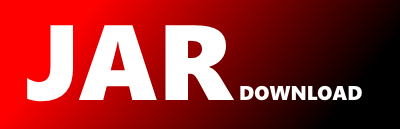
com.fasterxml.jackson.dataformat.avro.AvroWriteContext Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jackson-dataformat-avro Show documentation
Show all versions of jackson-dataformat-avro Show documentation
Support for reading and writing AVRO-encoded data via Jackson
abstractions.
package com.fasterxml.jackson.dataformat.avro;
import java.io.IOException;
import org.apache.avro.Schema;
import org.apache.avro.generic.*;
import org.apache.avro.io.BinaryEncoder;
import com.fasterxml.jackson.core.JsonStreamContext;
import com.fasterxml.jackson.dataformat.avro.ser.ArrayWriteContext;
import com.fasterxml.jackson.dataformat.avro.ser.ObjectWriteContext;
public abstract class AvroWriteContext
extends JsonStreamContext
{
protected final AvroWriteContext _parent;
protected final AvroGenerator _generator;
protected final Schema _schema;
/*
/**********************************************************
/* Life-cycle
/**********************************************************
*/
protected AvroWriteContext(int type, AvroWriteContext parent,
AvroGenerator generator, Schema schema)
{
super();
_type = type;
_parent = parent;
_generator = generator;
_schema = schema;
}
// // // Factory methods
public static AvroWriteContext createRootContext(AvroGenerator generator, Schema schema) {
return new RootContext(generator, schema);
}
/**
* Factory method called to get a placeholder context that is only
* in place until actual schema is handed.
*/
public static AvroWriteContext createNullContext() {
return NullContext.instance;
}
public abstract AvroWriteContext createChildArrayContext();
public abstract AvroWriteContext createChildObjectContext();
@Override
public final AvroWriteContext getParent() { return _parent; }
@Override
public String getCurrentName() { return null; }
/**
* Method that writer is to call before it writes a field name.
*
* @return True for Object (record) context; false for others
*/
public boolean writeFieldName(String name) { return false; }
public abstract void writeValue(Object value);
public void complete(BinaryEncoder encoder) throws IOException {
throw new IllegalStateException("Can not be called on "+getClass().getName());
}
public boolean canClose() { return true; }
protected abstract void appendDesc(StringBuilder sb);
// // // Overridden standard methods
/**
* Overridden to provide developer writeable "JsonPath" representation
* of the context.
*/
@Override
public final String toString()
{
StringBuilder sb = new StringBuilder(64);
appendDesc(sb);
return sb.toString();
}
// // // Shared helper methods
protected GenericRecord _createRecord(Schema schema)
{
// Quick check: if type is Union, need to find actual record type...
if (schema.getType() == Schema.Type.UNION) {
Schema match = null;
for (Schema s : schema.getTypes()) {
if (s.getType() == Schema.Type.RECORD) {
if (match != null) {
throw new IllegalStateException("Multiple Record types, can not figure out which to use for: "
+schema);
}
match = s;
}
}
if (match == null) {
throw new IllegalStateException("No Record type found in union type: "+schema);
}
schema = match;
}
return new GenericData.Record(schema);
}
protected GenericArray
© 2015 - 2025 Weber Informatics LLC | Privacy Policy