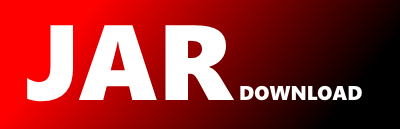
com.fasterxml.jackson.jr.ob.api.CollectionBuilder Maven / Gradle / Ivy
Show all versions of jackson-jr-all Show documentation
package com.fasterxml.jackson.jr.ob.api;
import java.lang.reflect.Array;
import java.util.*;
import com.fasterxml.jackson.jr.ob.JSON.Feature;
/**
* Helper class that is used for constructing {@link java.util.Collection}s
* to map JSON Array values in.
*
* Objects server both as "factories" for creating new builders (blueprint
* style), and as actual builders. For each distinct read operation,
* {@link #newBuilder} will be called at least once; this instance
* may be used and reused multiple times, as calling {@link #start}
* will reset the state so that more {@link Collection}s may be built.
*/
public abstract class CollectionBuilder
{
protected final static Object[] EMPTY_ARRAY = new Object[0];
protected final int _features;
/**
* Optional {@link Collection} implementation class, used when specific
* implementation is desired.
*/
protected final Class> _collectionType;
/*
/**********************************************************************
/* Construction
/**********************************************************************
*/
protected CollectionBuilder(int features, Class> collImpl) {
_features = features;
_collectionType = collImpl;
}
/**
* Factory method for getting a blueprint instance of the default
* {@link CollectionBuilder} implementation.
*/
public static CollectionBuilder defaultImpl() {
return new Default(0, null);
}
public abstract CollectionBuilder newBuilder(int features);
public abstract CollectionBuilder newBuilder(Class> collImpl);
public CollectionBuilder newBuilder() {
return newBuilder(_features);
}
/*
/**********************************************************************
/* Simple accessors
/**********************************************************************
*/
public final boolean isEnabled(Feature f) {
return f.isEnabled(_features);
}
/*
/**********************************************************************
/* Actual building
/**********************************************************************
*/
public abstract CollectionBuilder start();
public abstract CollectionBuilder add(Object value);
/**
* The usual build method to use for constructing {@link Collection}
*/
public abstract Collection