
public List> select(String sqlStr, Object... params) throws Exception {
String cacheKey = null;
String cacheTag = null;
inTime = System.currentTimeMillis();
if (getDatabaseInfo().isCache() && isUseCache()) {
cacheKey = buildCacheKeyBySql(sqlStr, params);
cacheTag = buildCacheTagBySql(sqlStr);
IFastCache iFastCacheProvider = FastChar.getCache();
if (iFastCacheProvider.exists(cacheTag, cacheKey)) {
List> cache = iFastCacheProvider.get(cacheTag, cacheKey);
if (cache != null) {
cacheLog(sqlStr);
return cache;
}
}
}
Connection connection = null;
PreparedStatement preparedStatement = null;
ResultSet resultSet = null;
try {
connection = getConnection();
if (connection == null) {
return null;
}
preparedStatement = connection.prepareStatement(sqlStr);
for (int i = 0; i < params.length; i++) {
Object value = params[i];
if (value != null) {
preparedStatement.setObject(i + 1, value);
} else {
preparedStatement.setNull(i + 1, Types.NULL);
}
}
resultSet = preparedStatement.executeQuery();
List> listResult = new FastResultSet(resultSet).getListResult();
if (getDatabaseInfo().isCache() && isUseCache()) {
IFastCache iFastCacheProvider = FastChar.getCache();
iFastCacheProvider.set(cacheTag, cacheKey, listResult);
}
return listResult;
} finally {
close(connection, preparedStatement, resultSet);
log(sqlStr, params);
}
}
public FastPage> select(int page, int pageSize, String sqlStr, Object... params) throws Exception {
inTime = System.currentTimeMillis();
FastPage> fastPage = new FastPage<>();
String type = getDatabaseInfo().getType();
if (page > 0) {
String countSql = FastSql.getInstance(type).getCountSql(sqlStr, "ct");
FastEntity countResult = selectFirst(countSql, params);
int countRow = countResult.getInt("ct");
fastPage.setTotalRow(countRow);
fastPage.setPage(page);
fastPage.setPageSize(pageSize);
fastPage.setTotalPage(countRow % pageSize == 0 ? countRow / pageSize : countRow / pageSize + 1);
} else {
fastPage.setTotalPage(1);
fastPage.setPage(1);
}
String pageSql = FastSql.getInstance(type).buildPageSql(sqlStr, page, pageSize);
FastSqlInfo fastSqlInfo = FastSqlInfo.newInstance().setSql(sqlStr).setParams(params);
fastSqlInfo.fromProperty();
fastPage.setSqlInfo(fastSqlInfo);
fastPage.setList(select(pageSql, params));
if (page < 0) {
fastPage.setTotalRow(fastPage.getList().size());
fastPage.setPageSize(fastPage.getTotalRow());
}
return fastPage;
}
/**
* 执行查询(带参数)
*
* @param sqlStr
* @param params
* @return
*/
public FastEntity> selectFirst(String sqlStr, Object... params) throws Exception {
inTime = System.currentTimeMillis();
String cacheKey = null;
String cacheTag = null;
if (getDatabaseInfo().isCache() && isUseCache()) {
cacheKey = buildCacheKeyBySql(sqlStr, params);
cacheTag = buildCacheTagBySql(sqlStr);
IFastCache iFastCacheProvider = FastChar.getCache();
if (iFastCacheProvider.exists(cacheTag, cacheKey)) {
FastEntity cache = iFastCacheProvider.get(cacheTag, cacheKey);
if (cache != null) {
cacheLog(sqlStr);
return cache;
}
}
}
Connection connection = null;
PreparedStatement preparedStatement = null;
ResultSet resultSet = null;
try {
connection = getConnection();
if (connection == null) {
return null;
}
preparedStatement = connection.prepareStatement(sqlStr);
for (int i = 0; i < params.length; i++) {
Object value = params[i];
if (value != null) {
preparedStatement.setObject(i + 1, value);
} else {
preparedStatement.setNull(i + 1, Types.NULL);
}
}
resultSet = preparedStatement.executeQuery();
FastEntity firstResult = new FastResultSet(resultSet).getFirstResult();
if (getDatabaseInfo().isCache() && isUseCache()) {
IFastCache iFastCacheProvider = FastChar.getCache();
iFastCacheProvider.set(cacheTag, cacheKey, firstResult);
}
return firstResult;
} finally {
close(connection, preparedStatement, resultSet);
log(sqlStr, params);
}
}
/**
* 执行查询(带参数)
*
* @param sqlStr
* @param params
* @return
*/
public FastEntity> selectLast(String sqlStr, Object... params) throws Exception {
inTime = System.currentTimeMillis();
String cacheKey = null;
String cacheTag = null;
if (getDatabaseInfo().isCache() && isUseCache()) {
cacheKey = buildCacheKeyBySql(sqlStr, params);
cacheTag = buildCacheTagBySql(sqlStr);
IFastCache iFastCacheProvider = FastChar.getCache();
if (iFastCacheProvider.exists(cacheTag, cacheKey)) {
FastEntity> cache = iFastCacheProvider.get(cacheTag, cacheKey);
if (cache != null) {
cacheLog(sqlStr);
return cache;
}
}
}
Connection connection = null;
PreparedStatement preparedStatement = null;
ResultSet resultSet = null;
try {
connection = getConnection();
if (connection == null) {
return null;
}
preparedStatement = connection.prepareStatement(sqlStr);
for (int i = 0; i < params.length; i++) {
Object value = params[i];
if (value != null) {
preparedStatement.setObject(i + 1, value);
} else {
preparedStatement.setNull(i + 1, Types.NULL);
}
}
resultSet = preparedStatement.executeQuery();
FastEntity lastResult = new FastResultSet(resultSet).getLastResult();
if (getDatabaseInfo().isCache() && isUseCache()) {
IFastCache iFastCacheProvider = FastChar.getCache();
iFastCacheProvider.set(cacheTag, cacheKey, lastResult);
}
return lastResult;
} finally {
close(connection, preparedStatement, resultSet);
log(sqlStr, params);
}
}
/**
* 执行更新sql
*
* @param sqlStr
* @param params
* @return 更新成功的数量
*/
public int update(String sqlStr, Object... params) throws Exception {
inTime = System.currentTimeMillis();
if (getDatabaseInfo().isCache() && isUseCache()) {
List allTables = getDatabaseInfo().getAllTables(sqlStr);
IFastCache iFastCacheProvider = FastChar.getCache();
for (String table : allTables) {
Set tags = iFastCacheProvider.getTags("*" + buildCacheTag(table) + "*");
for (String tag : tags) {
iFastCacheProvider.delete(tag);
}
}
}
int count;
Connection connection = null;
PreparedStatement preparedStatement = null;
try {
connection = getConnection();
if (connection == null) {
return -1;
}
preparedStatement = connection.prepareStatement(sqlStr);
for (int i = 0; i < params.length; i++) {
Object value = params[i];
if (value != null) {
preparedStatement.setObject(i + 1, value);
} else {
preparedStatement.setNull(i + 1, Types.NULL);
}
}
count = preparedStatement.executeUpdate();
} finally {
close(connection, preparedStatement);
log(sqlStr, params);
}
return count;
}
/**
* 执行插入数据
*
* @param sqlStr
* @param params
* @return 主键
*/
public int insert(String sqlStr, Object... params) throws Exception {
inTime = System.currentTimeMillis();
if (getDatabaseInfo().isCache() && isUseCache()) {
List allTables = getDatabaseInfo().getAllTables(sqlStr);
IFastCache iFastCacheProvider = FastChar.getCache();
for (String table : allTables) {
Set tags = iFastCacheProvider.getTags("*" + buildCacheTag(table) + "*");
for (String tag : tags) {
iFastCacheProvider.delete(tag);
}
}
}
int primary = 0;
Connection connection = null;
PreparedStatement preparedStatement = null;
ResultSet resultSet = null;
try {
connection = getConnection();
if (connection == null) {
return primary;
}
preparedStatement = connection.prepareStatement(sqlStr, Statement.RETURN_GENERATED_KEYS);
for (int i = 0; i < params.length; i++) {
Object value = params[i];
if (value != null) {
preparedStatement.setObject(i + 1, value);
} else {
preparedStatement.setNull(i + 1, Types.NULL);
}
}
primary = preparedStatement.executeUpdate();
resultSet = preparedStatement.getGeneratedKeys();
while (resultSet.next()) {
primary = resultSet.getInt(1);
}
} finally {
close(connection, preparedStatement, resultSet);
log(sqlStr, params);
}
return primary;
}
public boolean run(String sql) throws Exception {
inTime = System.currentTimeMillis();
Connection connection = null;
Statement statement = null;
try {
connection = getConnection();
if (connection == null) {
return false;
}
statement = connection.createStatement();
return statement.execute(sql);
} finally {
close(connection, statement);
log(sql, null);
}
}
/**
* 批量执行sql
*
* @param sqlArray sql数组
* @return 执行结果
*/
public int[] batch(String[] sqlArray, int batchSize) throws Exception {
return batch(Arrays.asList(sqlArray), batchSize);
}
/**
* 批量执行sql
*
* @param sqlList sql数组
* @return 执行结果
*/
public int[] batch(List sqlList, int batchSize) throws Exception {
inTime = System.currentTimeMillis();
if (getDatabaseInfo().isCache() && isUseCache()) {
for (String sqlStr : sqlList) {
List allTables = getDatabaseInfo().getAllTables(sqlStr);
IFastCache iFastCacheProvider = FastChar.getCache();
for (String table : allTables) {
Set tags = iFastCacheProvider.getTags("*" + buildCacheTag(table) + "*");
for (String tag : tags) {
iFastCacheProvider.delete(tag);
}
}
}
}
Connection connection = null;
Statement statement = null;
try {
List result = new ArrayList<>();
int count = 0;
connection = getConnection();
if (connection == null) {
return new int[0];
}
statement = connection.createStatement();
for (String sql : sqlList) {
statement.addBatch(sql);
if (++count % batchSize == 0) {
int[] executeBatch = statement.executeBatch();
Integer[] integers = FastArrayUtils.toObject(executeBatch);
if (integers != null) {
result.addAll(Arrays.asList(integers));
}
statement.clearBatch();
}
}
int[] executeBatch = statement.executeBatch();
Integer[] integers = FastArrayUtils.toObject(executeBatch);
if (integers != null) {
result.addAll(Arrays.asList(integers));
}
return FastArrayUtils.toPrimitive(result.toArray(new Integer[]{}));
} finally {
close(connection, statement);
for (String sql : sqlList) {
log(sql, null);
}
}
}
/**
* 批量执行sql
*
* @param sqlStr 共同的sql语句
* @param params 参数数据的数组
*/
public int[] batch(String sqlStr, List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy