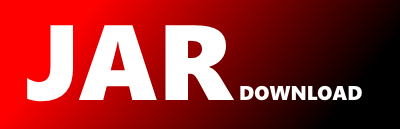
com.fasterxml.clustermate.api.RequestPathBuilder Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of clustermate-api Show documentation
Show all versions of clustermate-api Show documentation
Data types needed for service abstraction,
used by both server and client components.
The newest version!
package com.fasterxml.clustermate.api;
import java.util.*;
import com.fasterxml.storemate.shared.compress.Compression;
/**
* Builder object used for constructing {@link RequestPath}
* instances.
*/
public abstract class RequestPathBuilder<
THIS extends RequestPathBuilder
>
{
/**
* Method that will construct the immutable {@link RequestPath} instance
* with information builder has accumulated.
*/
public abstract RequestPath build();
/*
/*********************************************************************
/* Mutators
/*********************************************************************
*/
/**
* Method that will append a single path segment, escaping characters
* as necessary, including escaping of slash character so that
* the whole value remains parseable path segment.
*
* @return Builder instance to use for further calls (may be 'this',
* but does not have to be)
*/
public abstract THIS addPathSegment(String segment);
/**
* Method that will append one or more path segments; contents are
* only escaped only for mandatory characters, but slashes are NOT
* escaped, so input may contain multiple segments
*
* @return Builder instance to use for further calls (may be 'this',
* but does not have to be)
*/
public abstract THIS addPathSegmentsRaw(String segments);
/**
* Convenience method for appending a sequence of path segments,
* as if calling {@link #addPathSegment(String)} once per segment,
* in the order indicated.
*/
public THIS addPathSegments(String[] segments)
{
RequestPathBuilder builder = this;
for (String segment : segments) {
builder = builder.addPathSegment(segment);
}
return _this(builder);
}
/**
* Method that will add a single query parameter in the logical
* path, with given value.
*
* @return Builder instance to use for further calls (may be 'this',
* but does not have to be)
*/
public abstract THIS addParameter(String key, String value);
/**
* Convenience method, functionally equivalent to:
*
* addParameter(String.valueOf(value));
*
*/
public THIS addParameter(String key, int value) {
return addParameter(key, String.valueOf(value));
}
/**
* Convenience method, functionally equivalent to:
*
* addParameter(String.valueOf(value));
*
*/
public THIS addParameter(String key, long value) {
return addParameter(key, String.valueOf(value));
}
public abstract THIS addHeader(String key, String value);
public THIS addHeader(String key, long value) {
return addHeader(key, String.valueOf(value));
}
public abstract THIS setHeader(String key, String value);
public THIS setHeader(String key, long value) {
return setHeader(key, String.valueOf(value));
}
public abstract THIS setContentType(String contentType);
public THIS addCompression(Compression comp, long originalLength) {
if (comp != null) {
THIS req = addHeader(ClusterMateConstants.HTTP_HEADER_COMPRESSION,
comp.asContentEncoding());
if (comp != Compression.NONE) {
req = req.addHeader(ClusterMateConstants.CUSTOM_HTTP_HEADER_UNCOMPRESSED_LENGTH,
originalLength);
}
return req;
}
return _this();
}
/*
/*********************************************************************
/* Accessors
/*********************************************************************
*/
/**
* Method for returning only the logical "server part", which also includes
* the protocol (like 'http') and port number, as well as trailing
* slash.
*/
public abstract String getServerPart();
/**
* Method for returning only the logical "path" part, without including
* either server part or query parameters.
*/
public abstract String getPath();
public abstract boolean hasHeaders();
/**
* Implementations MUST override this to produce a valid URL that
* represents the current state of builder.
*/
@Override
public abstract String toString();
/*
/*********************************************************************
/* Helper methods for sub-classes
/*********************************************************************
*/
@SuppressWarnings("unchecked")
protected THIS _this() {
return (THIS) this;
}
@SuppressWarnings("unchecked")
protected THIS _this(RequestPathBuilder b) {
return (THIS) b;
}
protected static List _arrayToList(String[] qp)
{
if (qp == null) {
return null;
}
int len = qp.length;
List list = new ArrayList(Math.min(8, len));
if (len > 0) {
for (int i = 0; i < len; ++i) {
list.add(qp[i]);
}
}
return list;
}
protected static Map _arrayToMap(Object[] qp)
{
if (qp == null) {
return null;
}
int len = qp.length;
Map result = new LinkedHashMap(len >> 1);
for (int i = 0; i < len; i += 2) {
result.put((String) qp[i], qp[i+1]);
}
return result;
}
protected Map _newHeaderMap() {
return new LinkedHashMap(8);
}
@SuppressWarnings("unchecked")
protected Map _defaultAddHeader(Map headers,
String key, String value)
{
if (headers == null) {
headers = _newHeaderMap();
headers.put(key, value);
return headers;
}
Object ob = headers.get(key);
if (ob == null) {
headers.put(key, value);
} else {
List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy