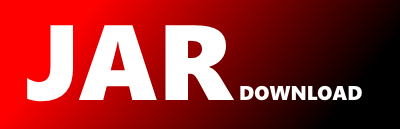
com.fasterxml.jackson.jr.private_.io.doubleparser.FastDoubleParser Maven / Gradle / Ivy
Show all versions of jackson-jr-all Show documentation
/**
* References:
*
* - This class has been derived from "FastDoubleParser".
* - Copyright (c) Werner Randelshofer. Apache 2.0 License.
* github.com.
*
*/
package com.fasterxml.jackson.core.io.doubleparser;
/**
* Provides static method for parsing a {@code double} from a
* {@link CharSequence}, {@code char} array or {@code byte} array.
*/
public class FastDoubleParser {
/**
* Don't let anyone instantiate this class.
*/
private FastDoubleParser() {
}
/**
* Convenience method for calling {@link #parseDouble(CharSequence, int, int)}.
*
* @param str the string to be parsed
* @return the parsed double value
* @throws NumberFormatException if the string can not be parsed
*/
public static double parseDouble(CharSequence str) throws NumberFormatException {
return parseDouble(str, 0, str.length());
}
/**
* Parses a {@code FloatingPointLiteral} from a {@link CharSequence} and converts it
* into a {@code double} value.
*
* See {@link com.fasterxml.jackson.core.io.doubleparser} for the syntax of {@code FloatingPointLiteral}.
*
* @param str the string to be parsed
* @param offset the start offset of the {@code FloatingPointLiteral} in {@code str}
* @param length the length of {@code FloatingPointLiteral} in {@code str}
* @return the parsed double value
* @throws NumberFormatException if the string can not be parsed
*/
public static double parseDouble(CharSequence str, int offset, int length) throws NumberFormatException {
long bitPattern = new DoubleBitsFromCharSequence().parseFloatingPointLiteral(str, offset, length);
if (bitPattern == AbstractFloatValueParser.PARSE_ERROR) {
throw new NumberFormatException("Illegal input");
}
return Double.longBitsToDouble(bitPattern);
}
/**
* Convenience method for calling {@link #parseDouble(char[], int, int)}.
*
* @param str the string to be parsed
* @return the parsed double value
* @throws NumberFormatException if the string can not be parsed
*/
public static double parseDouble(char[] str) throws NumberFormatException {
return parseDouble(str, 0, str.length);
}
/**
* Parses a {@code FloatingPointLiteral} from a {@code byte}-Array and converts it
* into a {@code double} value.
*
* See {@link com.fasterxml.jackson.core.io.doubleparser} for the syntax of {@code FloatingPointLiteral}.
*
* @param str the string to be parsed, a byte array with characters
* in ISO-8859-1, ASCII or UTF-8 encoding
* @param offset The index of the first character to parse
* @param length The number of characters to parse
* @return the parsed double value
* @throws NumberFormatException if the string can not be parsed
*/
public static double parseDouble(char[] str, int offset, int length) throws NumberFormatException {
long bitPattern = new DoubleBitsFromCharArray().parseFloatingPointLiteral(str, offset, length);
if (bitPattern == AbstractFloatValueParser.PARSE_ERROR) {
throw new NumberFormatException("Illegal input");
}
return Double.longBitsToDouble(bitPattern);
}
/**
* Parses a {@code FloatingPointLiteral} from a {@link CharSequence} and converts it
* into a bit pattern that encodes a {@code double} value.
*
* See {@link com.fasterxml.jackson.core.io.doubleparser} for the syntax of {@code FloatingPointLiteral}.
*
* Usage example:
*
* long bitPattern = parseDoubleBits("3.14", 0, 4);
* if (bitPattern == -1L) {
* ...handle parse error...
* } else {
* double d = Double.longBitsToDouble(bitPattern);
* }
*
*
* @param str the string to be parsed
* @param offset the start offset of the {@code FloatingPointLiteral} in {@code str}
* @param length the length of {@code FloatingPointLiteral} in {@code str}
* @return the bit pattern of the parsed value, if the input is legal;
* otherwise, {@code -1L}.
*/
public static long parseDoubleBits(CharSequence str, int offset, int length) {
return new DoubleBitsFromCharSequence().parseFloatingPointLiteral(str, offset, length);
}
/**
* Parses a {@code FloatingPointLiteral} from a {@code byte}-Array and converts it
* into a bit pattern that encodes a {@code double} value.
*
* See {@link com.fasterxml.jackson.core.io.doubleparser} for the syntax of {@code FloatingPointLiteral}.
*
* See {@link #parseDoubleBits(CharSequence, int, int)} for a usage example.
*
* @param str the string to be parsed, a byte array with characters
* in ISO-8859-1, ASCII or UTF-8 encoding
* @param offset The index of the first character to parse
* @param length The number of characters to parse
* @return the bit pattern of the parsed value, if the input is legal;
* otherwise, {@code -1L}.
*/
public static long parseDoubleBits(char[] str, int offset, int length) {
return new DoubleBitsFromCharArray().parseFloatingPointLiteral(str, offset, length);
}
}